Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Fork of UniGraphic by
TFT_MIPI.cpp
00001 /* mbed UniGraphic library - Device specific class 00002 * Copyright (c) 2015 Giuliano Dianda 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 #include "Protocols.h " 00006 #include "TFT_MIPI.h" 00007 00008 ////////////////////////////////////////////////////////////////////////////////// 00009 // display settings /////////////////////////////////////////////////////// 00010 ///////////////////////////////////////////////////////////////////////// 00011 00012 // put in constructor 00013 //#define LCDSIZE_X 320 // display X pixels, TFTs are usually portrait view 00014 //#define LCDSIZE_Y 480 // display Y pixels 00015 00016 00017 00018 TFT_MIPI::TFT_MIPI(proto_t displayproto, PortName port, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const char *name , unsigned int LCDSIZE_X, unsigned int LCDSIZE_Y) 00019 : TFT(displayproto, port, CS, reset, DC, WR, RD, LCDSIZE_X, LCDSIZE_Y, name) 00020 { 00021 hw_reset(); 00022 BusEnable(true); 00023 identify(); // will collect tftID, set mipistd flag 00024 init(); 00025 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00026 // scrollbugfix=1; // when scrolling 1 line, the last line disappears, set to 1 to fix it, for ili9481 is set automatically in identify() 00027 set_orientation(0); 00028 // FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. Give a try 00029 cls(); 00030 locate(0,0); 00031 } 00032 TFT_MIPI::TFT_MIPI(proto_t displayproto, int Hz, PinName mosi, PinName miso, PinName sclk, PinName CS, PinName reset, PinName DC, const char *name , unsigned int LCDSIZE_X , unsigned int LCDSIZE_Y ) 00033 : TFT(displayproto, Hz, mosi, miso, sclk, CS, reset, DC, LCDSIZE_X, LCDSIZE_Y, name) 00034 { 00035 hw_reset(); //TFT class forwards to Protocol class 00036 BusEnable(true); //TFT class forwards to Protocol class 00037 identify(); // will collect tftID and set mipistd flag 00038 init(); // per display custom init cmd sequence, implemented here 00039 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00040 // scrollbugfix=1; // when scrolling 1 line, the last line disappears, set to 1 to fix it, for ili9481 is set automatically in identify() 00041 set_orientation(0); //TFT class does for MIPI standard and some ILIxxx 00042 // FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. Give a try 00043 cls(); 00044 locate(0,0); 00045 } 00046 // reset and init the lcd controller 00047 void TFT_MIPI::init() 00048 { 00049 /* Start Initial Sequence ----------------------------------------------------*/ 00050 00051 /* Start Initial Sequence ----------------------------------------------------*/ 00052 wr_cmd8(0xD0); // POWER SETTING 00053 wr_data8(0x07); 00054 wr_data8(0x42); 00055 wr_data8(0x18); 00056 00057 wr_cmd8(0xD1); // VCOM control 00058 wr_data8(0x00); 00059 wr_data8(0x07); 00060 wr_data8(0x10); 00061 00062 wr_cmd8(0xD2); // Power_Setting for Normal Mode 00063 wr_data8(0x01); // LCD power supply current 00064 wr_data8(0x02); // charge pumps 00065 00066 wr_cmd8(0xC0); // Panel Driving Setting 00067 wr_data8(0x10); // 10 orig 00068 wr_data8(0x3B); //number of lines+1 *8 00069 wr_data8(0x00); 00070 wr_data8(0x02); 00071 wr_data8(0x11); 00072 00073 // C1 missing? Display_Timing_Setting for Normal Mode 00074 00075 //renesas does not have this 00076 // wr_cmd8(0xC5); // Frame Rate and Inversion Control 00077 // wr_data8(0x03); // 72hz, datashet tells default 02=85hz 00078 00079 wr_cmd8(0xC8); // Gamma settings 00080 wr_data8(0x00); 00081 wr_data8(0x32); 00082 wr_data8(0x36); 00083 wr_data8(0x45); 00084 wr_data8(0x06); 00085 wr_data8(0x16); 00086 wr_data8(0x37); 00087 wr_data8(0x75); 00088 wr_data8(0x77); 00089 wr_data8(0x54); 00090 wr_data8(0x0C); 00091 wr_data8(0x00); 00092 00093 00094 00095 wr_cmd8(0x36); // MEMORY_ACCESS_CONTROL (orientation stuff) 00096 wr_data8(0x0A); // 0A as per chinese example (vertical flipped) 00097 00098 wr_cmd8(0x3A); // COLMOD_PIXEL_FORMAT_SET, not present in AN 00099 wr_data8(0x55); // 16 bit pixel 00100 00101 wr_cmd8(0x13); // Nomal Displaymode 00102 00103 wr_cmd8(0x11); // sleep out 00104 wait_ms(150); 00105 00106 wr_cmd8(0x29); // display on 00107 wait_ms(150); 00108 00109 }
Generated on Wed Jul 13 2022 21:19:22 by
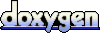