Generic library for working with PN532-like chips
Fork of PN532 by
mac_link.h
00001 00002 00003 #ifndef __MAC_LINK_H__ 00004 #define __MAC_LINK_H__ 00005 00006 #include "PN532.h " 00007 00008 /** 00009 * The MACLink class 00010 */ 00011 class MACLink { 00012 public: 00013 MACLink(PN532Interface &interface) : pn532(interface) { 00014 00015 }; 00016 00017 /** 00018 * @brief Activate PN532 as a target 00019 * @param timeout max time to wait, 0 means no timeout 00020 * @return > 0 success 00021 * = 0 timeout 00022 * < 0 failed 00023 */ 00024 int8_t activateAsTarget(uint16_t timeout = 0); 00025 00026 /** 00027 * @brief write a PDU packet, the packet should be less than (255 - 2) bytes 00028 * @param header packet header 00029 * @param hlen length of header 00030 * @param body packet body 00031 * @param blen length of body 00032 * @return true success 00033 * false failed 00034 */ 00035 bool write(const uint8_t *header, uint8_t hlen, const uint8_t *body = 0, uint8_t blen = 0); 00036 00037 /** 00038 * @brief read a PDU packet, the packet will be less than (255 - 2) bytes 00039 * @param buf the buffer to contain the PDU packet 00040 * @param len lenght of the buffer 00041 * @return >=0 length of the PDU packet 00042 * <0 failed 00043 */ 00044 int16_t read(uint8_t *buf, uint8_t len); 00045 00046 uint8_t *getHeaderBuffer(uint8_t *len) { 00047 return pn532.getBuffer(len); 00048 }; 00049 00050 private: 00051 PN532 pn532; 00052 }; 00053 00054 #endif // __MAC_LINK_H__
Generated on Sun Jul 24 2022 19:12:02 by
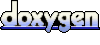