
MUTEX and its use in MBED MUTEX LOCK and Unlock mechanism
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 21_ MUTEX a Guard at door - Basic program 00002 MUTEX allow only one Thread / Function call inside CS, Mutex have ownership type locking mechanism. 00003 Procees /or Thread who locked block,only same process /or thread can unlock it. 00004 */ 00005 00006 00007 //PROGRAM CREATED BY : JAYDEEP SHAH -- radheec@gmail.com 00008 //DATE : 25 JULY 20 ,VERSION 1.0 00009 00010 //OUTPUT : USE SERIAL TERMINAL 9600 8-N-1 00011 00012 00013 #include "mbed.h" //MBED LIBRARY 00014 00015 Mutex M_LOCK; // Create MUTEX OBJECT -class = MUTEX 00016 00017 //Create Two Thread 00018 00019 Thread t2; 00020 00021 Thread t3; 00022 00023 00024 //Here below section act as CS critical section 00025 00026 void common_function(const char *name, int state) //TWO ARGUMENTS 1) WHO CALLED THIS FUNCTION 2)STATE OF CALLER 00027 { 00028 printf("Thread arrive at door %s: %d\n\r", name, state); 00029 00030 M_LOCK.lock(); //After arrive lock the code---------------------------LOCK THE BLOCK 00031 00032 printf("This Thread lock the code %s: %d\n\r", name, state); 00033 wait(0.5); //sleep 00034 printf("Thread cross & unlock %s: %d\n\r", name, state); //OUTSIDE CODE BLOCK --------- 00035 M_LOCK.unlock(); //After completing task unlock the code ------------- UNLOCK THE BLOCK 00036 00037 //DO NOT WRITE any print statement after unlock() it create missunderstanding .... 00038 /* print statement require more clk cycle,after unlock the MUTEX Flag - schedular quickly schedule next thread before print the statement.*/ 00039 } 00040 00041 00042 00043 //Function -- We will connect more than one thread with this function 00044 //So because of context switching this Function become sharable between multiple process. 00045 void test_thread(void const *args) 00046 { 00047 while (true) { 00048 common_function((const char *)args, 0); 00049 ThisThread::sleep_for(500); 00050 common_function((const char *)args, 1); 00051 ThisThread::sleep_for(500); 00052 } 00053 } 00054 00055 int main() 00056 { 00057 t2.start(callback(test_thread, (void *)"Th 2")); 00058 t3.start(callback(test_thread, (void *)"Th 3")); 00059 00060 test_thread((void *)"Th 1"); // DIRECT CALL via main thread 00061 }
Generated on Thu Sep 1 2022 07:55:37 by
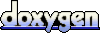