Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Fork of UniGraphic by
SSD1306.h
00001 #ifndef MBED_SSD1306_H 00002 #define MBED_SSD1306_H 00003 00004 #include "mbed.h" 00005 #include "LCD.h" 00006 00007 /** Class for SSD1306 display controller 00008 * to be copypasted and adapted for other controllers 00009 */ 00010 class SSD1306 : public LCD 00011 { 00012 00013 public: 00014 00015 /** Create a PAR display interface 00016 * @param displayproto only supports PAR_8 00017 * @param port GPIO port name to use 00018 * @param CS pin connected to CS of display 00019 * @param reset pin connected to RESET of display 00020 * @param DC pin connected to data/command of display 00021 * @param WR pin connected to SDI of display 00022 * @param RD pin connected to RS of display 00023 * @param name The name used by the parent class to access the interface 00024 * @param LCDSIZE_X x size in pixel - optional 00025 * @param LCDSIZE_Y y size in pixel - optional 00026 */ 00027 SSD1306(proto_t displayproto, PortName port, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const char* name, unsigned int LCDSIZE_X = 128, unsigned int LCDSIZE_Y = 64); 00028 00029 /** Create an SPI display interface 00030 * @param displayproto SPI_8 or SPI_16 00031 * @param Hz SPI speed in Hz 00032 * @param mosi SPI pin 00033 * @param miso SPI pin 00034 * @param sclk SPI pin 00035 * @param CS pin connected to CS of display 00036 * @param reset pin connected to RESET of display 00037 * @param DC pin connected to data/command of display 00038 * @param name The name used by the parent class to access the interface 00039 * @param LCDSIZE_X x size in pixel - optional 00040 * @param LCDSIZE_Y y size in pixel - optional 00041 */ 00042 SSD1306(proto_t displayproto, int Hz, PinName mosi, PinName miso, PinName sclk, PinName CS, PinName reset, PinName DC, const char* name , unsigned int LCDSIZE_X = 128, unsigned int LCDSIZE_Y = 64); 00043 00044 /** set the contrast of the screen 00045 * @note here overrided because of not standard value range 00046 * @param o contrast 0-255 00047 */ 00048 virtual void set_contrast(int o); 00049 00050 /** set automatc horizontal scroll mode 00051 * @param l_r direction - left = 0, right = 1 00052 * @param s_page start page 00053 * @param e_page end page 00054 * @param speed time between horizontal shift. 0 slow .. 7 fast 00055 */ 00056 void horizontal_scroll(int l_r,int s_page,int e_page,int speed); 00057 00058 /** automatic horizontal + vertical scroll mode 00059 * @param l_r direction - left = 0, right = 1 00060 * @param s_page start page 00061 * @param e_page end page 00062 * @param v_off vertical offset for scroll 00063 * @param speed time between horizontal shift. 0 slow .. 7 fast 00064 */ 00065 void horiz_vert_scroll(int l_r,int s_page,int e_page,int v_off,int speed); 00066 00067 /** end scroll mode 00068 * 00069 */ 00070 void end_scroll(void); 00071 protected: 00072 00073 00074 /** Init command sequence 00075 */ 00076 void init(); 00077 00078 /** set mirror mode 00079 * @note here overriding the LCD class default one because of not standard commands 00080 * @param mode NONE, X, Y, XY 00081 */ 00082 virtual void mirrorXY(mirror_t mode); 00083 00084 }; 00085 00086 00087 #endif
Generated on Wed Jul 13 2022 21:19:22 by
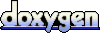