This is a VERY low-level library for controlling the TSI hardware module in a KL25 microcontroller. The programmer creates the TSI object passing basic parameters, and selects the active channels. Then, a scan on a given channel can be started, and the raw result can be read.
TSIHW.cpp
00001 #include "mbed.h" 00002 #include "TSIHW.h" 00003 00004 /* TSI constructor 00005 Parameters: the values of the fields REFCHRG, EXTCHRG, DVOLT, PS, and NSCN from TSI0_GENCS register 00006 Not using interrupts, capacitive sensing (non-noise detection), not configured to work in STOP modes 00007 */ 00008 TSI::TSI(char rchg, char echg, char dvolt, char ps, char nscn) { 00009 // The first version is preconfigured for non-noise detection, no interrupts, not running on stop modes, software trigger 00010 SIM->SCGC5 |= 0x00000E20; // clock gate for TSI and PORTS A, B, and C 00011 TSI0->GENCS = 0xC0000080 | ((rchg & 0x07) << 21) | ((echg & 0x07) << 16) | ((dvolt & 0x03) << 19) | ((ps & 0x07) << 13) | ((nscn & 0x1F) << 8); 00012 } 00013 00014 /* TSI destructor */ 00015 TSI::~TSI() { } 00016 00017 /* Function to configure a pin to work with the corresponding channel (passed as the single parameter) */ 00018 void TSI::ActivateChannel(char ch) { 00019 // reads channel number and sets the MUX of the corresponding pin to ALT0 function 00020 switch(ch) { 00021 case 0: PORTB->PCR[0] &= 0xFFFFF8FF; break; 00022 case 1: PORTA->PCR[0] &= 0xFFFFF8FF; break; 00023 case 2: PORTA->PCR[1] &= 0xFFFFF8FF; break; 00024 case 3: PORTA->PCR[2] &= 0xFFFFF8FF; break; 00025 case 4: PORTA->PCR[3] &= 0xFFFFF8FF; break; 00026 case 5: PORTA->PCR[4] &= 0xFFFFF8FF; break; 00027 case 6: PORTB->PCR[1] &= 0xFFFFF8FF; break; 00028 case 7: PORTB->PCR[2] &= 0xFFFFF8FF; break; 00029 case 8: PORTB->PCR[3] &= 0xFFFFF8FF; break; 00030 case 9: PORTB->PCR[16] &= 0xFFFFF8FF; break; 00031 case 10: PORTB->PCR[17] &= 0xFFFFF8FF; break; 00032 case 11: PORTB->PCR[18] &= 0xFFFFF8FF; break; 00033 case 12: PORTB->PCR[19] &= 0xFFFFF8FF; break; 00034 case 13: PORTC->PCR[0] &= 0xFFFFF8FF; break; 00035 case 14: PORTC->PCR[1] &= 0xFFFFF8FF; break; 00036 case 15: PORTC->PCR[2] &= 0xFFFFF8FF; break; 00037 default: error("PinName provided to TSI::ActivateChannel() does not correspond to any known TSI channel."); 00038 } 00039 } 00040 00041 // Function to trigger the reading of a given channel 00042 void TSI::Start(char ch) { 00043 //writes 1 to the software trigger bit, defining the channel number in the respective bits 00044 TSI0->GENCS |= 0x00000004; // clears EOSF 00045 TSI0->DATA = 0x00400000 | (ch << 28); 00046 } 00047 00048 // Function to read scan result; returns zero if not finished 00049 uint16_t TSI::Read() { 00050 uint16_t aux; 00051 00052 if(!(TSI0->GENCS & 0x00000004)) { 00053 return 0; 00054 } else { 00055 aux = TSI0->DATA & 0x0000FFFF; 00056 TSI0->GENCS |= 0x00000004; // clears EOSF 00057 return aux; 00058 } 00059 }
Generated on Thu Jul 14 2022 13:17:10 by
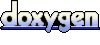