Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Fork of UniGraphic by
PAR16.cpp
00001 /* mbed UniGraphic library - PAR16 protocol class 00002 * Copyright (c) 2015 Giuliano Dianda 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 * 00005 * Derived work of: 00006 * 00007 * mbed library for 240*320 pixel display TFT based on ILI9341 LCD Controller 00008 * Copyright (c) 2013 Peter Drescher - DC2PD 00009 * 00010 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00011 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00012 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00013 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00014 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00015 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00016 * THE SOFTWARE. 00017 */ 00018 #include "PAR16.h" 00019 00020 PAR16::PAR16(PortName port, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD) 00021 : _port(port,0xFFFF), _CS(CS), _reset(reset), _DC(DC), _WR(WR), _RD(RD) 00022 { 00023 _reset = 1; 00024 _DC=1; 00025 _WR=1; 00026 _RD=1; 00027 _CS=1; 00028 _port.mode(PullNone); 00029 _port.output(); // will re-enable our GPIO port 00030 hw_reset(); 00031 } 00032 00033 void PAR16::wr_cmd8(unsigned char cmd) 00034 { 00035 _DC = 0; // 0=cmd 00036 _port.write(cmd); // write 8bit 00037 _WR=0; 00038 _WR=1; 00039 _DC = 1; // 1=data next 00040 } 00041 void PAR16::wr_data8(unsigned char data) 00042 { 00043 _port.write(data); // write 8bit 00044 _WR=0; 00045 _WR=1; 00046 } 00047 void PAR16::wr_cmd16(unsigned short cmd) 00048 { 00049 _DC = 0; // 0=cmd 00050 _port.write(cmd>>8); // write 8bit 00051 _WR=0; 00052 _WR=1; 00053 _port.write(cmd&0xFF); // write 8bit 00054 _WR=0; 00055 _WR=1; 00056 _DC = 1; // 1=data next 00057 } 00058 void PAR16::wr_data16(unsigned short data) 00059 { 00060 _port.write(data>>8); // write 8bit 00061 _WR=0; 00062 _WR=1; 00063 _port.write(data&0xFF); // write 8bit 00064 _WR=0; 00065 _WR=1; 00066 } 00067 void PAR16::wr_gram(unsigned short data) 00068 { 00069 _port.write(data); // write 16bit 00070 _WR=0; 00071 _WR=1; 00072 } 00073 void PAR16::wr_gram(unsigned short data, unsigned int count) 00074 { 00075 while(count) 00076 { 00077 _port.write(data); // rewrite even if same data, otherwise too much fast 00078 _WR=0; 00079 _WR=1; 00080 count--; 00081 } 00082 } 00083 void PAR16::wr_grambuf(unsigned short* data, unsigned int lenght) 00084 { 00085 while(lenght) 00086 { 00087 _port.write(*data); // write 16bit 00088 _WR=0; 00089 _WR=1; 00090 data++; 00091 lenght--; 00092 } 00093 } 00094 unsigned short PAR16::rd_gram(bool convert) 00095 { 00096 unsigned int r=0; 00097 _port.input(); 00098 00099 _RD = 0; 00100 _port.read(); //dummy read 00101 _RD = 1; 00102 00103 _RD = 0; 00104 // _RD = 0; // add wait 00105 r |= _port.read(); 00106 _RD = 1; 00107 if(convert) 00108 { 00109 r <<= 8; 00110 _RD = 0; 00111 // _RD = 0; // add wait 00112 r |= _port.read()>>8; //MSB of port read is blue, LSB is red of next pixel 00113 _RD = 1; 00114 // gram is 18bit/pixel, if you set 16bit/pixel (cmd 3A), during writing the 16bits are expanded to 18bit 00115 // during reading, you read the raw 18bit gram 00116 r = RGB24to16((r&0xFF0000)>>16, (r&0xFF00)>>8, r&0xFF);// 18bit pixel padded to 24bits, rrrrrr00_gggggg00_bbbbbb00, converted to 16bit 00117 } 00118 _port.output(); 00119 return (unsigned short)r; 00120 } 00121 unsigned int PAR16::rd_reg_data32(unsigned char reg) 00122 { 00123 wr_cmd8(reg); 00124 unsigned int r=0; 00125 // _DC = 1; // 1=data 00126 _port.input(); 00127 00128 _RD = 0; 00129 _port.read(); //dummy read 00130 _RD = 1; 00131 00132 _RD = 0; 00133 // _RD = 0; // add wait 00134 r |= (_port.read()&0xFF); 00135 r <<= 8; 00136 _RD = 1; 00137 00138 _RD = 0; 00139 // _RD = 0; // add wait 00140 r |= (_port.read()&0xFF); 00141 r <<= 8; 00142 _RD = 1; 00143 00144 _RD = 0; 00145 // _RD = 0; // add wait 00146 r |= (_port.read()&0xFF); 00147 r <<= 8; 00148 _RD = 1; 00149 00150 _RD = 0; 00151 // _RD = 0; // add wait 00152 r |= (_port.read()&0xFF); 00153 _RD = 1; 00154 00155 _CS = 1; // toggle CS to interupt the cmd in case was not supported 00156 _CS = 0; 00157 00158 _port.output(); 00159 return r; 00160 } 00161 // in Par mode EXTC regs (0xB0-0xFF) can be directly read 00162 unsigned int PAR16::rd_extcreg_data32(unsigned char reg, unsigned char SPIreadenablecmd) 00163 { 00164 return rd_reg_data32(reg); 00165 } 00166 // ILI932x specific 00167 void PAR16::dummyread() 00168 { 00169 _port.input(); 00170 _RD = 0; 00171 _port.read(); // dummy read 00172 _RD=1; 00173 // _port.output(); 00174 } 00175 // ILI932x specific 00176 void PAR16::reg_select(unsigned char reg, bool forread) 00177 { 00178 _DC = 0; 00179 _port.write(reg); // write 16bit 00180 _WR=0; 00181 _WR=1; 00182 _DC = 1; // 1=data next 00183 } 00184 // ILI932x specific 00185 void PAR16::reg_write(unsigned char reg, unsigned short data) 00186 { 00187 _DC = 0; 00188 _port.write(reg); // write 16bit 00189 _WR=0; 00190 _WR=1; 00191 _DC = 1; 00192 _port.write(data); // write 16bit 00193 _WR=0; 00194 _WR=1; 00195 } 00196 // ILI932x specific 00197 unsigned short PAR16::reg_read(unsigned char reg) 00198 { 00199 unsigned short r=0; 00200 _DC = 0; 00201 _port.write(reg); // write 16bit 00202 _WR=0; 00203 _WR=1; 00204 _DC = 1; 00205 _port.input(); 00206 _RD=0; 00207 r |= _port.read(); // read 16bit 00208 _RD=1; 00209 _port.output(); 00210 return r; 00211 } 00212 void PAR16::hw_reset() 00213 { 00214 wait_ms(15); 00215 _DC = 1; 00216 _CS = 1; 00217 _WR = 1; 00218 _RD = 1; 00219 _reset = 0; // display reset 00220 wait_ms(2); 00221 _reset = 1; // end reset 00222 wait_ms(100); 00223 } 00224 void PAR16::BusEnable(bool enable) 00225 { 00226 _CS = enable ? 0:1; 00227 }
Generated on Wed Jul 13 2022 21:19:22 by
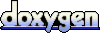