user config
Fork of TextLCD by
Embed:
(wiki syntax)
Show/hide line numbers
TextLCD.h
00001 /* mbed TextLCD Library, for a 4-bit LCD based on HD44780 00002 * Copyright (c) 2007-2010, sford, http://mbed.org 00003 * 2013, v01: WH, Added LCD types, fixed LCD address issues, added Cursor and UDCs 00004 * 2013, v02: WH, Added I2C and SPI bus interfaces 00005 * 2013, v03: WH, Added support for LCD40x4 which uses 2 controllers 00006 * 2013, v04: WH, Added support for Display On/Off, improved 4bit bootprocess 00007 * 2013, v05: WH, Added support for 8x2B, added some UDCs 00008 * 2013, v06: WH, Added support for devices that use internal DC/DC converters 00009 * 2013, v07: WH, Added support for backlight and include portdefinitions for LCD2004 Module from DFROBOT 00010 * 2014, v08: WH, Refactored in Base and Derived Classes to deal with mbed lib change regarding 'NC' defined DigitalOut pins 00011 * 2014, v09: WH/EO, Added Class for Native SPI controllers such as ST7032 00012 * 2014, v10: WH, Added Class for Native I2C controllers such as ST7032i, Added support for MCP23008 I2C portexpander, Added support for Adafruit module 00013 * 2014, v11: WH, Added support for native I2C controllers such as PCF21XX, Improved the _initCtrl() method to deal with differences between all supported controllers 00014 * 2014, v12: WH, Added support for native I2C controller PCF2119 and native I2C/SPI controllers SSD1803, ST7036, added setContrast method (by JH1PJL) for supported devices (eg ST7032i) 00015 * 2014, v13: WH, Added support for controllers US2066/SSD1311 (OLED), added setUDCBlink() method for supported devices (eg SSD1803), fixed issue in setPower() 00016 * 2014, v14: WH, Added support for PT6314 (VFD), added setOrient() method for supported devices (eg SSD1803, US2066), added Double Height lines for supported devices, 00017 * added 16 UDCs for supported devices (eg PCF2103), moved UDC defines to TextLCD_UDC file, added TextLCD_Config.h for feature and footprint settings. 00018 * 2014, v15: WH, Added AC780 support, added I2C expander modules, fixed setBacklight() for inverted logic modules. Fixed bug in LCD_SPI_N define 00019 * 2014, v16: WH, Added ST7070 and KS0073 support, added setIcon(), clrIcon() and setInvert() method for supported devices 00020 * 00021 * Permission is hereby granted, free of charge, to any person obtaining a copy 00022 * of this software and associated documentation files (the "Software"), to deal 00023 * in the Software without restriction, including without limitation the rights 00024 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00025 * copies of the Software, and to permit persons to whom the Software is 00026 * furnished to do so, subject to the following conditions: 00027 * 00028 * The above copyright notice and this permission notice shall be included in 00029 * all copies or substantial portions of the Software. 00030 * 00031 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00032 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00033 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00034 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00035 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00036 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00037 * THE SOFTWARE. 00038 */ 00039 00040 #ifndef MBED_TEXTLCD_H 00041 #define MBED_TEXTLCD_H 00042 00043 #include "mbed.h" 00044 #include "TextLCD_Config.h" 00045 #include "TextLCD_UDC.h" 00046 00047 /** A TextLCD interface for driving 4-bit HD44780-based LCDs 00048 * 00049 * Currently supports 8x1, 8x2, 12x3, 12x4, 16x1, 16x2, 16x3, 16x4, 20x2, 20x4, 24x1, 24x2, 24x4, 40x2 and 40x4 panels. 00050 * Interface options include direct mbed pins, I2C portexpander (PCF8474/PCF8574A or MCP23008) or SPI bus shiftregister (74595). 00051 * Supports some controllers with native I2C or SPI interface. Supports some controllers that provide internal DC/DC converters for VLCD or VLED. 00052 * Supports some controllers that feature programmable contrast control, powerdown, blinking UDCs and/or top/down orientation modes. 00053 * 00054 * @code 00055 * #include "mbed.h" 00056 * #include "TextLCD.h" 00057 * 00058 * // I2C Communication 00059 * I2C i2c_lcd(p28,p27); // SDA, SCL 00060 * 00061 * // SPI Communication 00062 * SPI spi_lcd(p5, NC, p7); // MOSI, MISO, SCLK 00063 * 00064 * //TextLCD lcd(p15, p16, p17, p18, p19, p20); // RS, E, D4-D7, LCDType=LCD16x2, BL=NC, E2=NC, LCDTCtrl=HD44780 00065 * //TextLCD_SPI lcd(&spi_lcd, p8, TextLCD::LCD40x4); // SPI bus, 74595 expander, CS pin, LCD Type 00066 * TextLCD_I2C lcd(&i2c_lcd, 0x42, TextLCD::LCD20x4); // I2C bus, PCF8574 Slaveaddress, LCD Type 00067 * //TextLCD_I2C lcd(&i2c_lcd, 0x42, TextLCD::LCD16x2, TextLCD::WS0010); // I2C bus, PCF8574 Slaveaddress, LCD Type, Device Type (OLED) 00068 * //TextLCD_SPI_N lcd(&spi_lcd, p8, p9); // SPI bus, CS pin, RS pin, LCDType=LCD16x2, BL=NC, LCDTCtrl=ST7032_3V3 00069 * //TextLCD_I2C_N lcd(&i2c_lcd, ST7032_SA, TextLCD::LCD16x2, NC, TextLCD::ST7032_3V3); // I2C bus, Slaveaddress, LCD Type, BL=NC, LCDTCtrl=ST7032_3V3 00070 * //TextLCD_SPI_N_3_24 lcd(&spi_lcd, p8, TextLCD::LCD20x4D, NC, TextLCD::SSD1803_3V3); // SPI bus, CS pin, LCDType=LCD20x4D, BL=NC, LCDTCtrl=SSD1803 00071 * //TextLCD_SPI_N_3_24 lcd(&spi_lcd, p8, TextLCD::LCD20x2, NC, TextLCD::US2066_3V3); // SPI bus, CS pin, LCDType=LCD20x2, BL=NC, LCDTCtrl=US2066 (OLED) 00072 * 00073 * int main() { 00074 * lcd.printf("Hello World!\n"); 00075 * } 00076 * @endcode 00077 */ 00078 00079 //The TextLCD_Config.h file selects hardware interface options to reduce memory footprint 00080 //and provides Pin Defines for I2C PCF8574/PCF8574A or MCP23008 and SPI 74595 bus expander interfaces. 00081 //The LCD and serial portexpanders should be wired accordingly. 00082 00083 /* LCD Type information on Rows, Columns and Variant. This information is encoded in 00084 * an int and used for the LCDType enumerators in order to simplify code maintenance */ 00085 // Columns encoded in b7..b0 00086 #define LCD_T_COL_MSK 0x000000FF 00087 #define LCD_T_C6 0x00000006 00088 #define LCD_T_C8 0x00000008 00089 #define LCD_T_C10 0x0000000A 00090 #define LCD_T_C12 0x0000000C 00091 #define LCD_T_C16 0x00000010 00092 #define LCD_T_C20 0x00000014 00093 #define LCD_T_C24 0x00000018 00094 #define LCD_T_C32 0x00000020 00095 #define LCD_T_C40 0x00000028 00096 00097 // Rows encoded in b15..b8 00098 #define LCD_T_ROW_MSK 0x0000FF00 00099 #define LCD_T_R1 0x00000100 00100 #define LCD_T_R2 0x00000200 00101 #define LCD_T_R3 0x00000300 00102 #define LCD_T_R4 0x00000400 00103 00104 // Addressing mode encoded in b19..b16 00105 #define LCD_T_ADR_MSK 0x000F0000 00106 #define LCD_T_A 0x00000000 /*Mode A Default 1, 2 or 4 line display */ 00107 #define LCD_T_B 0x00010000 /*Mode B, Alternate 8x2 (actually 16x1 display) */ 00108 #define LCD_T_C 0x00020000 /*Mode C, Alternate 16x1 (actually 8x2 display) */ 00109 #define LCD_T_D 0x00030000 /*Mode D, Alternate 3 or 4 line display (12x4, 20x4, 24x4) */ 00110 #define LCD_T_D1 0x00040000 /*Mode D1, Alternate 3 out of 4 line display (12x3, 20x3, 24x3) */ 00111 #define LCD_T_E 0x00050000 /*Mode E, 40x4 display (actually two 40x2) */ 00112 #define LCD_T_F 0x00060000 /*Mode F, 16x3 display (actually 24x2) */ 00113 #define LCD_T_G 0x00070000 /*Mode G, 16x3 display */ 00114 00115 /* LCD Ctrl information on interface support and features. This information is encoded in 00116 * an int and used for the LCDCtrl enumerators in order to simplify code maintenance */ 00117 // Interface encoded in b31..b24 00118 #define LCD_C_BUS_MSK 0xFF000000 00119 #define LCD_C_PAR 0x01000000 /*Parallel 4 or 8 bit data, E pin, RS pin, RW=GND */ 00120 #define LCD_C_SPI3_8 0x02000000 /*SPI 3 line (MOSI, SCL, CS pins), 8 bits (Count Command initiates Data transfer) */ 00121 #define LCD_C_SPI3_9 0x04000000 /*SPI 3 line (MOSI, SCL, CS pins), 9 bits (RS + 8 Data) */ 00122 #define LCD_C_SPI3_10 0x08000000 /*SPI 3 line (MOSI, SCL, CS pins), 10 bits (RS, RW + 8 Data) */ 00123 #define LCD_C_SPI3_16 0x10000000 /*SPI 3 line (MOSI, SCL, CS pins), 16 bits (RS, RW + 8 Data) */ 00124 #define LCD_C_SPI3_24 0x20000000 /*SPI 3 line (MOSI, SCL, CS pins), 24 bits (RS, RW + 8 Data) */ 00125 #define LCD_C_SPI4 0x40000000 /*SPI 4 line (MOSI, SCL, CS, RS pin), RS pin + 8 Data */ 00126 #define LCD_C_I2C 0x80000000 /*I2C (SDA, SCL pin), 8 control bits (Co, RS, RW) + 8 Data */ 00127 // Features encoded in b23..b16 00128 #define LCD_C_FTR_MSK 0x00FF0000 00129 #define LCD_C_BST 0x00010000 /*Booster */ 00130 #define LCD_C_CTR 0x00020000 /*Contrast Control */ 00131 #define LCD_C_ICN 0x00040000 /*Icons */ 00132 #define LCD_C_PDN 0x00080000 /*Power Down */ 00133 00134 /** A TextLCD interface for driving 4-bit HD44780-based LCDs 00135 * 00136 * @brief Currently supports 8x1, 8x2, 12x2, 12x3, 12x4, 16x1, 16x2, 16x3, 16x4, 20x2, 20x4, 24x2, 24x4, 40x2 and 40x4 panels 00137 * Interface options include direct mbed pins, I2C portexpander (PCF8474/PCF8574A or MCP23008) or SPI bus shiftregister (74595) and some native I2C or SPI devices 00138 * 00139 */ 00140 class TextLCD_Base : public Stream { 00141 //class TextLCD_Base{ 00142 00143 //Unfortunately this #define selection breaks Doxygen !!! 00144 //#if (LCD_PRINTF == 1) 00145 //class TextLCD_Base : public Stream { 00146 //#else 00147 //class TextLCD_Base{ 00148 //#endif 00149 00150 public: 00151 00152 /** LCD panel format */ 00153 // The commented out types exist but have not yet been tested with the library 00154 enum LCDType { 00155 // LCD6x1 = (LCD_T_A | LCD_T_C6 | LCD_T_R1), /**< 6x1 LCD panel */ 00156 // LCD6x2 = (LCD_T_A | LCD_T_C6 | LCD_T_R2), /**< 6x2 LCD panel */ 00157 LCD8x1 = (LCD_T_A | LCD_T_C8 | LCD_T_R1), /**< 8x1 LCD panel */ 00158 LCD8x2 = (LCD_T_A | LCD_T_C8 | LCD_T_R2), /**< 8x2 LCD panel */ 00159 LCD8x2B = (LCD_T_D | LCD_T_C8 | LCD_T_R2), /**< 8x2 LCD panel (actually 16x1) */ 00160 LCD12x1 = (LCD_T_A | LCD_T_C12 | LCD_T_R1), /**< 12x1 LCD panel */ 00161 LCD12x2 = (LCD_T_A | LCD_T_C12 | LCD_T_R2), /**< 12x2 LCD panel */ 00162 LCD12x3D = (LCD_T_D | LCD_T_C12 | LCD_T_R3), /**< 12x3 LCD panel, special mode PCF21XX, KS0073 */ 00163 LCD12x3D1 = (LCD_T_D1 | LCD_T_C12 | LCD_T_R3), /**< 12x3 LCD panel, special mode PCF21XX, KS0073 */ 00164 // LCD12x3G = (LCD_T_G | LCD_T_C12 | LCD_T_R3), /**< 12x3 LCD panel, special mode ST7036 */ 00165 LCD12x4 = (LCD_T_A | LCD_T_C12 | LCD_T_R4), /**< 12x4 LCD panel */ 00166 LCD12x4D = (LCD_T_B | LCD_T_C12 | LCD_T_R4), /**< 12x4 LCD panel, special mode PCF21XX, KS0073 */ 00167 LCD16x1 = (LCD_T_A | LCD_T_C16 | LCD_T_R1), /**< 16x1 LCD panel */ 00168 LCD16x1C = (LCD_T_C | LCD_T_C16 | LCD_T_R1), /**< 16x1 LCD panel (actually 8x2) */ 00169 LCD16x2 = (LCD_T_A | LCD_T_C16 | LCD_T_R2), /**< 16x2 LCD panel (default) */ 00170 // LCD16x2B = (LCD_T_B | LCD_T_C16 | LCD_T_R2), /**< 16x2 LCD panel, alternate addressing, wrong.. */ 00171 LCD16x3D = (LCD_T_D | LCD_T_C16 | LCD_T_R3), /**< 16x3 LCD panel, special mode SSD1803 */ 00172 // LCD16x3D1 = (LCD_T_D1 | LCD_T_C16 | LCD_T_R3), /**< 16x3 LCD panel, special mode SSD1803 */ 00173 LCD16x3F = (LCD_T_F | LCD_T_C16 | LCD_T_R3), /**< 16x3 LCD panel (actually 24x2) */ 00174 LCD16x3G = (LCD_T_G | LCD_T_C16 | LCD_T_R3), /**< 16x3 LCD panel, special mode ST7036 */ 00175 LCD16x4 = (LCD_T_A | LCD_T_C16 | LCD_T_R4), /**< 16x4 LCD panel */ 00176 // LCD16x4D = (LCD_T_D | LCD_T_C16 | LCD_T_R4), /**< 16x4 LCD panel, special mode SSD1803 */ 00177 LCD20x1 = (LCD_T_A | LCD_T_C20 | LCD_T_R1), /**< 20x1 LCD panel */ 00178 LCD20x2 = (LCD_T_A | LCD_T_C20 | LCD_T_R2), /**< 20x2 LCD panel */ 00179 // LCD20x3 = (LCD_T_A | LCD_T_C20 | LCD_T_R3), /**< 20x3 LCD panel */ 00180 // LCD20x3D = (LCD_T_D | LCD_T_C20 | LCD_T_R3), /**< 20x3 LCD panel, special mode SSD1803 */ 00181 // LCD20x3D1 = (LCD_T_D1 | LCD_T_C20 | LCD_T_R3), /**< 20x3 LCD panel, special mode SSD1803 */ 00182 LCD20x4 = (LCD_T_A | LCD_T_C20 | LCD_T_R4), /**< 20x4 LCD panel */ 00183 LCD20x4D = (LCD_T_D | LCD_T_C20 | LCD_T_R4), /**< 20x4 LCD panel, special mode SSD1803 */ 00184 LCD24x1 = (LCD_T_A | LCD_T_C24 | LCD_T_R1), /**< 24x1 LCD panel */ 00185 LCD24x2 = (LCD_T_A | LCD_T_C24 | LCD_T_R2), /**< 24x2 LCD panel */ 00186 // LCD24x3D = (LCD_T_D | LCD_T_C24 | LCD_T_R3), /**< 24x3 LCD panel */ 00187 // LCD24x3D1 = (LCD_T_D | LCD_T_C24 | LCD_T_R3), /**< 24x3 LCD panel */ 00188 LCD24x4D = (LCD_T_D | LCD_T_C24 | LCD_T_R4), /**< 24x4 LCD panel, special mode KS0078 */ 00189 // LCD32x1 = (LCD_T_A | LCD_T_C32 | LCD_T_R1), /**< 32x1 LCD panel */ 00190 // LCD32x1C = (LCD_T_C | LCD_T_C32 | LCD_T_R1), /**< 32x1 LCD panel (actually 16x2) */ 00191 // LCD32x2 = (LCD_T_A | LCD_T_C32 | LCD_T_R2), /**< 32x2 LCD panel */ 00192 // LCD32x4 = (LCD_T_A | LCD_T_C32 | LCD_T_R4), /**< 32x4 LCD panel */ 00193 // LCD40x1 = (LCD_T_A | LCD_T_C40 | LCD_T_R1), /**< 40x1 LCD panel */ 00194 // LCD40x1C = (LCD_T_C | LCD_T_C40 | LCD_T_R1), /**< 40x1 LCD panel (actually 20x2) */ 00195 LCD40x2 = (LCD_T_A | LCD_T_C40 | LCD_T_R2), /**< 40x2 LCD panel */ 00196 LCD40x4 = (LCD_T_E | LCD_T_C40 | LCD_T_R4) /**< 40x4 LCD panel, Two controller version */ 00197 }; 00198 00199 00200 /** LCD Controller Device */ 00201 enum LCDCtrl { 00202 HD44780 = 0 | LCD_C_PAR, /**< HD44780 or full equivalent (default) */ 00203 WS0010 = 1 | (LCD_C_PAR | LCD_C_SPI3_10 | LCD_C_PDN), /**< WS0010/RS0010 OLED Controller, 4/8 bit, SPI3 */ 00204 ST7036_3V3 = 2 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< ST7036 3V3 with Booster, 4/8 bit, SPI4, I2C */ 00205 ST7036_5V = 3 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< ST7036 5V no Booster, 4/8 bit, SPI4, I2C */ 00206 ST7032_3V3 = 4 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< ST7032 3V3 with Booster, 4/8 bit, SPI4, I2C */ 00207 ST7032_5V = 5 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_CTR), /**< ST7032 5V no Booster, 4/8 bit, SPI4, I2C */ 00208 KS0078 = 6 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_PDN), /**< KS0078 24x4 support, 4/8 bit, SPI3 */ 00209 PCF2103_3V3 = 7 | (LCD_C_PAR | LCD_C_I2C), /**< PCF2103 3V3 no Booster, 4/8 bit, I2C */ 00210 PCF2113_3V3 = 8 | (LCD_C_PAR | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< PCF2113 3V3 with Booster, 4/8 bit, I2C */ 00211 PCF2116_3V3 = 9 | (LCD_C_PAR | LCD_C_I2C | LCD_C_BST), /**< PCF2116 3V3 with Booster, 4/8 bit, I2C */ 00212 PCF2116_5V = 10 | (LCD_C_PAR | LCD_C_I2C), /**< PCF2116 5V no Booster, 4/8 bit, I2C */ 00213 PCF2119_3V3 = 11 | (LCD_C_PAR | LCD_C_I2C | LCD_C_BST | LCD_C_CTR), /**< PCF2119 3V3 with Booster, 4/8 bit, I2C */ 00214 // PCF2119_5V = 12 | (LCD_C_PAR | LCD_C_I2C), /**< PCF2119 5V no Booster, 4/8 bit, I2C */ 00215 AIP31068 = 13 | (LCD_C_SPI3_9 | LCD_C_I2C | LCD_C_BST), /**< AIP31068 I2C, SPI3 */ 00216 SSD1803_3V3 = 14 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR | LCD_C_PDN), /**< SSD1803 3V3 with Booster, 4/8 bit, I2C, SPI3 */ 00217 // SSD1803_5V = 15 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_I2C | LCD_C_BST | LCD_C_CTR | LCD_C_PDN), /**< SSD1803 3V3 with Booster, 4/8 bit, I2C, SPI3 */ 00218 US2066_3V3 = 16 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_I2C | LCD_C_CTR | LCD_C_PDN), /**< US2066/SSD1311 3V3, 4/8 bit, I2C, SPI3 */ 00219 PT6314 = 17 | (LCD_C_PAR | LCD_C_SPI3_16 | LCD_C_CTR), /**< PT6314 VFD, 4/8 bit, SPI3 */ 00220 AC780 = 18 | (LCD_C_PAR | LCD_C_SPI4 | LCD_C_I2C | LCD_C_PDN), /**< AC780/KS0066i 4/8 bit, SPI, I2C */ 00221 // WS0012 = 19 | (LCD_C_PAR | LCD_C_SPI3_10 | LCD_C_I2C | LCD_C_PDN), /**< WS0012 4/8 bit, SPI, I2C */ 00222 ST7070 = 20 | (LCD_C_PAR | LCD_C_SPI3_8 | LCD_C_SPI4), /**< ST7070 4/8 bit, SPI3 */ 00223 KS0073 = 21 | (LCD_C_PAR | LCD_C_SPI3_24 | LCD_C_PDN) /**< KS0073 4/8 bit, SPI3 */ 00224 }; 00225 00226 00227 /** LCD Cursor control */ 00228 enum LCDCursor { 00229 CurOff_BlkOff = 0x00, /**< Cursor Off, Blinking Char Off */ 00230 CurOn_BlkOff = 0x02, /**< Cursor On, Blinking Char Off */ 00231 CurOff_BlkOn = 0x01, /**< Cursor Off, Blinking Char On */ 00232 CurOn_BlkOn = 0x03 /**< Cursor On, Blinking Char On */ 00233 }; 00234 00235 /** LCD Display control */ 00236 enum LCDMode { 00237 DispOff = 0x00, /**< Display Off */ 00238 DispOn = 0x04 /**< Display On */ 00239 }; 00240 00241 /** LCD Backlight control */ 00242 enum LCDBacklight { 00243 LightOff, /**< Backlight Off */ 00244 LightOn /**< Backlight On */ 00245 }; 00246 00247 /** LCD Blink control (UDC), supported for some Controllers */ 00248 enum LCDBlink { 00249 BlinkOff, /**< Blink Off */ 00250 BlinkOn /**< Blink On */ 00251 }; 00252 00253 /** LCD Orientation control, supported for some Controllers */ 00254 enum LCDOrient { 00255 Top, /**< Top view */ 00256 Bottom /**< Upside down view */ 00257 }; 00258 00259 /** LCD BigFont control, supported for some Controllers */ 00260 enum LCDBigFont { 00261 None, /**< no lines */ 00262 TopLine, /**< 1+2 line */ 00263 CenterLine, /**< 2+3 line */ 00264 BottomLine, /**< 2+3 line or 3+4 line */ 00265 TopBottomLine /**< 1+2 line and 3+4 line */ 00266 }; 00267 00268 00269 #if(LCD_PRINTF != 1) 00270 /** Write a character to the LCD 00271 * 00272 * @param c The character to write to the display 00273 */ 00274 int putc(int c); 00275 00276 /** Write a raw string to the LCD 00277 * 00278 * @param string text, may be followed by variables to emulate formatting the string. 00279 * However, printf formatting is NOT supported and variables will be ignored! 00280 */ 00281 int printf(const char* text, ...); 00282 #else 00283 #if DOXYGEN_ONLY 00284 /** Write a character to the LCD 00285 * 00286 * @param c The character to write to the display 00287 */ 00288 int putc(int c); 00289 00290 /** Write a formatted string to the LCD 00291 * 00292 * @param format A printf-style format string, followed by the 00293 * variables to use in formatting the string. 00294 */ 00295 int printf(const char* format, ...); 00296 #endif 00297 00298 #endif 00299 00300 /** Locate cursor to a screen column and row 00301 * 00302 * @param column The horizontal position from the left, indexed from 0 00303 * @param row The vertical position from the top, indexed from 0 00304 */ 00305 void locate(int column, int row); 00306 00307 /** Return the memoryaddress of screen column and row location 00308 * 00309 * @param column The horizontal position from the left, indexed from 0 00310 * @param row The vertical position from the top, indexed from 0 00311 * @return The memoryaddress of screen column and row location 00312 */ 00313 int getAddress(int column, int row); 00314 00315 /** Set the memoryaddress of screen column and row location 00316 * 00317 * @param column The horizontal position from the left, indexed from 0 00318 * @param row The vertical position from the top, indexed from 0 00319 */ 00320 void setAddress(int column, int row); 00321 00322 /** Clear the screen and locate to 0,0 00323 */ 00324 void cls(); 00325 00326 /** Return the number of rows 00327 * 00328 * @return The number of rows 00329 */ 00330 int rows(); 00331 00332 /** Return the number of columns 00333 * 00334 * @return The number of columns 00335 */ 00336 int columns(); 00337 00338 /** Set the Cursormode 00339 * 00340 * @param cursorMode The Cursor mode (CurOff_BlkOff, CurOn_BlkOff, CurOff_BlkOn, CurOn_BlkOn) 00341 */ 00342 void setCursor(LCDCursor cursorMode); 00343 00344 /** Set the Displaymode 00345 * 00346 * @param displayMode The Display mode (DispOff, DispOn) 00347 */ 00348 void setMode(LCDMode displayMode); 00349 00350 /** Set the Backlight mode 00351 * 00352 * @param backlightMode The Backlight mode (LightOff, LightOn) 00353 */ 00354 void setBacklight(LCDBacklight backlightMode); 00355 00356 /** Set User Defined Characters (UDC) 00357 * 00358 * @param unsigned char c The Index of the UDC (0..7) for HD44780 clones and (0..15) for some more advanced controllers 00359 * @param char *udc_data The bitpatterns for the UDC (8 bytes of 5 significant bits for bitpattern and 3 bits for blinkmode (advanced types)) 00360 */ 00361 void setUDC(unsigned char c, char *udc_data); 00362 00363 /** Set UDC Blink and Icon blink 00364 * setUDCBlink method is supported by some compatible devices (eg SSD1803) 00365 * 00366 * @param blinkMode The Blink mode (BlinkOff, BlinkOn) 00367 */ 00368 void setUDCBlink(LCDBlink blinkMode); 00369 00370 /** Set Contrast 00371 * setContrast method is supported by some compatible devices (eg ST7032i) that have onboard LCD voltage generation 00372 * Code imported from fork by JH1PJL 00373 * 00374 * @param unsigned char c contrast data (6 significant bits, valid range 0..63, Value 0 will disable the Vgen) 00375 * @return none 00376 */ 00377 void setContrast(unsigned char c = LCD_DEF_CONTRAST); 00378 00379 /** Set Power 00380 * setPower method is supported by some compatible devices (eg SSD1803) that have power down modes 00381 * 00382 * @param bool powerOn Power on/off 00383 * @return none 00384 */ 00385 void setPower(bool powerOn = true); 00386 00387 /** Set Orient 00388 * setOrient method is supported by some compatible devices (eg SSD1803, US2066) that have top/bottom view modes 00389 * 00390 * @param LCDOrient orient Orientation 00391 * @return none 00392 */ 00393 void setOrient(LCDOrient orient = Top); 00394 00395 /** Set Big Font 00396 * setBigFont method is supported by some compatible devices (eg SSD1803, US2066) 00397 * 00398 * @param lines The selected Big Font lines (None, TopLine, CenterLine, BottomLine, TopBottomLine) 00399 * Double height characters can be shown on lines 1+2, 2+3, 3+4 or 1+2 and 3+4 00400 * Valid double height lines depend on the LCDs number of rows. 00401 */ 00402 void setBigFont(LCDBigFont lines); 00403 00404 /** Set Icons 00405 * 00406 * @param unsigned char idx The Index of the icon pattern (0..15) for KS0073 and similar controllers 00407 * and Index (0..31) for PCF2103 and similar controllers 00408 * @param unsigned char data The bitpattern for the icons (6 lsb for KS0073 bitpattern (5 lsb for KS0078) and 2 msb for blinkmode) 00409 * The bitpattern for the PCF2103 icons is 5 lsb (UDC 0..2) and 5 lsb for blinkmode (UDC 4..6) 00410 */ 00411 void setIcon(unsigned char idx, unsigned char data); 00412 00413 /** Clear Icons 00414 * 00415 * @param none 00416 * @return none 00417 */ 00418 //@TODO Add support for 40x4 dual controller 00419 void clrIcon(); 00420 00421 /** Set Invert 00422 * setInvert method is supported by some compatible devices (eg KS0073) to swap between black and white 00423 * 00424 * @param bool invertOn Invert on/off 00425 * @return none 00426 */ 00427 //@TODO Add support for 40x4 dual controller 00428 void setInvert(bool invertOn); 00429 00430 protected: 00431 00432 /** LCD controller select, mainly used for LCD40x4 00433 */ 00434 enum _LCDCtrl_Idx { 00435 _LCDCtrl_0, /*< Primary */ 00436 _LCDCtrl_1, /*< Secondary */ 00437 }; 00438 00439 /** LCD Datalength control to select between 4 or 8 bit data/commands, used for native Serial interface */ 00440 enum _LCDDatalength { 00441 _LCD_DL_4 = 0x00, /**< Datalength 4 bit */ 00442 _LCD_DL_8 = 0x10 /**< Datalength 8 bit */ 00443 }; 00444 00445 /** Create a TextLCD_Base interface 00446 * @brief Base class, can not be instantiated 00447 * 00448 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00449 * @param ctrl LCD controller (default = HD44780) 00450 */ 00451 TextLCD_Base(LCDType type = LCD16x2, LCDCtrl ctrl = HD44780); 00452 00453 // Stream implementation functions 00454 virtual int _putc(int value); 00455 virtual int _getc(); 00456 00457 /** Medium level initialisation method for LCD controller 00458 * @param _LCDDatalength dl sets the datalength of data/commands 00459 * @return none 00460 */ 00461 void _init(_LCDDatalength dl = _LCD_DL_4); 00462 00463 /** Low level initialisation method for LCD controller 00464 * Set number of lines, fonttype, no cursor etc 00465 * The controller is accessed in 4-bit parallel mode either directly via mbed pins or through I2C or SPI expander. 00466 * Some controllers also support native I2C or SPI interfaces. 00467 * 00468 * @param _LCDDatalength dl sets the 4 or 8 bit datalength of data/commands. Required for some native serial modes. 00469 * @return none 00470 */ 00471 void _initCtrl(_LCDDatalength dl = _LCD_DL_4); 00472 00473 /** Low level character address set method 00474 */ 00475 int _address(int column, int row); 00476 00477 /** Low level cursor enable or disable method 00478 */ 00479 void _setCursor(LCDCursor show); 00480 00481 /** Low level method to store user defined characters for current controller 00482 * 00483 * @param unsigned char c The Index of the UDC (0..7) for HD44780 clones and (0..15) for some more advanced controllers 00484 * @param char *udc_data The bitpatterns for the UDC (8 bytes of 5 significant bits) 00485 */ 00486 void _setUDC(unsigned char c, char *udc_data); 00487 00488 /** Low level method to restore the cursortype and display mode for current controller 00489 */ 00490 void _setCursorAndDisplayMode(LCDMode displayMode, LCDCursor cursorType); 00491 00492 /** Low level nibble write operation to LCD controller (serial or parallel) 00493 */ 00494 void _writeNibble(int value); 00495 00496 /** Low level command byte write operation to LCD controller. 00497 * Methods resets the RS bit and provides the required timing for the command. 00498 */ 00499 void _writeCommand(int command); 00500 00501 /** Low level data byte write operation to LCD controller (serial or parallel). 00502 * Methods sets the RS bit and provides the required timing for the data. 00503 */ 00504 void _writeData(int data); 00505 00506 /** Pure Virtual Low level writes to LCD Bus (serial or parallel) 00507 * Set the Enable pin. 00508 */ 00509 virtual void _setEnable(bool value) = 0; 00510 00511 /** Pure Virtual Low level writes to LCD Bus (serial or parallel) 00512 * Set the RS pin ( 0 = Command, 1 = Data). 00513 */ 00514 virtual void _setRS(bool value) = 0; 00515 00516 /** Pure Virtual Low level writes to LCD Bus (serial or parallel) 00517 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00518 */ 00519 virtual void _setBL(bool value) = 0; 00520 00521 /** Pure Virtual Low level writes to LCD Bus (serial or parallel) 00522 * Set the databus value (4 bit). 00523 */ 00524 virtual void _setData(int value) = 0; 00525 00526 /** Low level byte write operation to LCD controller (serial or parallel) 00527 * Depending on the RS pin this byte will be interpreted as data or command 00528 */ 00529 virtual void _writeByte(int value); 00530 00531 //Display type 00532 LCDType _type; 00533 int _nr_cols; 00534 int _nr_rows; 00535 int _addr_mode; 00536 00537 //Display mode 00538 LCDMode _currentMode; 00539 00540 //Controller type 00541 LCDCtrl _ctrl; 00542 00543 //Controller select, mainly used for LCD40x4 00544 _LCDCtrl_Idx _ctrl_idx; 00545 00546 // Cursor 00547 int _column; 00548 int _row; 00549 LCDCursor _currentCursor; 00550 00551 // Function modes saved to allow switch between Instruction sets after initialisation time 00552 int _function, _function_1, _function_x; 00553 00554 // Icon, Booster mode and contrast saved to allow contrast change at later time 00555 // Only available for controllers with added features 00556 int _icon_power, _contrast; 00557 }; 00558 00559 //--------- End TextLCD_Base ----------- 00560 00561 00562 //--------- Start TextLCD Bus ----------- 00563 00564 /** Create a TextLCD interface for using regular mbed pins 00565 * 00566 */ 00567 class TextLCD : public TextLCD_Base { 00568 public: 00569 /** Create a TextLCD interface for using regular mbed pins 00570 * 00571 * @param rs Instruction/data control line 00572 * @param e Enable line (clock) 00573 * @param d4-d7 Data lines for using as a 4-bit interface 00574 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00575 * @param bl Backlight control line (optional, default = NC) 00576 * @param e2 Enable2 line (clock for second controller, LCD40x4 only) 00577 * @param ctrl LCD controller (default = HD44780) 00578 */ 00579 TextLCD(PinName rs, PinName e, PinName d4, PinName d5, PinName d6, PinName d7, LCDType type = LCD16x2, PinName bl = NC, PinName e2 = NC, LCDCtrl ctrl = HD44780); 00580 00581 /** Destruct a TextLCD interface for using regular mbed pins 00582 * 00583 * @param none 00584 * @return none 00585 */ 00586 virtual ~TextLCD(); 00587 00588 private: 00589 00590 /** Implementation of pure Virtual Low level writes to LCD Bus (parallel) 00591 * Set the Enable pin. 00592 */ 00593 virtual void _setEnable(bool value); 00594 00595 /** Implementation of pure Virtual Low level writes to LCD Bus (parallel) 00596 * Set the RS pin (0 = Command, 1 = Data). 00597 */ 00598 virtual void _setRS(bool value); 00599 00600 /** Implementation of pure Virtual Low level writes to LCD Bus (parallel) 00601 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00602 */ 00603 virtual void _setBL(bool value); 00604 00605 /** Implementation of pure Virtual Low level writes to LCD Bus (parallel) 00606 * Set the databus value (4 bit). 00607 */ 00608 virtual void _setData(int value); 00609 00610 00611 /** Regular mbed pins bus 00612 */ 00613 DigitalOut _rs, _e; 00614 BusOut _d; 00615 00616 /** Optional Hardware pins for the Backlight and LCD40x4 device 00617 * Default PinName value is NC, must be used as pointer to avoid issues with mbed lib and DigitalOut pins 00618 */ 00619 DigitalOut *_bl, *_e2; 00620 }; 00621 00622 //----------- End TextLCD --------------- 00623 00624 00625 //--------- Start TextLCD_I2C ----------- 00626 #if(LCD_I2C == 1) /* I2C Expander PCF8574/MCP23008 */ 00627 00628 /** Create a TextLCD interface using an I2C PCF8574 (or PCF8574A) or MCP23008 portexpander 00629 * 00630 */ 00631 class TextLCD_I2C : public TextLCD_Base { 00632 public: 00633 /** Create a TextLCD interface using an I2C PCF8574 (or PCF8574A) or MCP23008 portexpander 00634 * 00635 * @param i2c I2C Bus 00636 * @param deviceAddress I2C slave address (PCF8574 or PCF8574A, default = PCF8574_SA0 = 0x40) 00637 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00638 * @param ctrl LCD controller (default = HD44780) 00639 */ 00640 TextLCD_I2C(I2C *i2c, char deviceAddress = PCF8574_SA0, LCDType type = LCD16x2, LCDCtrl ctrl = HD44780); 00641 00642 private: 00643 00644 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00645 * Set the Enable pin. 00646 */ 00647 virtual void _setEnable(bool value); 00648 00649 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00650 * Set the RS pin (0 = Command, 1 = Data). 00651 */ 00652 virtual void _setRS(bool value); 00653 00654 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00655 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00656 */ 00657 virtual void _setBL(bool value); 00658 00659 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00660 * Set the databus value (4 bit). 00661 */ 00662 virtual void _setData(int value); 00663 00664 /** Write data to MCP23008 I2C portexpander 00665 * @param reg register to write 00666 * @param value data to write 00667 * @return none 00668 * 00669 */ 00670 void _write_register (int reg, int value); 00671 00672 //I2C bus 00673 I2C *_i2c; 00674 char _slaveAddress; 00675 00676 // Internal bus mirror value for serial bus only 00677 char _lcd_bus; 00678 }; 00679 #endif /* I2C Expander PCF8574/MCP23008 */ 00680 00681 //---------- End TextLCD_I2C ------------ 00682 00683 00684 //--------- Start TextLCD_SPI ----------- 00685 #if(LCD_SPI == 1) /* SPI Expander SN74595 */ 00686 00687 /** Create a TextLCD interface using an SPI 74595 portexpander 00688 * 00689 */ 00690 class TextLCD_SPI : public TextLCD_Base { 00691 public: 00692 /** Create a TextLCD interface using an SPI 74595 portexpander 00693 * 00694 * @param spi SPI Bus 00695 * @param cs chip select pin (active low) 00696 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00697 * @param ctrl LCD controller (default = HD44780) 00698 */ 00699 TextLCD_SPI(SPI *spi, PinName cs, LCDType type = LCD16x2, LCDCtrl ctrl = HD44780); 00700 00701 private: 00702 00703 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00704 * Set the Enable pin. 00705 */ 00706 virtual void _setEnable(bool value); 00707 00708 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00709 * Set the RS pin (0 = Command, 1 = Data). 00710 */ 00711 virtual void _setRS(bool value); 00712 00713 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00714 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00715 */ 00716 virtual void _setBL(bool value); 00717 00718 /** Implementation of pure Virtual Low level writes to LCD Bus (serial expander) 00719 * Set the databus value (4 bit). 00720 */ 00721 virtual void _setData(int value); 00722 00723 /** Implementation of Low level writes to LCD Bus (serial expander) 00724 * Set the CS pin (0 = select, 1 = deselect). 00725 */ 00726 virtual void _setCS(bool value); 00727 00728 ///** Low level writes to LCD serial bus only (serial expander) 00729 // */ 00730 // void _writeBus(); 00731 00732 // SPI bus 00733 SPI *_spi; 00734 DigitalOut _cs; 00735 00736 // Internal bus mirror value for serial bus only 00737 char _lcd_bus; 00738 }; 00739 #endif /* SPI Expander SN74595 */ 00740 //---------- End TextLCD_SPI ------------ 00741 00742 00743 //--------- Start TextLCD_SPI_N ----------- 00744 #if(LCD_SPI_N == 1) /* Native SPI bus */ 00745 00746 /** Create a TextLCD interface using a controller with native SPI4 interface 00747 * 00748 */ 00749 class TextLCD_SPI_N : public TextLCD_Base { 00750 public: 00751 /** Create a TextLCD interface using a controller with native SPI4 interface 00752 * 00753 * @param spi SPI Bus 00754 * @param cs chip select pin (active low) 00755 * @param rs Instruction/data control line 00756 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00757 * @param bl Backlight control line (optional, default = NC) 00758 * @param ctrl LCD controller (default = ST7032_3V3) 00759 */ 00760 TextLCD_SPI_N(SPI *spi, PinName cs, PinName rs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = ST7032_3V3); 00761 virtual ~TextLCD_SPI_N(void); 00762 00763 private: 00764 00765 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00766 * Set the Enable pin. 00767 */ 00768 virtual void _setEnable(bool value); 00769 00770 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00771 * Set the RS pin (0 = Command, 1 = Data). 00772 */ 00773 virtual void _setRS(bool value); 00774 00775 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00776 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00777 */ 00778 virtual void _setBL(bool value); 00779 00780 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00781 * Set the databus value (4 bit). 00782 */ 00783 virtual void _setData(int value); 00784 00785 /** Low level writes to LCD serial bus only (serial native) 00786 */ 00787 virtual void _writeByte(int value); 00788 00789 // SPI bus 00790 SPI *_spi; 00791 DigitalOut _cs; 00792 DigitalOut _rs; 00793 00794 //Backlight 00795 DigitalOut *_bl; 00796 }; 00797 #endif /* Native SPI bus */ 00798 //---------- End TextLCD_SPI_N ------------ 00799 00800 00801 //-------- Start TextLCD_SPI_N_3_8 -------- 00802 #if(LCD_SPI_N_3_8 == 1) /* Native SPI bus */ 00803 00804 class TextLCD_SPI_N_3_8 : public TextLCD_Base { 00805 public: 00806 /** Create a TextLCD interface using a controller with a native SPI3 8 bits interface 00807 * This mode is supported by ST7070. Note that implementation in TexTLCD is not very efficient due to 00808 * structure of the TextLCD library: each databyte is written separately and requires a separate 'count command' set to 1 byte. 00809 * 00810 * @param spi SPI Bus 00811 * @param cs chip select pin (active low) 00812 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00813 * @param bl Backlight control line (optional, default = NC) 00814 * @param ctrl LCD controller (default = ST7070) 00815 */ 00816 TextLCD_SPI_N_3_8(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = ST7070); 00817 00818 virtual ~TextLCD_SPI_N_3_8(void); 00819 00820 private: 00821 00822 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00823 * Set the Enable pin. 00824 */ 00825 virtual void _setEnable(bool value); 00826 00827 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00828 * Set the RS pin (0 = Command, 1 = Data). 00829 */ 00830 virtual void _setRS(bool value); 00831 00832 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00833 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00834 */ 00835 virtual void _setBL(bool value); 00836 00837 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00838 * Set the databus value (4 bit). 00839 */ 00840 virtual void _setData(int value); 00841 00842 /** Low level writes to LCD serial bus only (serial native) 00843 */ 00844 virtual void _writeByte(int value); 00845 00846 // SPI bus 00847 SPI *_spi; 00848 DigitalOut _cs; 00849 00850 // controlbyte to select between data and command. Internal value for serial bus only 00851 char _controlbyte; 00852 00853 //Backlight 00854 DigitalOut *_bl; 00855 }; 00856 00857 #endif /* Native SPI bus */ 00858 //------- End TextLCD_SPI_N_3_8 ----------- 00859 00860 00861 00862 //------- Start TextLCD_SPI_N_3_9 --------- 00863 #if(LCD_SPI_N_3_9 == 1) /* Native SPI bus */ 00864 //Code checked out on logic analyser. Not yet tested on hardware.. 00865 00866 /** Create a TextLCD interface using a controller with native SPI3 9 bits interface 00867 * Note: current mbed libs only support SPI 9 bit mode for NXP platforms 00868 * 00869 */ 00870 class TextLCD_SPI_N_3_9 : public TextLCD_Base { 00871 public: 00872 /** Create a TextLCD interface using a controller with native SPI3 9 bits interface 00873 * Note: current mbed libs only support SPI 9 bit mode for NXP platforms 00874 * 00875 * @param spi SPI Bus 00876 * @param cs chip select pin (active low) 00877 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00878 * @param bl Backlight control line (optional, default = NC) 00879 * @param ctrl LCD controller (default = AIP31068) 00880 */ 00881 TextLCD_SPI_N_3_9(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = AIP31068); 00882 virtual ~TextLCD_SPI_N_3_9(void); 00883 00884 private: 00885 00886 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00887 * Set the Enable pin. 00888 */ 00889 virtual void _setEnable(bool value); 00890 00891 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00892 * Set the RS pin (0 = Command, 1 = Data). 00893 */ 00894 virtual void _setRS(bool value); 00895 00896 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00897 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00898 */ 00899 virtual void _setBL(bool value); 00900 00901 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00902 * Set the databus value (4 bit). 00903 */ 00904 virtual void _setData(int value); 00905 00906 /** Low level writes to LCD serial bus only (serial native) 00907 */ 00908 virtual void _writeByte(int value); 00909 00910 // SPI bus 00911 SPI *_spi; 00912 DigitalOut _cs; 00913 00914 // controlbyte to select between data and command. Internal value for serial bus only 00915 char _controlbyte; 00916 00917 //Backlight 00918 DigitalOut *_bl; 00919 }; 00920 #endif /* Native SPI bus */ 00921 //-------- End TextLCD_SPI_N_3_9 ---------- 00922 00923 00924 //------- Start TextLCD_SPI_N_3_10 --------- 00925 #if(LCD_SPI_N_3_10 == 1) /* Native SPI bus */ 00926 00927 /** Create a TextLCD interface using a controller with native SPI3 10 bits interface 00928 * Note: current mbed libs only support SPI 10 bit mode for NXP platforms 00929 * 00930 */ 00931 class TextLCD_SPI_N_3_10 : public TextLCD_Base { 00932 public: 00933 /** Create a TextLCD interface using a controller with native SPI3 10 bits interface 00934 * Note: current mbed libs only support SPI 10 bit mode for NXP platforms 00935 * 00936 * @param spi SPI Bus 00937 * @param cs chip select pin (active low) 00938 * @param type Sets the panel size/addressing mode (default = LCD16x2) 00939 * @param bl Backlight control line (optional, default = NC) 00940 * @param ctrl LCD controller (default = AIP31068) 00941 */ 00942 TextLCD_SPI_N_3_10(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = AIP31068); 00943 virtual ~TextLCD_SPI_N_3_10(void); 00944 00945 private: 00946 00947 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00948 * Set the Enable pin. 00949 */ 00950 virtual void _setEnable(bool value); 00951 00952 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00953 * Set the RS pin (0 = Command, 1 = Data). 00954 */ 00955 virtual void _setRS(bool value); 00956 00957 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00958 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 00959 */ 00960 virtual void _setBL(bool value); 00961 00962 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 00963 * Set the databus value (4 bit). 00964 */ 00965 virtual void _setData(int value); 00966 00967 /** Low level writes to LCD serial bus only (serial native) 00968 */ 00969 virtual void _writeByte(int value); 00970 00971 // SPI bus 00972 SPI *_spi; 00973 DigitalOut _cs; 00974 00975 // controlbyte to select between data and command. Internal value for serial bus only 00976 char _controlbyte; 00977 00978 //Backlight 00979 DigitalOut *_bl; 00980 }; 00981 00982 #endif /* Native SPI bus */ 00983 //-------- End TextLCD_SPI_N_3_10 ---------- 00984 00985 00986 //------- Start TextLCD_SPI_N_3_16 --------- 00987 #if(LCD_SPI_N_3_16 == 1) /* Native SPI bus */ 00988 //Code checked out on logic analyser. Not yet tested on hardware.. 00989 00990 /** Create a TextLCD interface using a controller with native SPI3 16 bits interface 00991 * 00992 */ 00993 class TextLCD_SPI_N_3_16 : public TextLCD_Base { 00994 public: 00995 /** Create a TextLCD interface using a controller with native SPI3 16 bits interface 00996 * 00997 * @param spi SPI Bus 00998 * @param cs chip select pin (active low) 00999 * @param type Sets the panel size/addressing mode (default = LCD16x2) 01000 * @param bl Backlight control line (optional, default = NC) 01001 * @param ctrl LCD controller (default = PT6314) 01002 */ 01003 TextLCD_SPI_N_3_16(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = PT6314); 01004 virtual ~TextLCD_SPI_N_3_16(void); 01005 01006 private: 01007 01008 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01009 * Set the Enable pin. 01010 */ 01011 virtual void _setEnable(bool value); 01012 01013 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01014 * Set the RS pin (0 = Command, 1 = Data). 01015 */ 01016 virtual void _setRS(bool value); 01017 01018 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01019 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 01020 */ 01021 virtual void _setBL(bool value); 01022 01023 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01024 * Set the databus value (4 bit). 01025 */ 01026 virtual void _setData(int value); 01027 01028 /** Low level writes to LCD serial bus only (serial native) 01029 */ 01030 virtual void _writeByte(int value); 01031 01032 // SPI bus 01033 SPI *_spi; 01034 DigitalOut _cs; 01035 01036 // controlbyte to select between data and command. Internal value for serial bus only 01037 char _controlbyte; 01038 01039 //Backlight 01040 DigitalOut *_bl; 01041 }; 01042 #endif /* Native SPI bus */ 01043 //-------- End TextLCD_SPI_N_3_16 ---------- 01044 01045 01046 //------- Start TextLCD_SPI_N_3_24 --------- 01047 #if(LCD_SPI_N_3_24 == 1) /* Native SPI bus */ 01048 01049 /** Create a TextLCD interface using a controller with native SPI3 24 bits interface 01050 * Note: lib uses SPI 8 bit mode 01051 * 01052 */ 01053 class TextLCD_SPI_N_3_24 : public TextLCD_Base { 01054 public: 01055 /** Create a TextLCD interface using a controller with native SPI3 24 bits interface 01056 * Note: lib uses SPI 8 bit mode 01057 * 01058 * @param spi SPI Bus 01059 * @param cs chip select pin (active low) 01060 * @param type Sets the panel size/addressing mode (default = LCD16x2) 01061 * @param bl Backlight control line (optional, default = NC) 01062 * @param ctrl LCD controller (default = SSD1803) 01063 */ 01064 TextLCD_SPI_N_3_24(SPI *spi, PinName cs, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = SSD1803_3V3); 01065 virtual ~TextLCD_SPI_N_3_24(void); 01066 01067 private: 01068 01069 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01070 * Set the Enable pin. 01071 */ 01072 virtual void _setEnable(bool value); 01073 01074 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01075 * Set the RS pin (0 = Command, 1 = Data). 01076 */ 01077 virtual void _setRS(bool value); 01078 01079 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01080 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 01081 */ 01082 virtual void _setBL(bool value); 01083 01084 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01085 * Set the databus value (4 bit). 01086 */ 01087 virtual void _setData(int value); 01088 01089 /** Low level writes to LCD serial bus only (serial native) 01090 */ 01091 virtual void _writeByte(int value); 01092 01093 // SPI bus 01094 SPI *_spi; 01095 DigitalOut _cs; 01096 01097 // controlbyte to select between data and command. Internal value for serial bus only 01098 char _controlbyte; 01099 01100 //Backlight 01101 DigitalOut *_bl; 01102 }; 01103 #endif /* Native SPI bus */ 01104 //-------- End TextLCD_SPI_N_3_24 ---------- 01105 01106 01107 //--------- Start TextLCD_I2C_N ----------- 01108 #if(LCD_I2C_N == 1) /* Native I2C */ 01109 01110 /** Create a TextLCD interface using a controller with native I2C interface 01111 * 01112 */ 01113 class TextLCD_I2C_N : public TextLCD_Base { 01114 public: 01115 /** Create a TextLCD interface using a controller with native I2C interface 01116 * 01117 * @param i2c I2C Bus 01118 * @param deviceAddress I2C slave address (default = ST7032_SA = 0x7C) 01119 * @param type Sets the panel size/addressing mode (default = LCD16x2) 01120 * @param bl Backlight control line (optional, default = NC) 01121 * @param ctrl LCD controller (default = ST7032_3V3) 01122 */ 01123 TextLCD_I2C_N(I2C *i2c, char deviceAddress = ST7032_SA, LCDType type = LCD16x2, PinName bl = NC, LCDCtrl ctrl = ST7032_3V3); 01124 virtual ~TextLCD_I2C_N(void); 01125 01126 private: 01127 01128 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01129 * Set the Enable pin. 01130 */ 01131 virtual void _setEnable(bool value); 01132 01133 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01134 * Set the RS pin ( 0 = Command, 1 = Data). 01135 */ 01136 virtual void _setRS(bool value); 01137 01138 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01139 * Set the BL pin (0 = Backlight Off, 1 = Backlight On). 01140 */ 01141 virtual void _setBL(bool value); 01142 01143 /** Implementation of pure Virtual Low level writes to LCD Bus (serial native) 01144 * Set the databus value (4 bit). 01145 */ 01146 virtual void _setData(int value); 01147 01148 /** Low level writes to LCD serial bus only (serial native) 01149 */ 01150 virtual void _writeByte(int value); 01151 01152 //I2C bus 01153 I2C *_i2c; 01154 char _slaveAddress; 01155 01156 // controlbyte to select between data and command. Internal value for serial bus only 01157 char _controlbyte; 01158 01159 //Backlight 01160 DigitalOut *_bl; 01161 01162 }; 01163 #endif /* Native I2C */ 01164 //---------- End TextLCD_I2C_N ------------ 01165 01166 #endif
Generated on Thu Jul 14 2022 07:15:20 by
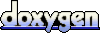