
This program shows how to use the VCNL4010 (proximity and ambient light sensor) on the QW dev kit for detecting an open closet door. When the closet is open, a message will be displayed in the console window and a Sigfox message will be send.
Fork of QW-Closet-detection by
main.cpp
00001 /* This program demonstrates how to use the VCNL4010 for detecting an open closet door. 00002 * Open a serial console to the board to see extra info. 00003 */ 00004 00005 #include "mbed.h" 00006 #include "VCNL4010.h" 00007 00008 #define SER_BUFFER_SIZE 32 00009 00010 /* The 4 onboard LEDs */ 00011 DigitalOut LED_0 (PB_6); 00012 DigitalOut LED_1 (PA_7); 00013 DigitalOut LED_2 (PA_6); 00014 DigitalOut LED_3 (PA_5); 00015 00016 /* The 2 user buttons */ 00017 InterruptIn SW1(PA_8); 00018 InterruptIn SW2(PB_10); 00019 00020 /* Proximity and ambient light sensor*/ 00021 VCNL40x0 VCNL4010(PB_9, PB_8, VCNL40x0_ADDRESS); // SDA, SCL pin and I2C address 00022 00023 /* Function prototypes */ 00024 void sw1interrupt(); 00025 void sw2interrupt(); 00026 void sertmout(); 00027 bool modem_command_check_ok(char * command); 00028 void modem_setup(); 00029 00030 bool ser_timeout = false; 00031 00032 /* Serial port over USB */ 00033 Serial pc(USBTX, USBRX); 00034 00035 /* Serial connection to sigfox modem */ 00036 Serial modem(PA_9, PA_10); 00037 00038 00039 bool indoor = false; 00040 int indoorLight; 00041 00042 00043 int main() { 00044 00045 unsigned char ID=0; 00046 unsigned int AmbiValue=0; 00047 00048 /* Setup TD120x */ 00049 wait(3); 00050 modem_setup(); 00051 00052 /* Turn off all LED */ 00053 LED_0 = 1; 00054 LED_1 = 1; 00055 LED_2 = 1; 00056 LED_3 = 1; 00057 00058 /* Setup button interrupts */ 00059 SW1.fall(&sw1interrupt); 00060 SW2.fall(&sw2interrupt); 00061 00062 /* Read VCNL40x0 product ID revision register */ 00063 VCNL4010.ReadID (&ID); 00064 pc.printf("\n\nProduct ID Revision Register: %d", ID); 00065 00066 wait_ms(3000); //Wait 3s (only for display) 00067 00068 /* Calibrate the system by measuring the indoor light */ 00069 while(indoor != true){ 00070 LED_3 = 0; 00071 wait_ms(500); 00072 LED_3 = 1; 00073 wait_ms(500); 00074 } 00075 VCNL4010.ReadAmbiOnDemand (&AmbiValue); //Read ambi value on demand 00076 indoorLight = AmbiValue; 00077 pc.printf("\rIndoor light value is: %5.0i\n", indoorLight); 00078 00079 while(1) { 00080 VCNL4010.ReadAmbiOnDemand (&AmbiValue); //Read ambi value on demand 00081 00082 if(AmbiValue > (indoorLight+50)){ 00083 LED_0 = 0; //Turn on LED_0 to indicate open closet and Sigfox transmission 00084 pc.printf("\rCloset door has been opened!\n"); 00085 char command[SER_BUFFER_SIZE]; 00086 sprintf(command, "AT$SF=05%04x,2,0\n", (int) AmbiValue ); 00087 pc.printf("Sending ambient value %5.0i over Sigfox using modem command: %s\n", AmbiValue , command); 00088 modem_command_check_ok(command); 00089 LED_0 = 1; //Turn off LED_0 00090 wait_ms(5000); //Wait 5 seconds before checking again 00091 } 00092 } 00093 } 00094 00095 void modem_setup() 00096 { 00097 /* Reset to factory defaults */ 00098 if(modem_command_check_ok("AT&F")) 00099 { 00100 pc.printf("Factory reset succesfull\r\n"); 00101 } 00102 else 00103 { 00104 pc.printf("Factory reset TD120x failed\r\n"); 00105 } 00106 /* Disable local echo */ 00107 modem.printf("ATE0\n"); 00108 if(modem_command_check_ok("ATE0")) 00109 { 00110 pc.printf("Local echo disabled\r\n"); 00111 } 00112 /* Write to mem */ 00113 if(modem_command_check_ok("AT&W")) 00114 { 00115 pc.printf("Settings saved!\r\n"); 00116 } 00117 } 00118 00119 bool modem_command_check_ok(char * command) 00120 { 00121 /* First clear serial data buffers */ 00122 while(modem.readable()) modem.getc(); 00123 /* Timeout for response of the modem */ 00124 Timeout tmout; 00125 ser_timeout = false; 00126 /* Buffer for incoming data */ 00127 char responsebuffer[6]; 00128 /* Flag to set when we get 'OK' response */ 00129 bool ok = false; 00130 bool error = false; 00131 /* Print command to TD120x */ 00132 modem.printf(command); 00133 /* Newline to activate command */ 00134 modem.printf("\n"); 00135 /* Wait untill serial feedback, min 7 seconds before timeout */ 00136 tmout.attach(&sertmout, 7.0); 00137 while(!modem.readable()&& ser_timeout == false); 00138 while(!ok && !ser_timeout && !error) 00139 { 00140 if(modem.readable()) 00141 { 00142 for(int i = 0; i < 5; i++) 00143 { 00144 responsebuffer[i] = responsebuffer[i+1]; 00145 } 00146 responsebuffer[5] = modem.getc(); 00147 if(responsebuffer[0] == '\r' && responsebuffer[1] == '\n' && responsebuffer[2] == 'O' && responsebuffer[3] == 'K' && responsebuffer[4] == '\r' && responsebuffer[5] == '\n' ) 00148 { 00149 ok = true; 00150 } 00151 else if(responsebuffer[0] == '\r' && responsebuffer[1] == '\n' && responsebuffer[2] == 'E' && responsebuffer[3] == 'R' && responsebuffer[4] == 'R' && responsebuffer[5] == 'O' ) 00152 { 00153 error = true; 00154 } 00155 } 00156 } 00157 tmout.detach(); 00158 return ok; 00159 } 00160 00161 /* Button 1 ISR */ 00162 void sw1interrupt() 00163 { 00164 pc.printf("\n\rButton 1 pressed\n\r"); 00165 indoor = true; 00166 pc.printf("\n\rIndoor light measured\n\r"); 00167 } 00168 00169 /* Button 2 ISR */ 00170 void sw2interrupt() 00171 { 00172 pc.printf("\n\rButton 2 pressed\n\r"); 00173 indoor = true; 00174 pc.printf("\n\rIndoor light measured\n\r"); 00175 00176 } 00177 00178 /* ISR for serial timeout */ 00179 void sertmout() 00180 { 00181 ser_timeout = true; 00182 } 00183 00184 00185
Generated on Sun Jul 17 2022 22:00:27 by
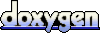