
aquarium light controller wannabe
Dependencies: DS3231 FastPWM Menu QEI TextLCD _24LCXXX mbed
main.cpp
00001 #include "mbed.h" 00002 #include "TextLCD.h" 00003 #include "QEI.h" 00004 #include "Menu.h" 00005 #include "FastPWM.h" 00006 #include "DS3231.h" 00007 #include "_24LCXXX.h" 00008 00009 00010 Serial pc(USBTX, USBRX); //DEBUG 00011 DigitalOut myled(LED1); //DEBUG 00012 00013 // I2C Bus 00014 I2C i2c(PB_4, PA_8); // SDA, SCL 00015 00016 TextLCD_I2C lcd(&i2c, PCF8574_SA7); 00017 _24LCXXX eeprom(&i2c, 0x57); 00018 DS3231 rtc(PB_4, PA_8); 00019 //End I2C BUS 00020 00021 QEI qei(PH_0, PH_1, NC, 80, QEI::X4_ENCODING); //Index is NC(see QEI class), we use PC_13 as digital input 00022 DigitalIn qei_idx(PC_13); 00023 00024 bool qei_pb() //check and debounces qei_idx 00025 { 00026 int check = qei_idx; 00027 wait_us(5); 00028 if((!check) && !qei_idx) { 00029 return true; 00030 } else return false; 00031 } 00032 00033 //PA_11 = ESP SERIAL!!! 00034 FastPWM Pwm_Array[] = {PA_7,PA_5,PA_6,PB_6,PA_9,PB_3,PB_5,PB_7,PA_10,PB_10,PC_8,PB_8,PA_11,PC_9,PB_9}; 00035 00036 //Ticker Pwm_Ticker[sizeof(Pwm_Array)/sizeof(FastPWM)]; 00037 Ticker Sunrise_Ticker; 00038 Ticker Sunset_Ticker; 00039 00040 //uint8_t Pwm_Min_Max_Array[sizeof(Pwm_Array)/sizeof(FastPWM)][1][2] = { 00041 // { {0, 100} }, 00042 // { {20, 80} }, 00043 // { {0, 100} }, 00044 // { {0, 100} }, 00045 // { {0, 100} }, 00046 // { {0, 100} }, 00047 // { {0, 100} }, 00048 // { {0, 100} }, 00049 // { {0, 100} }, 00050 // { {0, 100} }, 00051 // { {0, 100} }, 00052 // { {0, 100} }, 00053 // { {0, 100} }, 00054 // { {0, 100} }, 00055 // { {0, 100} } 00056 //}; 00057 00058 00059 uint8_t Pwm_Min_Max_Array[sizeof(Pwm_Array)/sizeof(FastPWM)][2] = { 00060 {0, 1}, //violet 00061 {1, 35}, //royal blue 00062 {0, 40}, //20000k 00063 {0, 35}, 00064 {0, 35}, 00065 {0, 35}, 00066 {0, 35}, 00067 {0, 35}, 00068 {0, 35}, 00069 {0, 35}, 00070 {0, 35}, 00071 {0, 35}, 00072 {0, 35}, 00073 {0, 35}, 00074 {0, 35} 00075 }; 00076 00077 int pwm_idx = 0; 00078 00079 uint8_t SunRiseSet[2][2] = {{8,0},{20,30}}; 00080 00081 uint8_t ramptime = 30; //in minutes 00082 00083 bool refresh_display = true; 00084 00085 enum { IDLE, 00086 MAIN, 00087 SETTINGS, 00088 PWM_MIN, 00089 PWM_MAX, 00090 SUNRISE, 00091 SUNSET, 00092 RAMPTIME, 00093 SUNRISE_HOUR, 00094 SUNRISE_MINUTE, 00095 SUNSET_HOUR, 00096 SUNSET_MINUTE, 00097 SET_DATE_TIME, 00098 SET_DATE_DAY, 00099 SET_DATE_MONTH, 00100 SET_DATE_YEAR, 00101 SET_TIME_HOUR, 00102 SET_TIME_MINUTE, 00103 SET_TIME_SECOND 00104 } menu_state = MAIN ; // Initial state = MAIN ; 00105 00106 int cursorPos = 0; 00107 Menu *activeMenu; 00108 00109 //struct tm *t; //read time 00110 struct tm t; //mktime 00111 00112 Ticker qei_t; //ticker for qei_cb() 00113 00114 void qei_cb() 00115 { 00116 if(qei.getCurrentState() == 3 && qei.getPulses() < 0) { //one tab left 00117 refresh_display = true; 00118 switch (menu_state) { 00119 case SETTINGS: 00120 cursorPos--; 00121 if (cursorPos < 0) 00122 cursorPos = activeMenu->selections.size()-1; 00123 break; 00124 case PWM_MIN: 00125 if (Pwm_Min_Max_Array[cursorPos][0] > 0) 00126 Pwm_Min_Max_Array[cursorPos][0]--; 00127 break; 00128 case PWM_MAX: 00129 if (Pwm_Min_Max_Array[cursorPos][1] > 0) 00130 Pwm_Min_Max_Array[cursorPos][1]--; 00131 break; 00132 case RAMPTIME: 00133 ramptime--; 00134 break; 00135 case SUNRISE_HOUR: 00136 SunRiseSet[0][0]--; 00137 break; 00138 case SUNRISE_MINUTE: 00139 SunRiseSet[0][1]--; 00140 break; 00141 case SUNSET_HOUR: 00142 SunRiseSet[1][0]--; 00143 break; 00144 case SUNSET_MINUTE: 00145 SunRiseSet[1][1]--; 00146 break; 00147 } 00148 qei.reset(); 00149 } else if(qei.getCurrentState() == 3 && qei.getPulses() > 0) { //one tab right 00150 refresh_display = true; 00151 switch (menu_state) { 00152 case SETTINGS: 00153 cursorPos++; 00154 if (cursorPos >= activeMenu->selections.size()) 00155 cursorPos = 0; 00156 break; 00157 case PWM_MIN: 00158 if (Pwm_Min_Max_Array[cursorPos][0] < 100) 00159 Pwm_Min_Max_Array[cursorPos][0]++; 00160 break; 00161 case PWM_MAX: 00162 if (Pwm_Min_Max_Array[cursorPos][1] < 100) 00163 Pwm_Min_Max_Array[cursorPos][1]++; 00164 break; 00165 case RAMPTIME: 00166 ramptime++; 00167 break; 00168 case SUNRISE_HOUR: 00169 SunRiseSet[0][0]++; 00170 break; 00171 case SUNRISE_MINUTE: 00172 SunRiseSet[0][1]++; 00173 break; 00174 case SUNSET_HOUR: 00175 SunRiseSet[1][0]++; 00176 break; 00177 case SUNSET_MINUTE: 00178 SunRiseSet[1][1]++; 00179 break; 00180 } 00181 qei.reset(); 00182 } 00183 00184 if(qei_pb()) { //button press 00185 Timer qei_pb_t; 00186 if (qei_pb_t.read() == 0) { 00187 qei_pb_t.start(); 00188 } 00189 while(qei_pb()) { //check long press 00190 if(qei_pb_t.read() > 1) { 00191 qei_pb_t.stop(); 00192 pc.printf("long press");//do stuff on long press 00193 refresh_display = true; 00194 lcd.cls(); 00195 lcd.locate(0,0); 00196 lcd.printf("%s", activeMenu->menuID); 00197 lcd.locate(0,1); 00198 lcd.printf("%s", activeMenu->selections[cursorPos].selText); 00199 00200 menu_state = SETTINGS; 00201 wait(0.5); 00202 } 00203 } 00204 if(qei_pb_t.read() < 1) { 00205 qei_pb_t.stop(); 00206 //do stuff on short press 00207 pc.printf("short press"); 00208 refresh_display = true; 00209 switch (menu_state) { 00210 case MAIN: 00211 break; 00212 case SETTINGS: 00213 pc.printf("apasat SETTINGS\n"); 00214 if(activeMenu->selections[cursorPos].fun != NULL) { 00215 (activeMenu->selections[cursorPos].fun)(); 00216 } 00217 if(activeMenu->selections[cursorPos].childMenu != NULL) { 00218 activeMenu = activeMenu->selections[cursorPos].childMenu; 00219 cursorPos = 0; 00220 } 00221 // print_menu(); 00222 wait(0.2); 00223 break; 00224 case PWM_MIN: 00225 menu_state = SETTINGS; 00226 wait(0.2); 00227 break; 00228 case PWM_MAX: 00229 menu_state = SETTINGS; 00230 wait(0.2); 00231 break; 00232 case SUNRISE: 00233 wait(0.2); 00234 menu_state = SUNRISE_HOUR; 00235 break; 00236 case SUNRISE_HOUR: 00237 wait(0.2); 00238 menu_state = SUNRISE_MINUTE; 00239 break; 00240 case SUNSET: 00241 wait(0.2); 00242 menu_state = SUNSET_HOUR; 00243 break; 00244 case SUNSET_HOUR: 00245 wait(0.2); 00246 menu_state = SUNSET_MINUTE; 00247 break; 00248 default: 00249 menu_state = SETTINGS; 00250 wait(0.2); 00251 break; 00252 } 00253 wait(0.2); 00254 } 00255 } 00256 } 00257 00258 void exit_settings() 00259 { 00260 // menu_state = SAVE_SETTINGS; 00261 // lcd.locate(0,1); 00262 // lcd.printf("<N SAVE Y>"); 00263 menu_state = MAIN; 00264 } 00265 00266 void pwm_min() 00267 { 00268 menu_state = PWM_MIN; 00269 } 00270 00271 void pwm_max() 00272 { 00273 menu_state = PWM_MAX; 00274 } 00275 00276 void sun_rise() 00277 { 00278 menu_state = SUNRISE; 00279 } 00280 00281 void sun_set() 00282 { 00283 menu_state = SUNSET; 00284 } 00285 00286 void ramp_time() 00287 { 00288 menu_state = RAMPTIME; 00289 } 00290 00291 void set_date_time() 00292 { 00293 menu_state = SET_DATE_TIME; 00294 } 00295 00296 // void inc() { 00297 // _pin = _pin + 0.01/8.0; // 0.01 = 1% => 0.125% increments, if you modify this you need to modify 'ramp/8' too 00298 // } 00299 // void dec() { 00300 // _pin = _pin - 0.01/8.0; 00301 // } 00302 00303 //void bl(uint8_t pwmnameArray[10][3], PwmOut &pwmname, Ticker &pwmname_t, Ramp &pwmname_r) //pwmnameArray, pwmname, pwmname_t, pwmname_r 00304 //void bl() 00305 //{ 00306 // struct tm *t; 00307 // time_t seconds = time(NULL); 00308 // t = localtime(&seconds); 00309 // 00310 // int timerica = (t->tm_hour*3600) + (t->tm_min*60) + t->tm_sec; 00311 // 00312 // for (uint8_t index = 0; index < 10; index++) { //cycle array (sizeof(pwmArray)/sizeof(pwmArray[0])) = 0? 00313 // 00314 //// if (pwmnameArray[index][2] != 255 && pwmnameArray[index+1][2] != 255) { 00315 //// 00316 //// if ( timerica == (pwmnameArray[index][0]*3600) + (pwmnameArray[index][1]*60) ) { 00317 //// 00318 //// pwmname_t.detach(); 00319 //// 00320 //// pwmname = pwmnameArray[index][2]/100.0; 00321 //// 00322 //// float ramp = float( (((pwmnameArray[index+1][0]*3600) + (pwmnameArray[index+1][1]*60))-((pwmnameArray[index][0]*3600) + (pwmnameArray[index][1]*60))) ) / float( (pwmnameArray[index+1][2]-pwmnameArray[index][2]) ) ; 00323 //// 00324 //// pc.printf("ramp ?%f\n", ramp); 00325 //// 00326 //// if (ramp > 0) { 00327 //// pwmname_t.attach(&pwmname_r, &Ramp::inc, ramp/8); 00328 //// } 00329 //// if (ramp < 0) { 00330 //// pwmname_t.attach(&pwmname_r, &Ramp::dec, ramp/8); 00331 //// } 00332 //// 00333 //// pc.printf("PWMSTART\n"); 00334 //// pc.printf("index %d\n",index); 00335 //// } 00336 //// } 00337 // 00338 //// if (pwmnameArray[index][2] != 255 && pwmnameArray[index+1][2] == 255) { //pwm_end = 255 00339 //// if ( timerica == (pwmnameArray[index][0]*3600) + (pwmnameArray[index][1]*60) ) { 00340 //// 00341 //// pwmname_t.detach(); 00342 //// 00343 //// pwmname = pwmnameArray[index][2]/100.0; 00344 //// 00345 //// pc.printf("PWMZERO\n"); 00346 //// pc.printf("index %d\n",index); 00347 //// } 00348 //// } 00349 // } 00350 //} 00351 00352 void incs() 00353 { 00354 for(uint8_t index = 0; index < sizeof(Pwm_Array)/sizeof(FastPWM); index++) { //index < sizeof(Pwm_Array)/sizeof(FastPWM) 00355 00356 double ramp = double( (Pwm_Min_Max_Array[index][1]-Pwm_Min_Max_Array[index][0]) ) / double( (( ((SunRiseSet[0][0]*3600) + (SunRiseSet[0][1]*60))+(ramptime*60) )-( (SunRiseSet[0][0]*3600) + (SunRiseSet[0][1]*60) )) ) ; 00357 00358 if (Pwm_Array[index].read() < Pwm_Min_Max_Array[index][1]/100.0) { 00359 Pwm_Array[index] = Pwm_Array[index].read() + double(ramp/100.0); 00360 pc.printf("sunrise:[%i]%f ", index, Pwm_Array[index].read()); 00361 } 00362 if (Pwm_Array[index].read() > Pwm_Min_Max_Array[index][1]/100.0) { 00363 Pwm_Array[index] = Pwm_Min_Max_Array[index][1]/100.0; 00364 Sunrise_Ticker.detach(); 00365 pc.printf("\n sunrise end:[%i]%f \n", index, Pwm_Array[index].read()); 00366 } 00367 } 00368 } 00369 00370 void decs() 00371 { 00372 for(uint8_t index = 0; index < sizeof(Pwm_Array)/sizeof(FastPWM); index++) { //index < sizeof(Pwm_Array)/sizeof(FastPWM) 00373 00374 double ramp = double( (Pwm_Min_Max_Array[index][1]-Pwm_Min_Max_Array[index][0]) ) / double( (( ((SunRiseSet[1][0]*3600) + (SunRiseSet[1][1]*60))+(ramptime*60) )-( (SunRiseSet[1][0]*3600) + (SunRiseSet[1][1]*60) )) ) ; 00375 00376 if (Pwm_Array[index].read() > Pwm_Min_Max_Array[index][0]/100.0) { 00377 Pwm_Array[index] = Pwm_Array[index].read() - double(ramp/100.0); 00378 pc.printf("sunset:[%i]%f ", index, Pwm_Array[index].read()); 00379 } 00380 if (Pwm_Array[index].read() < Pwm_Min_Max_Array[index][0]/100.0) { 00381 Pwm_Array[index] = Pwm_Min_Max_Array[index][0]/100.0; 00382 Sunset_Ticker.detach(); 00383 pc.printf("\n sunset end:[%i]%f \n", index, Pwm_Array[index].read()); 00384 } 00385 } 00386 } 00387 00388 //TODO 00389 //init RTC 00390 00391 void set_time_from_rtc() 00392 { 00393 int rtc_hour; 00394 int rtc_min; 00395 int rtc_sec; 00396 int rtc_dow; //day of week 00397 int rtc_mday; 00398 int rtc_mon; 00399 int rtc_year; 00400 00401 rtc.readDateTime(&rtc_dow,&rtc_mday,&rtc_mon,&rtc_year,&rtc_hour,&rtc_min,&rtc_sec); 00402 //TODO 00403 //asuming that day of week is allways != 0, return an error if dow == 0. 00404 lcd.cls(); 00405 lcd.printf("rtc: %i / %02i-%02i-%02i %02i:%02i:%02i",rtc_dow,rtc_mday,rtc_mon,rtc_year,rtc_hour,rtc_min,rtc_sec); 00406 00407 00408 t.tm_sec = rtc_sec; // 0-59 00409 t.tm_min = rtc_min; // 0-59 00410 t.tm_hour = rtc_hour; // 0-23 00411 t.tm_mday = rtc_mday; // 1-31 00412 t.tm_mon = rtc_mon; // 0-11 00413 t.tm_year = rtc_year-1900; // year since 1900 00414 00415 // convert to timestamp and set (1256729737) 00416 time_t epoch = mktime(&t); 00417 set_time(epoch); 00418 wait(1); //not necessary 00419 lcd.cls(); 00420 epoch = time(NULL); 00421 lcd.printf("stm: %i / %02i-%02i-%02i %02i:%02i:%02i",rtc_dow,localtime(&epoch)->tm_mday,localtime(&epoch)->tm_mon,localtime(&epoch)->tm_year+1900,localtime(&epoch)->tm_hour,localtime(&epoch)->tm_min,localtime(&epoch)->tm_sec); 00422 wait(1); //not necessary 00423 } 00424 00425 void read_eeprom() //int nbyte_read( int mem_addr, void *data, int size ); //TODO 00426 { 00427 char data2[4096]; //32Kbit eeprom 00428 // pc.printf("memory int data read!\n"); 00429 eeprom.nbyte_read( 0, &data2, 4096 ); //MAXADR_24LCXXX <=4096 00430 //lcd.printf("int:%d sizeofint:%d",data2, sizeof(uint8_t)); 00431 // pc.printf("\nend\n"); 00432 lcd.printf("sizeof: %d", sizeof(data2)); 00433 } 00434 00435 int main() 00436 { 00437 //lcd init 00438 lcd.setBacklight(TextLCD::LightOn); 00439 lcd.setMode(TextLCD::DispOn); 00440 00441 //TODO 00442 //rtc init (set 32KHz output to enable, erase OSF, etc? 00443 00444 00445 char buffer[32]; 00446 struct tm *twhile; 00447 00448 set_time_from_rtc(); //read time from DS3231, set time to STM32 00449 //read external EEPROM and restore saved values (what values?) 00450 //lcd.cls(); 00451 //read_eeprom(); 00452 //wait(4); 00453 00454 //TODO set initial pwm_channel state (ie: if it's betweeen sunrise_end and sunset_start set it to sunrise_max, if it's ramping calculate the initial value 00455 00456 //initial state = pwm_min 00457 for(uint8_t index = 0; index < sizeof(Pwm_Array)/sizeof(FastPWM); index++) { 00458 Pwm_Array[index].period_ms(2); //500Hz 00459 Pwm_Array[index] = Pwm_Min_Max_Array[index][0]/100.0; 00460 } 00461 00462 //see if we are between sunrise and sunset 00463 time_t seconds = time(NULL); 00464 twhile = localtime(&seconds); 00465 00466 int timerica = (twhile->tm_hour*3600) + (twhile->tm_min*60) + twhile->tm_sec; 00467 00468 if ((timerica > (SunRiseSet[0][0]*3600) + (SunRiseSet[0][1]*60)) && (timerica < (SunRiseSet[1][0]*3600) + (SunRiseSet[1][1]*60))) { 00469 for(uint8_t index = 0; index < sizeof(Pwm_Array)/sizeof(FastPWM); index++) { 00470 Pwm_Array[index] = Pwm_Min_Max_Array[index][1]/100.0; //add smoothing 00471 pc.printf("pwm:[%i]%f ", index, Pwm_Array[index].read()); 00472 } 00473 } 00474 00475 00476 00477 qei_idx.mode(PullUp); 00478 qei_t.attach(&qei_cb, 0.05); //calls qei_cb every 50ms 00479 00480 Menu settingsMenu("SettingsID"); 00481 00482 Menu pwm_minMenu("PWM MinID"); 00483 pwm_minMenu.add(Selection(&pwm_min, 0, NULL, "< CH00 >")); 00484 pwm_minMenu.add(Selection(&pwm_min, 1, NULL, "< CH01 >")); 00485 pwm_minMenu.add(Selection(&pwm_min, 2, NULL, "< CH02 >")); 00486 pwm_minMenu.add(Selection(&pwm_min, 3, NULL, "< CH03 >")); 00487 pwm_minMenu.add(Selection(&pwm_min, 4, NULL, "< CH04 >")); 00488 pwm_minMenu.add(Selection(&pwm_min, 5, NULL, "< CH05 >")); 00489 pwm_minMenu.add(Selection(&pwm_min, 6, NULL, "< CH06 >")); 00490 pwm_minMenu.add(Selection(&pwm_min, 7, NULL, "< CH07 >")); 00491 pwm_minMenu.add(Selection(&pwm_min, 8, NULL, "< CH08 >")); 00492 pwm_minMenu.add(Selection(&pwm_min, 9, NULL, "< CH09 >")); 00493 pwm_minMenu.add(Selection(NULL, 10, &settingsMenu, "< Return >")); 00494 00495 Menu pwm_maxMenu("PWM MaxID"); 00496 pwm_maxMenu.add(Selection(&pwm_max, 0, NULL, "< CH00 >")); 00497 pwm_maxMenu.add(Selection(&pwm_max, 1, NULL, "< CH01 >")); 00498 pwm_maxMenu.add(Selection(&pwm_max, 2, NULL, "< CH02 >")); 00499 pwm_maxMenu.add(Selection(&pwm_max, 3, NULL, "< CH03 >")); 00500 pwm_maxMenu.add(Selection(&pwm_max, 4, NULL, "< CH04 >")); 00501 pwm_maxMenu.add(Selection(&pwm_max, 5, NULL, "< CH05 >")); 00502 pwm_maxMenu.add(Selection(&pwm_max, 6, NULL, "< CH06 >")); 00503 pwm_maxMenu.add(Selection(&pwm_max, 7, NULL, "< CH07 >")); 00504 pwm_maxMenu.add(Selection(&pwm_max, 8, NULL, "< CH08 >")); 00505 pwm_maxMenu.add(Selection(&pwm_max, 9, NULL, "< CH09 >")); 00506 pwm_maxMenu.add(Selection(NULL, 10, &settingsMenu, "< Return >")); 00507 00508 // Menu sunriseMenu("PWM MaxID"); 00509 // 00510 // Menu sunsetMenu("PWM MaxID"); 00511 00512 // Menu ramptimeMenu("RampTimeID"); 00513 00514 settingsMenu.add(Selection(NULL, 0, &pwm_maxMenu, "<PWM Day>")); 00515 settingsMenu.add(Selection(NULL, 1, &pwm_minMenu, "<PWM Night>")); 00516 settingsMenu.add(Selection(&sun_rise, 2, NULL, "<Sunrise>")); 00517 settingsMenu.add(Selection(&sun_set, 3, NULL, "<Sunset>")); 00518 settingsMenu.add(Selection(&ramp_time, 4, NULL, "<Ramp Time>")); 00519 settingsMenu.add(Selection(&set_date_time, 5, NULL, "<Set Date/Time>")); 00520 00521 settingsMenu.add(Selection(&exit_settings, 6, NULL, "<Exit Setup>")); 00522 00523 activeMenu = &settingsMenu; 00524 00525 while(1) { 00526 00527 time_t seconds = time(NULL); 00528 strftime(buffer, 32, "%H:%M:%S", localtime(&seconds)); 00529 00530 00531 twhile = localtime(&seconds); 00532 00533 int timerica = (twhile->tm_hour*3600) + (twhile->tm_min*60) + twhile->tm_sec; 00534 00535 00536 pc.printf("time: %s in seconds: %d sunrise: %d sunset: %d\n", buffer, timerica, (SunRiseSet[0][0]*3600) + (SunRiseSet[0][1]*60), (SunRiseSet[1][0]*3600) + (SunRiseSet[1][1]*60) ); 00537 00538 if (timerica == (SunRiseSet[0][0]*3600) + (SunRiseSet[0][1]*60)) { //TODO attach once at start out of while() 00539 Sunrise_Ticker.attach(&incs,1); //TODO check if allready attached 00540 } 00541 00542 if (timerica == (SunRiseSet[1][0]*3600) + (SunRiseSet[1][1]*60)) { 00543 Sunset_Ticker.attach(&decs,1); //TODO check if allready attached 00544 pc.printf("sunset timer attached to decs\n"); 00545 } 00546 00547 // for(uint8_t index = 0; index < 3; index++) { //index < sizeof(Pwm_Array)/sizeof(FastPWM) 00548 // pc.printf("pwm:[%i]%f ", index, Pwm_Array[index].read()); 00549 // } 00550 00551 00552 if (refresh_display == true) { 00553 switch(menu_state) { 00554 case MAIN: 00555 lcd.cls(); 00556 lcd.printf(" BAT-LED "); 00557 lcd.printf(" v.1.0 "); 00558 refresh_display = false; 00559 break; 00560 case SETTINGS: 00561 lcd.cls(); 00562 lcd.locate(0,0); 00563 lcd.printf("%s", activeMenu->menuID); 00564 lcd.locate(0,1); 00565 lcd.printf("%s", activeMenu->selections[cursorPos].selText); 00566 refresh_display = false; 00567 break; 00568 case PWM_MIN: 00569 lcd.cls(); 00570 lcd.printf("%s %2i", activeMenu->menuID, cursorPos); 00571 lcd.locate(0,1); 00572 lcd.printf("<- %3i%% +>", Pwm_Min_Max_Array[cursorPos][0]); 00573 refresh_display = false; 00574 break; 00575 case PWM_MAX: 00576 lcd.cls(); 00577 lcd.printf("%s %2i", activeMenu->menuID, cursorPos); 00578 lcd.locate(0,1); 00579 lcd.printf("<- %3i%% +>", Pwm_Min_Max_Array[cursorPos][1]); 00580 refresh_display = false; 00581 break; 00582 case SUNRISE: 00583 lcd.cls(); 00584 lcd.printf("%s %2i", activeMenu->menuID, cursorPos); 00585 lcd.locate(0,1); 00586 lcd.printf("%02d:%02d", SunRiseSet[0][0],SunRiseSet[0][1]); 00587 refresh_display = false; 00588 break; 00589 case SUNRISE_HOUR: 00590 while(menu_state == SUNRISE_HOUR) { 00591 lcd.locate(0,1); 00592 lcd.printf(" "); 00593 wait(0.1); 00594 lcd.locate(0,1); 00595 lcd.printf("%02d",SunRiseSet[0][0]); 00596 wait(0.2); 00597 } 00598 break; 00599 case SUNRISE_MINUTE: 00600 while(menu_state == SUNRISE_MINUTE) { 00601 lcd.locate(3,1); 00602 lcd.printf(" "); 00603 wait(0.1); 00604 lcd.locate(3,1); 00605 lcd.printf("%02d",SunRiseSet[0][1]); 00606 wait(0.2); 00607 } 00608 break; 00609 case SUNSET: 00610 lcd.cls(); 00611 lcd.printf("%s %2i", activeMenu->menuID, cursorPos); 00612 lcd.locate(0,1); 00613 lcd.printf("%02d:%02d", SunRiseSet[1][0],SunRiseSet[1][1]); 00614 refresh_display = false; 00615 break; 00616 case SUNSET_HOUR: 00617 while(menu_state == SUNSET_HOUR) { 00618 lcd.locate(0,1); 00619 lcd.printf(" "); 00620 wait(0.1); 00621 lcd.locate(0,1); 00622 lcd.printf("%02d",SunRiseSet[1][0]); 00623 wait(0.2); 00624 } 00625 break; 00626 case SUNSET_MINUTE: 00627 while(menu_state == SUNSET_MINUTE) { 00628 lcd.locate(3,1); 00629 lcd.printf(" "); 00630 wait(0.1); 00631 lcd.locate(3,1); 00632 lcd.printf("%02d",SunRiseSet[1][1]); 00633 wait(0.2); 00634 } 00635 break; 00636 case RAMPTIME: 00637 lcd.cls(); 00638 lcd.printf("%s %2i", activeMenu->menuID, cursorPos); 00639 lcd.locate(0,1); 00640 lcd.printf("<- %3i +>", ramptime); 00641 refresh_display = false; 00642 break; 00643 case SET_DATE_TIME: 00644 lcd.cls(); 00645 lcd.printf("%02d/%02d/%04d",twhile->tm_mday, twhile->tm_mon, twhile->tm_year + 1900); 00646 lcd.locate(0,1); 00647 lcd.printf("%02d:%02d:%02d",twhile->tm_hour, twhile->tm_min, twhile->tm_sec); 00648 refresh_display = false; 00649 break; 00650 } 00651 } 00652 wait(0.5); 00653 } 00654 }
Generated on Sun Jul 17 2022 02:50:17 by
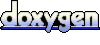