Basically i glued Peter Drescher and Simon Ford libs in a GraphicsDisplay class, then derived TFT or LCD class (which inherits Protocols class), then the most derived ones (Inits), which are per-display and are the only part needed to be adapted to diff hw.
Fork of UniGraphic by
ILI932x.cpp
00001 /* mbed UniGraphic library - Device specific class 00002 * Copyright (c) 2015 Giuliano Dianda 00003 * Released under the MIT License: http://mbed.org/license/mit 00004 */ 00005 00006 #include "Protocols.h " 00007 #include "ILI932x.h" 00008 00009 ////////////////////////////////////////////////////////////////////////////////// 00010 // display settings /////////////////////////////////////////////////////// 00011 ///////////////////////////////////////////////////////////////////////// 00012 00013 00014 ILI932x::ILI932x(proto_t displayproto, PortName port, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const char *name , unsigned int LCDSIZE_X, unsigned int LCDSIZE_Y) 00015 : TFT932x(displayproto, port, CS, reset, DC, WR, RD, LCDSIZE_X, LCDSIZE_Y, name) 00016 { 00017 hw_reset(); 00018 BusEnable(true); //set CS low, will stay low untill manually set high with BusEnable(false); 00019 identify(); // will collect tftID 00020 if(tftID==0x9325) init9325(); 00021 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00022 set_orientation(0); 00023 FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. 00024 cls(); 00025 locate(0,0); 00026 } 00027 ILI932x::ILI932x(proto_t displayproto, PinName* buspins, PinName CS, PinName reset, PinName DC, PinName WR, PinName RD, const char *name , unsigned int LCDSIZE_X, unsigned int LCDSIZE_Y) 00028 : TFT932x(displayproto, buspins, CS, reset, DC, WR, RD, LCDSIZE_X, LCDSIZE_Y, name) 00029 { 00030 hw_reset(); 00031 BusEnable(true); //set CS low, will stay low untill manually set high with BusEnable(false); 00032 identify(); // will collect tftID 00033 if(tftID==0x9325) init9325(); 00034 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00035 set_orientation(0); 00036 FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. 00037 cls(); 00038 locate(0,0); 00039 } 00040 ILI932x::ILI932x(proto_t displayproto, int Hz, PinName mosi, PinName miso, PinName sclk, PinName CS, PinName reset, const char *name, unsigned int LCDSIZE_X, unsigned int LCDSIZE_Y) 00041 : TFT932x(displayproto, Hz, mosi, miso, sclk, CS, reset, LCDSIZE_X, LCDSIZE_Y, name) 00042 { 00043 hw_reset(); //TFT class forwards to Protocol class 00044 BusEnable(true); //set CS low, TFT932x class will toggle CS every transfer 00045 identify(); // will collect tftID 00046 if(tftID==0x9325) init9325(); 00047 auto_gram_read_format();// try to get read gram pixel format, could be 16bit or 18bit, RGB or BGR. Will set flags accordingly 00048 set_orientation(0); 00049 FastWindow(true); // most but not all controllers support this, even if datasheet tells they should. 00050 cls(); 00051 locate(0,0); 00052 } 00053 // reset and init the lcd controller 00054 00055 void ILI932x::init9325() 00056 { 00057 /* Example for ILI9325 ----------------------------------------------------*/ 00058 00059 reg_write(0x0001,0x0100); 00060 reg_write(0x0002,0x0700); 00061 reg_write(0x0003,0x1030); 00062 reg_write(0x0004,0x0000); 00063 reg_write(0x0008,0x0207); 00064 reg_write(0x0009,0x0000); 00065 reg_write(0x000A,0x0000); 00066 reg_write(0x000C,0x0000); 00067 reg_write(0x000D,0x0000); 00068 reg_write(0x000F,0x0000); 00069 //power on sequence VGHVGL 00070 reg_write(0x0010,0x0000); 00071 reg_write(0x0011,0x0007); 00072 reg_write(0x0012,0x0000); 00073 reg_write(0x0013,0x0000); 00074 reg_write(0x0007,0x0001); 00075 wait_ms(200); 00076 //vgh 00077 reg_write(0x0010,0x1290); 00078 reg_write(0x0011,0x0227); 00079 wait_ms(50); 00080 //vregiout 00081 reg_write(0x0012,0x001d); //0x001b 00082 wait_ms(50); 00083 //vom amplitude 00084 reg_write(0x0013,0x1500); 00085 wait_ms(50); 00086 //vom H 00087 reg_write(0x0029,0x0018); 00088 reg_write(0x002B,0x000D); 00089 wait_ms(50); 00090 //gamma 00091 reg_write(0x0030,0x0004); 00092 reg_write(0x0031,0x0307); 00093 reg_write(0x0032,0x0002);// 0006 00094 reg_write(0x0035,0x0206); 00095 reg_write(0x0036,0x0408); 00096 reg_write(0x0037,0x0507); 00097 reg_write(0x0038,0x0204);//0200 00098 reg_write(0x0039,0x0707); 00099 reg_write(0x003C,0x0405);// 0504 00100 reg_write(0x003D,0x0F02); 00101 //ram 00102 reg_write(0x0050,0x0000); 00103 reg_write(0x0051,0x00EF); 00104 reg_write(0x0052,0x0000); 00105 reg_write(0x0053,0x013F); 00106 reg_write(0x0060,0xA700); 00107 reg_write(0x0061,0x0001); 00108 reg_write(0x006A,0x0000); 00109 // 00110 reg_write(0x0080,0x0000); 00111 reg_write(0x0081,0x0000); 00112 reg_write(0x0082,0x0000); 00113 reg_write(0x0083,0x0000); 00114 reg_write(0x0084,0x0000); 00115 reg_write(0x0085,0x0000); 00116 // 00117 reg_write(0x0090,0x0010); 00118 reg_write(0x0092,0x0600); 00119 reg_write(0x0093,0x0003); 00120 reg_write(0x0095,0x0110); 00121 reg_write(0x0097,0x0000); 00122 reg_write(0x0098,0x0000); 00123 00124 reg_write(0x0007,0x0133); // display on 00125 00126 }
Generated on Wed Jul 13 2022 21:19:22 by
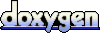