
DEMONSTRATION OF SIGNAL (EVENT & FLAG) in MBED RTOS. One Thread set particular signal and another thread receive it and proceed according signal state.
main.cpp
00001 /* SIGNAL USAGES IN RTOS 00002 What is Signal ? - Answer : Remember these five points 00003 00004 1) Signals are different from all the other types of kernel object in that they are not autonomous (user need to control or set - No automatioc control like semaphore) 00005 2) signals are associated with tasks/Threads and have no independent existence. 00006 3) If signals are configured for an application, each task has a set of eight signal flags. 00007 00008 4) Any task can set the signals of another task. Only the owner task can read the signals. 00009 5) The read is destructive – i.e. the signals are cleared by the process of reading. No other task can read or clear a task’s signals. 00010 00011 00012 In below example there are two threads 1)main thread and 2)Thread that is connected with onBoard_led functions 00013 We set the signal from main thread , second thread received the signal and toggle the led state. 00014 00015 Program name : LED BLINKING USING RTOS SIGNAL 00016 PLATFORM : STM NUCLEO 64 L476 00017 Created by : jaydeep shah 00018 email : radhey04ec@gmail.com 00019 */ 00020 00021 /* NOTE : WE ARE GOING TO USE EVENT FLAG CLASS METHOD The EventFlags class is used to control event flags or wait for event flags other threads control. 00022 */ 00023 #include "mbed.h" 00024 #include "rtos.h" 00025 00026 DigitalOut led(LED2); //ON BOARD LED 00027 00028 EventFlags event_flags; // EVESNT FLAG OBJECT CREATED (DOMAIN : SIGNAL) 00029 00030 //TASK which is related to signal 00031 void onBoard_led() { 00032 uint32_t read = 0; //CREATE VARIABLE TO STORE DATA 00033 while (true) { 00034 // Signal flags that are reported as event are automatically cleared -Autonomous object of Kernal 00035 00036 read = event_flags.wait_any(0x1 | 0x2); 00037 if(read == 0x1) 00038 { 00039 led = !led; //Toggle state 00040 } 00041 printf("\n Received this flag event 0x%08lx \n\r",read); 00042 00043 } 00044 } 00045 00046 int main (void) { 00047 Thread thread; //create threaed 00048 00049 thread.start(callback(onBoard_led)); //Thread start 00050 00051 while (true) { 00052 ThisThread::sleep_for(1000); //sleep for 1 sec 00053 event_flags.set(0x1); //set the signal for thread task from main thread --LED BLINK TASK 00054 ThisThread::sleep_for(1000); 00055 event_flags.set(0x2); //This signal for print the statement -- use serial terminal 00056 } 00057 }
Generated on Sun Jul 31 2022 12:33:08 by
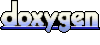