
Only encoders configured.
Dependencies: mbed-rtos mbed ros_lib_indigo
stm32f7xx_hal.h
00001 /** 00002 ****************************************************************************** 00003 * @file stm32f7xx_hal.h 00004 * @author MCD Application Team 00005 * @version V1.1.2 00006 * @date 23-September-2016 00007 * @brief This file contains all the functions prototypes for the HAL 00008 * module driver. 00009 ****************************************************************************** 00010 * @attention 00011 * 00012 * <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2> 00013 * 00014 * Redistribution and use in source and binary forms, with or without modification, 00015 * are permitted provided that the following conditions are met: 00016 * 1. Redistributions of source code must retain the above copyright notice, 00017 * this list of conditions and the following disclaimer. 00018 * 2. Redistributions in binary form must reproduce the above copyright notice, 00019 * this list of conditions and the following disclaimer in the documentation 00020 * and/or other materials provided with the distribution. 00021 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00022 * may be used to endorse or promote products derived from this software 00023 * without specific prior written permission. 00024 * 00025 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00026 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00027 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00028 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00029 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00030 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00031 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00032 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00033 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00034 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00035 * 00036 ****************************************************************************** 00037 */ 00038 00039 /* Define to prevent recursive inclusion -------------------------------------*/ 00040 #ifndef __STM32F7xx_HAL_H 00041 #define __STM32F7xx_HAL_H 00042 00043 #ifdef __cplusplus 00044 extern "C" { 00045 #endif 00046 00047 /* Includes ------------------------------------------------------------------*/ 00048 #include "stm32f7xx_hal_conf.h" 00049 00050 /** @addtogroup STM32F7xx_HAL_Driver 00051 * @{ 00052 */ 00053 00054 /** @addtogroup HAL 00055 * @{ 00056 */ 00057 00058 /* Exported types ------------------------------------------------------------*/ 00059 /* Exported constants --------------------------------------------------------*/ 00060 /** @defgroup SYSCFG_Exported_Constants SYSCFG Exported Constants 00061 * @{ 00062 */ 00063 00064 /** @defgroup SYSCFG_BootMode Boot Mode 00065 * @{ 00066 */ 00067 #define SYSCFG_MEM_BOOT_ADD0 ((uint32_t)0x00000000U) 00068 #define SYSCFG_MEM_BOOT_ADD1 SYSCFG_MEMRMP_MEM_BOOT 00069 /** 00070 * @} 00071 */ 00072 00073 /** 00074 * @} 00075 */ 00076 00077 /* Exported macro ------------------------------------------------------------*/ 00078 /** @defgroup HAL_Exported_Macros HAL Exported Macros 00079 * @{ 00080 */ 00081 00082 /** @brief Freeze/Unfreeze Peripherals in Debug mode 00083 */ 00084 #define __HAL_DBGMCU_FREEZE_TIM2() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_TIM2_STOP)) 00085 #define __HAL_DBGMCU_FREEZE_TIM3() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_TIM3_STOP)) 00086 #define __HAL_DBGMCU_FREEZE_TIM4() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_TIM4_STOP)) 00087 #define __HAL_DBGMCU_FREEZE_TIM5() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_TIM5_STOP)) 00088 #define __HAL_DBGMCU_FREEZE_TIM6() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_TIM6_STOP)) 00089 #define __HAL_DBGMCU_FREEZE_TIM7() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_TIM7_STOP)) 00090 #define __HAL_DBGMCU_FREEZE_TIM12() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_TIM12_STOP)) 00091 #define __HAL_DBGMCU_FREEZE_TIM13() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_TIM13_STOP)) 00092 #define __HAL_DBGMCU_FREEZE_TIM14() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_TIM14_STOP)) 00093 #define __HAL_DBGMCU_FREEZE_LPTIM1() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_LPTIM1_STOP)) 00094 #define __HAL_DBGMCU_FREEZE_RTC() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_RTC_STOP)) 00095 #define __HAL_DBGMCU_FREEZE_WWDG() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_WWDG_STOP)) 00096 #define __HAL_DBGMCU_FREEZE_IWDG() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_IWDG_STOP)) 00097 #define __HAL_DBGMCU_FREEZE_I2C1_TIMEOUT() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_I2C1_SMBUS_TIMEOUT)) 00098 #define __HAL_DBGMCU_FREEZE_I2C2_TIMEOUT() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_I2C2_SMBUS_TIMEOUT)) 00099 #define __HAL_DBGMCU_FREEZE_I2C3_TIMEOUT() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_I2C3_SMBUS_TIMEOUT)) 00100 #define __HAL_DBGMCU_FREEZE_I2C4_TIMEOUT() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_I2C4_SMBUS_TIMEOUT)) 00101 #define __HAL_DBGMCU_FREEZE_CAN1() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_CAN1_STOP)) 00102 #define __HAL_DBGMCU_FREEZE_CAN2() (DBGMCU->APB1FZ |= (DBGMCU_APB1_FZ_DBG_CAN2_STOP)) 00103 #define __HAL_DBGMCU_FREEZE_TIM1() (DBGMCU->APB2FZ |= (DBGMCU_APB2_FZ_DBG_TIM1_STOP)) 00104 #define __HAL_DBGMCU_FREEZE_TIM8() (DBGMCU->APB2FZ |= (DBGMCU_APB2_FZ_DBG_TIM8_STOP)) 00105 #define __HAL_DBGMCU_FREEZE_TIM9() (DBGMCU->APB2FZ |= (DBGMCU_APB2_FZ_DBG_TIM9_STOP)) 00106 #define __HAL_DBGMCU_FREEZE_TIM10() (DBGMCU->APB2FZ |= (DBGMCU_APB2_FZ_DBG_TIM10_STOP)) 00107 #define __HAL_DBGMCU_FREEZE_TIM11() (DBGMCU->APB2FZ |= (DBGMCU_APB2_FZ_DBG_TIM11_STOP)) 00108 00109 #define __HAL_DBGMCU_UNFREEZE_TIM2() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_TIM2_STOP)) 00110 #define __HAL_DBGMCU_UNFREEZE_TIM3() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_TIM3_STOP)) 00111 #define __HAL_DBGMCU_UNFREEZE_TIM4() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_TIM4_STOP)) 00112 #define __HAL_DBGMCU_UNFREEZE_TIM5() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_TIM5_STOP)) 00113 #define __HAL_DBGMCU_UNFREEZE_TIM6() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_TIM6_STOP)) 00114 #define __HAL_DBGMCU_UNFREEZE_TIM7() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_TIM7_STOP)) 00115 #define __HAL_DBGMCU_UNFREEZE_TIM12() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_TIM12_STOP)) 00116 #define __HAL_DBGMCU_UNFREEZE_TIM13() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_TIM13_STOP)) 00117 #define __HAL_DBGMCU_UNFREEZE_TIM14() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_TIM14_STOP)) 00118 #define __HAL_DBGMCU_UNFREEZE_LPTIM1() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_LPTIM1_STOP)) 00119 #define __HAL_DBGMCU_UNFREEZE_RTC() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_RTC_STOP)) 00120 #define __HAL_DBGMCU_UNFREEZE_WWDG() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_WWDG_STOP)) 00121 #define __HAL_DBGMCU_UNFREEZE_IWDG() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_IWDG_STOP)) 00122 #define __HAL_DBGMCU_UNFREEZE_I2C1_TIMEOUT() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_I2C1_SMBUS_TIMEOUT)) 00123 #define __HAL_DBGMCU_UNFREEZE_I2C2_TIMEOUT() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_I2C2_SMBUS_TIMEOUT)) 00124 #define __HAL_DBGMCU_UNFREEZE_I2C3_TIMEOUT() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_I2C3_SMBUS_TIMEOUT)) 00125 #define __HAL_DBGMCU_UNFREEZE_I2C4_TIMEOUT() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_I2C4_SMBUS_TIMEOUT)) 00126 #define __HAL_DBGMCU_UNFREEZE_CAN1() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_CAN1_STOP)) 00127 #define __HAL_DBGMCU_UNFREEZE_CAN2() (DBGMCU->APB1FZ &= ~(DBGMCU_APB1_FZ_DBG_CAN2_STOP)) 00128 #define __HAL_DBGMCU_UNFREEZE_TIM1() (DBGMCU->APB2FZ &= ~(DBGMCU_APB2_FZ_DBG_TIM1_STOP)) 00129 #define __HAL_DBGMCU_UNFREEZE_TIM8() (DBGMCU->APB2FZ &= ~(DBGMCU_APB2_FZ_DBG_TIM8_STOP)) 00130 #define __HAL_DBGMCU_UNFREEZE_TIM9() (DBGMCU->APB2FZ &= ~(DBGMCU_APB2_FZ_DBG_TIM9_STOP)) 00131 #define __HAL_DBGMCU_UNFREEZE_TIM10() (DBGMCU->APB2FZ &= ~(DBGMCU_APB2_FZ_DBG_TIM10_STOP)) 00132 #define __HAL_DBGMCU_UNFREEZE_TIM11() (DBGMCU->APB2FZ &= ~(DBGMCU_APB2_FZ_DBG_TIM11_STOP)) 00133 00134 00135 /** @brief FMC (NOR/RAM) mapped at 0x60000000 and SDRAM mapped at 0xC0000000 00136 */ 00137 #define __HAL_SYSCFG_REMAPMEMORY_FMC() (SYSCFG->MEMRMP &= ~(SYSCFG_MEMRMP_SWP_FMC)) 00138 00139 00140 /** @brief FMC/SDRAM mapped at 0x60000000 (NOR/RAM) mapped at 0xC0000000 00141 */ 00142 #define __HAL_SYSCFG_REMAPMEMORY_FMC_SDRAM() do {SYSCFG->MEMRMP &= ~(SYSCFG_MEMRMP_SWP_FMC);\ 00143 SYSCFG->MEMRMP |= (SYSCFG_MEMRMP_SWP_FMC_0);\ 00144 }while(0); 00145 /** 00146 * @brief Return the memory boot mapping as configured by user. 00147 * @retval The boot mode as configured by user. The returned value can be one 00148 * of the following values: 00149 * @arg @ref SYSCFG_MEM_BOOT_ADD0 00150 * @arg @ref SYSCFG_MEM_BOOT_ADD1 00151 */ 00152 #define __HAL_SYSCFG_GET_BOOT_MODE() READ_BIT(SYSCFG->MEMRMP, SYSCFG_MEMRMP_MEM_BOOT) 00153 00154 #if defined (STM32F765xx) || defined (STM32F767xx) || defined (STM32F769xx) || defined (STM32F777xx) || defined (STM32F779xx) 00155 /** @brief SYSCFG Break Cortex-M7 Lockup lock. 00156 * Enable and lock the connection of Cortex-M7 LOCKUP (Hardfault) output to TIM1/8 Break input. 00157 * @note The selected configuration is locked and can be unlocked only by system reset. 00158 */ 00159 #define __HAL_SYSCFG_BREAK_LOCKUP_LOCK() SET_BIT(SYSCFG->CBR, SYSCFG_CBR_CLL) 00160 00161 /** @brief SYSCFG Break PVD lock. 00162 * Enable and lock the PVD connection to Timer1/8 Break input, as well as the PVDE and PLS[2:0] in the PWR_CR1 register. 00163 * @note The selected configuration is locked and can be unlocked only by system reset. 00164 */ 00165 #define __HAL_SYSCFG_BREAK_PVD_LOCK() SET_BIT(SYSCFG->CBR, SYSCFG_CBR_PVDL) 00166 #endif /* STM32F765xx || STM32F767xx || STM32F769xx || STM32F777xx || STM32F779xx */ 00167 00168 /** 00169 * @} 00170 */ 00171 00172 /* Exported functions --------------------------------------------------------*/ 00173 /** @addtogroup HAL_Exported_Functions 00174 * @{ 00175 */ 00176 /** @addtogroup HAL_Exported_Functions_Group1 00177 * @{ 00178 */ 00179 /* Initialization and de-initialization functions ******************************/ 00180 HAL_StatusTypeDef HAL_Init(void); 00181 HAL_StatusTypeDef HAL_DeInit(void); 00182 void HAL_MspInit(void); 00183 void HAL_MspDeInit(void); 00184 HAL_StatusTypeDef HAL_InitTick (uint32_t TickPriority); 00185 /** 00186 * @} 00187 */ 00188 00189 /** @addtogroup HAL_Exported_Functions_Group2 00190 * @{ 00191 */ 00192 /* Peripheral Control functions ************************************************/ 00193 void HAL_IncTick(void); 00194 void HAL_Delay(__IO uint32_t Delay); 00195 uint32_t HAL_GetTick(void); 00196 void HAL_SuspendTick(void); 00197 void HAL_ResumeTick(void); 00198 uint32_t HAL_GetHalVersion(void); 00199 uint32_t HAL_GetREVID(void); 00200 uint32_t HAL_GetDEVID(void); 00201 void HAL_DBGMCU_EnableDBGSleepMode(void); 00202 void HAL_DBGMCU_DisableDBGSleepMode(void); 00203 void HAL_DBGMCU_EnableDBGStopMode(void); 00204 void HAL_DBGMCU_DisableDBGStopMode(void); 00205 void HAL_DBGMCU_EnableDBGStandbyMode(void); 00206 void HAL_DBGMCU_DisableDBGStandbyMode(void); 00207 void HAL_EnableCompensationCell(void); 00208 void HAL_DisableCompensationCell(void); 00209 void HAL_EnableFMCMemorySwapping(void); 00210 void HAL_DisableFMCMemorySwapping(void); 00211 #if defined (STM32F765xx) || defined (STM32F767xx) || defined (STM32F769xx) || defined (STM32F777xx) || defined (STM32F779xx) 00212 void HAL_EnableMemorySwappingBank(void); 00213 void HAL_DisableMemorySwappingBank(void); 00214 #endif /* STM32F765xx || STM32F767xx || STM32F769xx || STM32F777xx || STM32F779xx */ 00215 /** 00216 * @} 00217 */ 00218 00219 /** 00220 * @} 00221 */ 00222 /* Private types -------------------------------------------------------------*/ 00223 /* Private variables ---------------------------------------------------------*/ 00224 /** @defgroup HAL_Private_Variables HAL Private Variables 00225 * @{ 00226 */ 00227 /** 00228 * @} 00229 */ 00230 /* Private constants ---------------------------------------------------------*/ 00231 /** @defgroup HAL_Private_Constants HAL Private Constants 00232 * @{ 00233 */ 00234 /** 00235 * @} 00236 */ 00237 /* Private macros ------------------------------------------------------------*/ 00238 /* Private functions ---------------------------------------------------------*/ 00239 /** 00240 * @} 00241 */ 00242 00243 /** 00244 * @} 00245 */ 00246 00247 #ifdef __cplusplus 00248 } 00249 #endif 00250 00251 #endif /* __STM32F7xx_HAL_H */ 00252 00253 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/ 00254
Generated on Wed Jul 13 2022 18:23:41 by
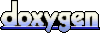