
Only encoders configured.
Dependencies: mbed-rtos mbed ros_lib_indigo
stm32f7xx_hal_msp.c
00001 /** 00002 ****************************************************************************** 00003 * File Name : stm32f7xx_hal_msp.c 00004 * Description : This file provides code for the MSP Initialization 00005 * and de-Initialization codes. 00006 ****************************************************************************** 00007 * 00008 * COPYRIGHT(c) 2017 STMicroelectronics 00009 * 00010 * Redistribution and use in source and binary forms, with or without modification, 00011 * are permitted provided that the following conditions are met: 00012 * 1. Redistributions of source code must retain the above copyright notice, 00013 * this list of conditions and the following disclaimer. 00014 * 2. Redistributions in binary form must reproduce the above copyright notice, 00015 * this list of conditions and the following disclaimer in the documentation 00016 * and/or other materials provided with the distribution. 00017 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00018 * may be used to endorse or promote products derived from this software 00019 * without specific prior written permission. 00020 * 00021 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00022 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00023 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00024 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00025 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00026 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00027 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00028 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00029 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00030 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00031 * 00032 ****************************************************************************** 00033 */ 00034 /* Includes ------------------------------------------------------------------*/ 00035 #include "stm32f7xx_hal.h" 00036 00037 extern void Error_Handler(void); 00038 /* USER CODE BEGIN 0 */ 00039 00040 /* USER CODE END 0 */ 00041 /** 00042 * Initializes the Global MSP. 00043 */ 00044 void HAL_MspInit(void) 00045 { 00046 /* USER CODE BEGIN MspInit 0 */ 00047 00048 /* USER CODE END MspInit 0 */ 00049 00050 HAL_NVIC_SetPriorityGrouping(NVIC_PRIORITYGROUP_4); 00051 00052 /* System interrupt init*/ 00053 /* MemoryManagement_IRQn interrupt configuration */ 00054 HAL_NVIC_SetPriority(MemoryManagement_IRQn, 0, 0); 00055 /* BusFault_IRQn interrupt configuration */ 00056 HAL_NVIC_SetPriority(BusFault_IRQn, 0, 0); 00057 /* UsageFault_IRQn interrupt configuration */ 00058 HAL_NVIC_SetPriority(UsageFault_IRQn, 0, 0); 00059 /* SVCall_IRQn interrupt configuration */ 00060 HAL_NVIC_SetPriority(SVCall_IRQn, 0, 0); 00061 /* DebugMonitor_IRQn interrupt configuration */ 00062 HAL_NVIC_SetPriority(DebugMonitor_IRQn, 0, 0); 00063 /* PendSV_IRQn interrupt configuration */ 00064 HAL_NVIC_SetPriority(PendSV_IRQn, 0, 0); 00065 /* SysTick_IRQn interrupt configuration */ 00066 HAL_NVIC_SetPriority(SysTick_IRQn, 0, 0); 00067 00068 /* USER CODE BEGIN MspInit 1 */ 00069 00070 /* USER CODE END MspInit 1 */ 00071 } 00072 00073 void HAL_TIM_Encoder_MspInit(TIM_HandleTypeDef* htim_encoder) 00074 { 00075 00076 GPIO_InitTypeDef GPIO_InitStruct; 00077 if(htim_encoder->Instance==TIM1) 00078 { 00079 /* USER CODE BEGIN TIM1_MspInit 0 */ 00080 00081 /* USER CODE END TIM1_MspInit 0 */ 00082 /* Peripheral clock enable */ 00083 __HAL_RCC_TIM1_CLK_ENABLE(); 00084 00085 /**TIM1 GPIO Configuration 00086 PE9 ------> TIM1_CH1 00087 PE11 ------> TIM1_CH2 00088 */ 00089 GPIO_InitStruct.Pin = GPIO_PIN_9|GPIO_PIN_11; 00090 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00091 GPIO_InitStruct.Pull = GPIO_PULLUP; 00092 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00093 GPIO_InitStruct.Alternate = GPIO_AF1_TIM1; 00094 HAL_GPIO_Init(GPIOE, &GPIO_InitStruct); 00095 00096 /* USER CODE BEGIN TIM1_MspInit 1 */ 00097 00098 /* USER CODE END TIM1_MspInit 1 */ 00099 } 00100 else if(htim_encoder->Instance==TIM2) 00101 { 00102 /* USER CODE BEGIN TIM2_MspInit 0 */ 00103 00104 /* USER CODE END TIM2_MspInit 0 */ 00105 /* Peripheral clock enable */ 00106 __HAL_RCC_TIM2_CLK_ENABLE(); 00107 00108 /**TIM2 GPIO Configuration 00109 PA0/WKUP ------> TIM2_CH1 00110 PA1 ------> TIM2_CH2 00111 */ 00112 GPIO_InitStruct.Pin = GPIO_PIN_0|GPIO_PIN_1; 00113 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00114 GPIO_InitStruct.Pull = GPIO_PULLUP; 00115 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00116 GPIO_InitStruct.Alternate = GPIO_AF1_TIM2; 00117 HAL_GPIO_Init(GPIOA, &GPIO_InitStruct); 00118 00119 /* USER CODE BEGIN TIM2_MspInit 1 */ 00120 00121 /* USER CODE END TIM2_MspInit 1 */ 00122 } 00123 else if(htim_encoder->Instance==TIM3) 00124 { 00125 /* USER CODE BEGIN TIM3_MspInit 0 */ 00126 00127 /* USER CODE END TIM3_MspInit 0 */ 00128 /* Peripheral clock enable */ 00129 __HAL_RCC_TIM3_CLK_ENABLE(); 00130 00131 /**TIM3 GPIO Configuration 00132 PA6 ------> TIM3_CH1 00133 PA7 ------> TIM3_CH2 00134 */ 00135 GPIO_InitStruct.Pin = GPIO_PIN_6|GPIO_PIN_7; 00136 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00137 GPIO_InitStruct.Pull = GPIO_PULLUP; 00138 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00139 GPIO_InitStruct.Alternate = GPIO_AF2_TIM3; 00140 HAL_GPIO_Init(GPIOA, &GPIO_InitStruct); 00141 00142 /* USER CODE BEGIN TIM3_MspInit 1 */ 00143 00144 /* USER CODE END TIM3_MspInit 1 */ 00145 } 00146 else if(htim_encoder->Instance==TIM4) 00147 { 00148 /* USER CODE BEGIN TIM4_MspInit 0 */ 00149 00150 /* USER CODE END TIM4_MspInit 0 */ 00151 /* Peripheral clock enable */ 00152 __HAL_RCC_TIM4_CLK_ENABLE(); 00153 00154 /**TIM4 GPIO Configuration 00155 PD12 ------> TIM4_CH1 00156 PD13 ------> TIM4_CH2 00157 */ 00158 GPIO_InitStruct.Pin = GPIO_PIN_12|GPIO_PIN_13; 00159 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00160 GPIO_InitStruct.Pull = GPIO_PULLUP; 00161 GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW; 00162 GPIO_InitStruct.Alternate = GPIO_AF2_TIM4; 00163 HAL_GPIO_Init(GPIOD, &GPIO_InitStruct); 00164 00165 /* USER CODE BEGIN TIM4_MspInit 1 */ 00166 00167 /* USER CODE END TIM4_MspInit 1 */ 00168 } 00169 00170 } 00171 00172 void HAL_TIM_Encoder_MspDeInit(TIM_HandleTypeDef* htim_encoder) 00173 { 00174 00175 if(htim_encoder->Instance==TIM1) 00176 { 00177 /* USER CODE BEGIN TIM1_MspDeInit 0 */ 00178 00179 /* USER CODE END TIM1_MspDeInit 0 */ 00180 /* Peripheral clock disable */ 00181 __HAL_RCC_TIM1_CLK_DISABLE(); 00182 00183 /**TIM1 GPIO Configuration 00184 PE9 ------> TIM1_CH1 00185 PE11 ------> TIM1_CH2 00186 */ 00187 HAL_GPIO_DeInit(GPIOE, GPIO_PIN_9|GPIO_PIN_11); 00188 00189 /* USER CODE BEGIN TIM1_MspDeInit 1 */ 00190 00191 /* USER CODE END TIM1_MspDeInit 1 */ 00192 } 00193 else if(htim_encoder->Instance==TIM2) 00194 { 00195 /* USER CODE BEGIN TIM2_MspDeInit 0 */ 00196 00197 /* USER CODE END TIM2_MspDeInit 0 */ 00198 /* Peripheral clock disable */ 00199 __HAL_RCC_TIM2_CLK_DISABLE(); 00200 00201 /**TIM2 GPIO Configuration 00202 PA0/WKUP ------> TIM2_CH1 00203 PA1 ------> TIM2_CH2 00204 */ 00205 HAL_GPIO_DeInit(GPIOA, GPIO_PIN_0|GPIO_PIN_1); 00206 00207 /* USER CODE BEGIN TIM2_MspDeInit 1 */ 00208 00209 /* USER CODE END TIM2_MspDeInit 1 */ 00210 } 00211 else if(htim_encoder->Instance==TIM3) 00212 { 00213 /* USER CODE BEGIN TIM3_MspDeInit 0 */ 00214 00215 /* USER CODE END TIM3_MspDeInit 0 */ 00216 /* Peripheral clock disable */ 00217 __HAL_RCC_TIM3_CLK_DISABLE(); 00218 00219 /**TIM3 GPIO Configuration 00220 PA6 ------> TIM3_CH1 00221 PA7 ------> TIM3_CH2 00222 */ 00223 HAL_GPIO_DeInit(GPIOA, GPIO_PIN_6|GPIO_PIN_7); 00224 00225 /* USER CODE BEGIN TIM3_MspDeInit 1 */ 00226 00227 /* USER CODE END TIM3_MspDeInit 1 */ 00228 } 00229 else if(htim_encoder->Instance==TIM4) 00230 { 00231 /* USER CODE BEGIN TIM4_MspDeInit 0 */ 00232 00233 /* USER CODE END TIM4_MspDeInit 0 */ 00234 /* Peripheral clock disable */ 00235 __HAL_RCC_TIM4_CLK_DISABLE(); 00236 00237 /**TIM4 GPIO Configuration 00238 PD12 ------> TIM4_CH1 00239 PD13 ------> TIM4_CH2 00240 */ 00241 HAL_GPIO_DeInit(GPIOD, GPIO_PIN_12|GPIO_PIN_13); 00242 00243 /* USER CODE BEGIN TIM4_MspDeInit 1 */ 00244 00245 /* USER CODE END TIM4_MspDeInit 1 */ 00246 } 00247 00248 } 00249 00250 /* USER CODE BEGIN 1 */ 00251 00252 /* USER CODE END 1 */ 00253 00254 /** 00255 * @} 00256 */ 00257 00258 /** 00259 * @} 00260 */ 00261 00262 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00263
Generated on Wed Jul 13 2022 18:23:41 by
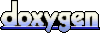