
Library implementing menu system, with RPG interface, navigator system
Dependencies: Menu RPG TextLCD mbed
main.cpp
00001 #include "mbed.h" 00002 #include "TextLCD.h" 00003 #include "RPG.h" 00004 #include "Selection.h" 00005 #include "Menu.h" 00006 #include "Navigator.h" 00007 #include <vector> 00008 #include <string> 00009 00010 DigitalOut led1(LED1), led2(LED2), led3(LED3), led4(LED4); 00011 TextLCD lcd(p15, p16, p17, p18, p19, p20); // rs, e, d4-d7 00012 RPG rpg(p21,p22,p23); 00013 00014 using namespace std; 00015 00016 // some functions to tie to selections 00017 void toggleLED1() { led1 = !led1; } 00018 void toggleLED2() { led2 = !led2; } 00019 void toggleLED3() { led3 = !led3; } 00020 void toggleLED4() { led4 = !led4; } 00021 void resetLED() 00022 { 00023 led1 = 0; 00024 led2 = 0; 00025 led3 = 0; 00026 led4 = 0; 00027 } 00028 00029 int main() { 00030 // In using this library, the user declares "Menus", which are essentially arrays of "Selections". 00031 // Selections describe each individual selectable item. 00032 // Selections can be tied to functions. Functions must output void and take in no arguments. 00033 // Selections can also have references to child menus. 00034 00035 // It makes sense to declare a root menu first, but you don't have to. 00036 // Menus should have an identifier (the argument in constructor). 00037 Menu rootMenu("root"); 00038 00039 // Selections are added to menus through the Menu's "add" method. 00040 // If a function is to be executed when the RPG is depressed, make a REFERENCE to it. Otherwise null. 00041 // The second argument is its position - the will be deprecated soon. 00042 // The last is the text to display. *INCLUDE A SPACE IN THE BEGINNING, this is where the cursor goes. 00043 // *This means the text is limited to 14 characters (not counting the space) with this display. 00044 // **It is 14 not 15 for reasons pertaining to the implementation of the TextLCD library. 00045 Menu ledMenu("LED menu"); 00046 ledMenu.add(Selection(&toggleLED1, 0, NULL, " Toggle LED1")); // The function argument of selection can be added directly 00047 ledMenu.add(Selection(NULL, 1, NULL, " Toggle LED2")); // It can also be set to NULL temporarily and changed later. 00048 ledMenu.selections[1].fun = &toggleLED2; // Useful for when functions are methods in other classes. 00049 ledMenu.add(Selection(&toggleLED3, 2, NULL, " Toggle LED3")); 00050 ledMenu.add(Selection(&toggleLED4, 3, NULL, " Toggle LED4")); 00051 ledMenu.add(Selection(&resetLED, 4, &rootMenu, " Go back")); // always add a Selection at the end to point to the parent 00052 00053 Menu aboutMenu("About Menu"); // about menu crediting us :) 00054 aboutMenu.add(Selection(NULL, 0, NULL, " Authors:")); 00055 aboutMenu.add(Selection(NULL, 1, NULL, " Ben Y & Tony T")); 00056 aboutMenu.add(Selection(NULL, 2, NULL, " ECE4180")); 00057 aboutMenu.add(Selection(NULL, 3, &rootMenu, " Go back")); 00058 00059 // Selections to the root menu should be added last 00060 rootMenu.add(Selection(NULL, 0, &ledMenu, " LED MENU")); 00061 rootMenu.add(Selection(NULL, 1, NULL, " Dummy menu 1")); // a dummy menu, doesn't do anything 00062 rootMenu.add(Selection(NULL, 2, &aboutMenu, " About menu")); 00063 00064 // Here is the heart of the system: the navigator. 00065 // The navigator takes in a reference to the root, an interface, and a reference to an lcd 00066 Navigator navigator(&rootMenu, rpg, &lcd); 00067 00068 while(1){ 00069 navigator.poll(); // In a loop, call navigator's poll method to determine if the user is interacting with the rpg. 00070 } 00071 }
Generated on Sat Jul 16 2022 07:47:52 by
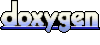