Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Fork of UniGraphic by
TFT932x.h
00001 #ifndef MBED_TFT932x_H 00002 #define MBED_TFT932x_H 00003 00004 #include "GraphicsDisplay.h" 00005 #include "PAR8.h" 00006 #include "PAR16.h" 00007 #include "BUS8.h" 00008 #include "BUS16.h" 00009 #include "SPI8.h" 00010 #include "SPI16.h" 00011 #include "Protocols.h " 00012 00013 00014 /** A custom base class for ILI932x color TFT Display (except ILI9327 which is MIPI standard) 00015 */ 00016 class TFT932x : public GraphicsDisplay 00017 { 00018 00019 public: 00020 00021 /** Create TFT Parallel Port interface 00022 * @param name The name used by the parent class to access the interface 00023 */ 00024 TFT932x(proto_t displayproto, PortName port, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const int lcdsize_x, const int lcdsize_y, const char* name); 00025 00026 /** Create TFT Parallel Bus interface 00027 * @param name The name used by the parent class to access the interface 00028 */ 00029 TFT932x(proto_t displayproto, PinName* buspins, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const int lcdsize_x, const int lcdsize_y, const char* name); 00030 00031 /** Create TFT SPI interface 00032 * @note ILI9325D has different SPI protocol, not supported here 00033 * @param name The name used by the parent class to access the interface 00034 */ 00035 TFT932x(proto_t displayproto, int Hz, PinName mosi, PinName miso, PinName sclk, PinName CS, PinName reset, const int lcdsize_x, const int lcdsize_y, const char* name); 00036 00037 /////// functions that come for free, but can be overwritten/////////////////////////////////////////////////// 00038 /////// ----------------------------------------------------/////////////////////////////////////////////////// 00039 00040 /** Draw a pixel in the specified color. 00041 * @param x is the horizontal offset to this pixel. 00042 * @param y is the vertical offset to this pixel. 00043 * @param color defines the color for the pixel. 00044 */ 00045 virtual void pixel(int x, int y, unsigned short color); 00046 00047 /** Set the window, which controls where items are written to the screen. 00048 * When something hits the window width, it wraps back to the left side 00049 * and down a row. If the initial write is outside the window, it will 00050 * be captured into the window when it crosses a boundary. 00051 * @param x is the left edge in pixels. 00052 * @param y is the top edge in pixels. 00053 * @param w is the window width in pixels. 00054 * @param h is the window height in pixels. 00055 */ 00056 virtual void window(int x, int y, int w, int h); 00057 00058 /** Read pixel color at location 00059 * @param x is the horizontal offset to this pixel. 00060 * @param y is the vertical offset to this pixel. 00061 * @returns 16bit color. 00062 */ 00063 virtual unsigned short pixelread(int x, int y); 00064 00065 /** Set the window from which gram is read from. Autoincrements row/column 00066 * @param x is the left edge in pixels. 00067 * @param y is the top edge in pixels. 00068 * @param w is the window width in pixels. 00069 * @param h is the window height in pixels. 00070 */ 00071 virtual void window4read(int x, int y, int w, int h); 00072 00073 /** Push a single pixel into the window and increment position. 00074 * You must first call window() then push pixels. 00075 * @param color is the pixel color. 00076 */ 00077 virtual void window_pushpixel(unsigned short color); 00078 00079 /** Push some pixels of the same color into the window and increment position. 00080 * You must first call window() then push pixels. 00081 * @param color is the pixel color. 00082 * @param count: how many 00083 */ 00084 virtual void window_pushpixel(unsigned short color, unsigned int count); 00085 00086 /** Push array of pixel colors into the window and increment position. 00087 * You must first call window() then push pixels. 00088 * @param color is the pixel color. 00089 */ 00090 virtual void window_pushpixelbuf(unsigned short* color, unsigned int lenght); 00091 00092 /** Framebuffer is not used for TFT 00093 */ 00094 virtual void copy_to_lcd(){ }; 00095 00096 /** display inverted colors 00097 * 00098 * @param o = 0 normal, 1 invert 00099 */ 00100 void invert(unsigned char o); 00101 00102 /** clear the entire screen 00103 * The inherited one sets windomax then fill with background color 00104 * We override it to speedup 00105 */ 00106 virtual void cls(); 00107 00108 /** Set the orientation of the screen 00109 * x,y: 0,0 is always top left 00110 * 00111 * @param o direction to use the screen (0-3) 00112 * 0 = default 0° portrait view 00113 * 1 = +90° landscape view 00114 * 2 = +180° portrait view 00115 * 3 = -90° landscape view 00116 * 00117 */ 00118 virtual void set_orientation(int o); 00119 00120 /** Set ChipSelect high or low 00121 * @param enable true/false 00122 */ 00123 virtual void BusEnable(bool enable); 00124 00125 /** Enable fast window (default disabled) 00126 * used to speedup functions that plots single pixels, like circle, oblique lines or just sparse pixels 00127 * @param enable true/false 00128 * @note most but not all controllers support this, even if datasheet tells they should 00129 */ 00130 void FastWindow(bool enable); 00131 00132 /** Enable scroll 00133 * scroll is done in hw but only on the native vertical axis 00134 * TFTs are mainly native protrait view, so horizontal scroll if rotated in landscape view 00135 * @note ILI932x does not allow partial screen scrolling, only full screen is selectable 00136 * @param startY unused, always 0 for ILI932x 00137 * @param areasize unused, always screensize_Y for ILI932x 00138 */ 00139 void setscrollarea (int startY=0, int areasize=0); 00140 00141 /** Scroll up(or left) the scrollarea 00142 * 00143 * @param lines number of lines to scroll, 1= scrollup 1, areasize-1= scrolldown 1 00144 */ 00145 void scroll (int lines); 00146 00147 /** Disable scroll and display un-scrolled screen 00148 * 00149 */ 00150 void scrollreset(); 00151 00152 /** get display X size in pixels (native, orientation independent) 00153 * @returns X size in pixels 00154 */ 00155 int sizeX(); 00156 00157 /** get display Y size in pixels (native, orientation independent) 00158 * @returns Y size in pixels 00159 */ 00160 int sizeY(); 00161 00162 unsigned int tftID; 00163 00164 00165 00166 00167 protected: 00168 00169 00170 ////// functions needed by parent class /////////////////////////////////////// 00171 ////// -------------------------------- /////////////////////////////////////// 00172 00173 /** ILI932x specific, does a dummy read cycle, number of bits is protocol dependent 00174 * for PAR protocols: a signle RD bit toggle 00175 * for SPI8: 8clocks 00176 * for SPI16: 16 clocks 00177 */ 00178 virtual void dummyread (); 00179 00180 /** ILI932x specific, select register for a successive write or read 00181 * 00182 * @param reg register to be selected 00183 * @param forread false = a write next (default), true = a read next 00184 * @note forread only used by SPI protocols 00185 */ 00186 virtual void reg_select(unsigned char reg, bool forread =false); 00187 00188 /** ILI932x specific, write register with data 00189 * 00190 * @param reg register to write 00191 * @param data 16bit data 00192 */ 00193 virtual void reg_write(unsigned char reg, unsigned short data); 00194 00195 /** ILI932x specific, read register 00196 * 00197 * @param reg register to be read 00198 * @returns 16bit register value 00199 */ 00200 virtual unsigned short reg_read(unsigned char reg); 00201 00202 /** Send 16bit pixeldata to display controller 00203 * 00204 * @param data: halfword to send 00205 * 00206 */ 00207 virtual void wr_gram(unsigned short data); 00208 00209 /** Send same 16bit pixeldata to display controller multiple times 00210 * 00211 * @param data: halfword to send 00212 * @param count: how many 00213 * 00214 */ 00215 virtual void wr_gram(unsigned short data, unsigned int count); 00216 00217 /** Send array of pixeldata shorts to display controller 00218 * 00219 * @param data: unsigned short pixeldata array 00220 * @param lenght: lenght (in shorts) 00221 * 00222 */ 00223 virtual void wr_grambuf(unsigned short* data, unsigned int lenght); 00224 00225 /** Read 16bit pixeldata from display controller (with dummy cycle) 00226 * 00227 * @note autoconverts 18to16bit based on display identify info 00228 * @returns 16bit color 00229 */ 00230 virtual unsigned short rd_gram(); 00231 00232 /** HW reset sequence (without display init commands) 00233 */ 00234 void hw_reset(); 00235 00236 /** Try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR 00237 * autoset internal flags so pixelread() will always return correct value. 00238 */ 00239 virtual void auto_gram_read_format(); 00240 00241 /** Try to identify display ID 00242 * @note support ILI9341,94xx, MIPI standard. May be be overridden in Init class for other specific IC 00243 */ 00244 virtual void identify(); 00245 00246 unsigned int scrollbugfix; 00247 00248 00249 00250 private: 00251 00252 Protocols* proto; 00253 const int screensize_X; 00254 const int screensize_Y; 00255 // pixel location 00256 int cur_x; 00257 int cur_y; 00258 // window location 00259 int win_x1; 00260 int win_x2; 00261 int win_y1; 00262 int win_y2; 00263 int orientation; 00264 bool dummycycles; 00265 bool usefastwindow; 00266 bool fastwindowready; 00267 bool fastwindowready4read; 00268 bool is18bit; 00269 bool isBGR; 00270 00271 }; 00272 00273 #endif
Generated on Wed Jul 13 2022 21:19:22 by
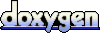