Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Fork of UniGraphic by
Protocols.h
00001 /* mbed UniGraphic library - Abstract protocol class 00002 * Copyright (c) 2015 Giuliano Dianda 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 /** @file Protocols.h 00007 */ 00008 #ifndef Protocols_H 00009 #define Protocols_H 00010 00011 #include "mbed.h" 00012 00013 #define RGB24to16(r,g,b) (((r&0xF8)<<8)|((g&0xFC)<<3)|((b&0xF8)>>3)) //5 red | 6 green | 5 blue 00014 #define BGR2RGB(color) (((color&0x1F)<<11) | (color&0x7E0) | ((color&0xF800)>>11)) 00015 00016 //#define USE_CS 00017 00018 /** Protocol types 00019 */ 00020 enum proto_t { 00021 PAR_8 /**< Parallel 8bit, port pins 0 to 7 */ 00022 ,PAR_16 /**< Parallel 16bit, port pins 0 to 15 */ 00023 ,BUS_8 /**< Parallel 8bit, scattered pins */ 00024 ,BUS_16 /**< Parallel 16bit, scattered pins */ 00025 ,SPI_8 /**< SPI 8bit */ 00026 ,SPI_16 /**< SPI 16bit */ 00027 }; 00028 00029 00030 /** Abstract interface class for spi and parallel protocols 00031 */ 00032 class Protocols 00033 { 00034 public: 00035 00036 /** Send 8bit command to display controller 00037 * 00038 * @param cmd: byte to send 00039 * 00040 */ 00041 virtual void wr_cmd8(unsigned char cmd) = 0; 00042 00043 /** Send 8bit data to display controller 00044 * 00045 * @param data: byte to send 00046 * 00047 */ 00048 virtual void wr_data8(unsigned char data) = 0; 00049 00050 /** Send 2x8bit command to display controller 00051 * 00052 * @param cmd: halfword to send 00053 * 00054 */ 00055 virtual void wr_cmd16(unsigned short cmd) = 0; 00056 00057 /** Send 2x8bit data to display controller 00058 * 00059 * @param data: halfword to send 00060 * 00061 */ 00062 virtual void wr_data16(unsigned short data) = 0; 00063 00064 /** Send 16bit pixeldata to display controller 00065 * 00066 * @param data: halfword to send 00067 * 00068 */ 00069 virtual void wr_gram(unsigned short data) = 0; 00070 00071 /** Send same 16bit pixeldata to display controller multiple times 00072 * 00073 * @param data: halfword to send 00074 * @param count: how many 00075 * 00076 */ 00077 virtual void wr_gram(unsigned short data, unsigned int count) = 0; 00078 00079 /** Send array of pixeldata shorts to display controller 00080 * 00081 * @param data: unsigned short pixeldata array 00082 * @param lenght: lenght (in shorts) 00083 * 00084 */ 00085 virtual void wr_grambuf(unsigned short* data, unsigned int lenght) = 0; 00086 00087 /** Read 16bit pixeldata from display controller (with dummy cycle) 00088 * 00089 * @param convert true/false. Convert 18bit to 16bit, some controllers returns 18bit 00090 * @returns 16bit color 00091 */ 00092 virtual unsigned short rd_gram(bool convert) = 0; 00093 00094 /** Read 4x8bit register data (with dummy cycle) 00095 * @param reg the register to read 00096 * @returns data as uint 00097 * 00098 */ 00099 virtual unsigned int rd_reg_data32(unsigned char reg) = 0; 00100 00101 /** Read 3x8bit ExtendedCommands register data 00102 * @param reg the register to read 00103 * @param SPIreadenablecmd vendor/device specific cmd to read EXTC registers 00104 * @returns data as uint 00105 * @note EXTC regs (0xB0 to 0xFF) are read/write registers but needs special cmd to be read in SPI mode 00106 */ 00107 virtual unsigned int rd_extcreg_data32(unsigned char reg, unsigned char SPIreadenablecmd) = 0; 00108 00109 /** ILI932x specific, does a dummy read cycle, number of bits is protocol dependent 00110 * for PAR protocols: a signle RD bit toggle 00111 * for SPI8: 8clocks 00112 * for SPI16: 16 clocks 00113 */ 00114 virtual void dummyread () = 0; 00115 00116 /** ILI932x specific, select register for a successive write or read 00117 * 00118 * @param reg register to be selected 00119 * @param forread false = a write next (default), true = a read next 00120 * @note forread only used by SPI protocols 00121 */ 00122 virtual void reg_select(unsigned char reg, bool forread =false) = 0; 00123 00124 /** ILI932x specific, write register with data 00125 * 00126 * @param reg register to write 00127 * @param data 16bit data 00128 */ 00129 virtual void reg_write(unsigned char reg, unsigned short data) = 0; 00130 00131 /** ILI932x specific, read register 00132 * 00133 * @param reg register to be read 00134 * @returns 16bit register value 00135 */ 00136 virtual unsigned short reg_read(unsigned char reg) = 0; 00137 00138 /** HW reset sequence (without display init commands) 00139 */ 00140 virtual void hw_reset() = 0; 00141 00142 /** Set ChipSelect high or low 00143 * @param enable 0/1 00144 */ 00145 virtual void BusEnable(bool enable) = 0; 00146 00147 }; 00148 #endif
Generated on Wed Jul 13 2022 21:19:22 by
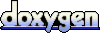