Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Fork of UniGraphic by
ILI9486.cpp
00001 /* mbed UniGraphic library - Device specific class 00002 * Copyright (c) 2015 Giuliano Dianda 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 #include "Protocols.h " 00006 #include "ILI9486.h" 00007 00008 ////////////////////////////////////////////////////////////////////////////////// 00009 // display settings /////////////////////////////////////////////////////// 00010 ///////////////////////////////////////////////////////////////////////// 00011 00012 // put in constructor 00013 //#define LCDSIZE_X 320 // display X pixels, TFTs are usually portrait view 00014 //#define LCDSIZE_Y 480 // display Y pixels 00015 00016 00017 00018 ILI9486::ILI9486(proto_t displayproto, PortName port, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const char *name , unsigned int LCDSIZE_X, unsigned int LCDSIZE_Y) 00019 : TFT(displayproto, port, CS, reset, DC, WR, RD, LCDSIZE_X, LCDSIZE_Y, name) 00020 { 00021 hw_reset(); 00022 BusEnable(true); 00023 identify(); // will collect tftID and set mipistd flag 00024 init(); 00025 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00026 set_orientation(0); 00027 // FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. ILI9486 does not, at least in par mode 00028 cls(); 00029 locate(0,0); 00030 } 00031 ILI9486::ILI9486(proto_t displayproto, int Hz, PinName mosi, PinName miso, PinName sclk, PinName CS, PinName reset, PinName DC, const char *name, unsigned int LCDSIZE_X, unsigned int LCDSIZE_Y) 00032 : TFT(displayproto, Hz, mosi, miso, sclk, CS, reset, DC, LCDSIZE_X, LCDSIZE_Y, name) 00033 { 00034 hw_reset(); //TFT class forwards to Protocol class 00035 BusEnable(true); //TFT class forwards to Protocol class 00036 identify(); // will collect tftID and set mipistd flag 00037 init(); // per display custom init cmd sequence, implemented here 00038 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00039 set_orientation(0); //TFT class does for MIPI standard and some ILIxxx 00040 // FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. ILI9486 does not, at least in par mode 00041 cls(); 00042 locate(0,0); 00043 } 00044 // reset and init the lcd controller 00045 void ILI9486::init() 00046 { 00047 /* Start Initial Sequence ----------------------------------------------------*/ 00048 00049 wr_cmd8(0xF1); 00050 wr_data8(0x36); 00051 wr_data8(0x04); 00052 wr_data8(0x00); 00053 wr_data8(0x3C); 00054 wr_data8(0x0F); 00055 wr_data8(0x8F); 00056 00057 00058 wr_cmd8(0xF2); 00059 wr_data8(0x18); 00060 wr_data8(0xA3); 00061 wr_data8(0x12); 00062 wr_data8(0x02); 00063 wr_data8(0xb2); 00064 wr_data8(0x12); 00065 wr_data8(0xFF); 00066 wr_data8(0x10); 00067 wr_data8(0x00); 00068 00069 wr_cmd8(0xF8); 00070 wr_data8(0x21); 00071 wr_data8(0x04); 00072 00073 wr_cmd8(0xF9); 00074 wr_data8(0x00); 00075 wr_data8(0x08); 00076 00077 wr_cmd8(0xC0); 00078 wr_data8(0x0f); //13 00079 wr_data8(0x0f); //10 00080 00081 wr_cmd8(0xC1); 00082 wr_data8(0x42); //43 00083 00084 wr_cmd8(0xC2); 00085 wr_data8(0x22); 00086 00087 wr_cmd8(0xC5); 00088 wr_data8(0x01); //00 00089 wr_data8(0x29); //4D 00090 wr_data8(0x80); 00091 00092 wr_cmd8(0xB6); 00093 wr_data8(0x00); 00094 wr_data8(0x02); //42 00095 wr_data8(0x3b); 00096 00097 wr_cmd8(0xB1); 00098 wr_data8(0xB0); //C0 00099 wr_data8(0x11); 00100 00101 wr_cmd8(0xB4); 00102 wr_data8(0x02); //01 00103 00104 wr_cmd8(0xE0); 00105 wr_data8(0x0F); 00106 wr_data8(0x18); 00107 wr_data8(0x15); 00108 wr_data8(0x09); 00109 wr_data8(0x0B); 00110 wr_data8(0x04); 00111 wr_data8(0x49); 00112 wr_data8(0x64); 00113 wr_data8(0x3D); 00114 wr_data8(0x08); 00115 wr_data8(0x15); 00116 wr_data8(0x06); 00117 wr_data8(0x12); 00118 wr_data8(0x07); 00119 wr_data8(0x00); 00120 00121 wr_cmd8(0xE1); 00122 wr_data8(0x0F); 00123 wr_data8(0x38); 00124 wr_data8(0x35); 00125 wr_data8(0x0a); 00126 wr_data8(0x0c); 00127 wr_data8(0x03); 00128 wr_data8(0x4A); 00129 wr_data8(0x42); 00130 wr_data8(0x36); 00131 wr_data8(0x04); 00132 wr_data8(0x0F); 00133 wr_data8(0x03); 00134 wr_data8(0x1F); 00135 wr_data8(0x1B); 00136 wr_data8(0x00); 00137 00138 wr_cmd8(0x20); // display inversion OFF 00139 00140 wr_cmd8(0x36); // MEMORY_ACCESS_CONTROL (orientation stuff) 00141 wr_data8(0x48); 00142 00143 wr_cmd8(0x3A); // COLMOD_PIXEL_FORMAT_SET 00144 wr_data8(0x55); // 16 bit pixel 00145 00146 wr_cmd8(0x13); // Nomal Displaymode 00147 00148 wr_cmd8(0x11); // sleep out 00149 wait_ms(150); 00150 00151 wr_cmd8(0x29); // display on 00152 wait_ms(150); 00153 }
Generated on Wed Jul 13 2022 21:19:22 by
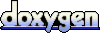