mbed device driver for HC-SR04 ultrasonic range finder
Dependents: ROBot Ultrasonic Ksen Core1000_SmartFarm ... more
HCSR04.h
00001 /* File: HCSR04.h 00002 * Author: Robert Abad Copyright (c) 2013 00003 * 00004 * Desc: driver for HCSR04 Ultrasonic Range Finder. The returned range 00005 * is in units of meters. 00006 * 00007 * To use this driver you must call the methods ::startMeas() 00008 * and ::getMeas(). The HCSR04 requires a trigger time of 00009 * 10 usec (microseconds) which is initiated by ::startMeas(). 00010 * If a successful measurement is made, getMeas() will return 00011 * RANGE_MEAS_VALID. If unsuccessful, initiate a new measurement. 00012 * 00013 * The datasheet for this device can be found here: 00014 * http://www.elecfreaks.com/store/download/product/Sensor/HC-SR04/HC-SR04_Ultrasonic_Module_User_Guide.pdf 00015 * 00016 * Below is some sample code: 00017 * 00018 * #include "mbed.h" 00019 * #include "HCSR04.h" 00020 * 00021 * #define PIN_TRIGGER (p14) 00022 * #define PIN_ECHO (p15) 00023 * 00024 * int main(void) 00025 * { 00026 * HCSR04 rangeFinder( PIN_TRIGGER, PIN_ECHO ); 00027 * float range; 00028 * 00029 * while (1) 00030 * { 00031 * rangeFinder.startMeas(); 00032 * wait(0.1); 00033 * if ( rangeFinder.getMeas(range) == RANGE_MEAS_VALID ) 00034 * { 00035 * printf("range = %f\n\r", range); 00036 * } 00037 * } 00038 * } 00039 */ 00040 00041 #ifndef __HCSR04_H__ 00042 #define __HCSR04_H__ 00043 00044 #include "mbed.h" 00045 00046 typedef enum 00047 { 00048 RANGE_MEAS_INVALID, 00049 RANGE_MEAS_VALID 00050 } etHCSR04_RANGE_STATUS; 00051 00052 class HCSR04 00053 { 00054 public: 00055 HCSR04( PinName pinTrigger, PinName pinEcho ); 00056 void startMeas(void); 00057 etHCSR04_RANGE_STATUS getMeas(float &rRangeMeters); 00058 00059 private: 00060 DigitalOut trigger; 00061 Ticker triggerTicker; 00062 InterruptIn echo; 00063 Timer echoTimer; 00064 unsigned long measTimeStart_us; 00065 unsigned long measTimeStop_us; 00066 00067 void triggerTicker_cb(void); // trigger ticker callback function 00068 void ISR_echoRising(void); // ISR for rising edge 00069 void ISR_echoFalling(void); // ISR for falling edge 00070 }; 00071 00072 #endif /* __HCSR04_H__ */
Generated on Wed Jul 13 2022 07:00:09 by
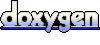