BLE shield
Fork of X_NUCLEO_IDB0XA1 by
Embed:
(wiki syntax)
Show/hide line numbers
BlueNRGGattServer.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 /** 00017 ****************************************************************************** 00018 * @file BlueNRGGattServer.cpp 00019 * @author STMicroelectronics 00020 * @brief Header file for BLE_API GattServer Class 00021 ****************************************************************************** 00022 * @copy 00023 * 00024 * THE PRESENT FIRMWARE WHICH IS FOR GUIDANCE ONLY AIMS AT PROVIDING CUSTOMERS 00025 * WITH CODING INFORMATION REGARDING THEIR PRODUCTS IN ORDER FOR THEM TO SAVE 00026 * TIME. AS A RESULT, STMICROELECTRONICS SHALL NOT BE HELD LIABLE FOR ANY 00027 * DIRECT, INDIRECT OR CONSEQUENTIAL DAMAGES WITH RESPECT TO ANY CLAIMS ARISING 00028 * FROM THE CONTENT OF SUCH FIRMWARE AND/OR THE USE MADE BY CUSTOMERS OF THE 00029 * CODING INFORMATION CONTAINED HEREIN IN CONNECTION WITH THEIR PRODUCTS. 00030 * 00031 * <h2><center>© COPYRIGHT 2013 STMicroelectronics</center></h2> 00032 */ 00033 00034 // ANDREA: Changed some types (e.g., tHalUint8 --> uint8_t) 00035 00036 #ifndef __BLUENRG_GATT_SERVER_H__ 00037 #define __BLUENRG_GATT_SERVER_H__ 00038 00039 #include "mbed.h" 00040 #include "ble/blecommon.h" 00041 #include "btle.h" 00042 #include "ble/GattService.h" 00043 #include "ble/GattServer.h" 00044 #include <vector> 00045 #include <map> 00046 00047 #define BLE_TOTAL_CHARACTERISTICS 10 00048 00049 // If the char has handle 'x', then the value declaration will have the handle 'x+1' 00050 // If the char has handle 'x', then the char descriptor declaration will have the handle 'x+2' 00051 #define CHAR_VALUE_OFFSET 1 00052 #define CHAR_DESC_OFFSET 2 00053 00054 using namespace std; 00055 00056 class BlueNRGGattServer : public GattServer 00057 { 00058 public: 00059 static BlueNRGGattServer &getInstance() { 00060 static BlueNRGGattServer m_instance; 00061 return m_instance; 00062 } 00063 00064 enum HandleEnum_t { 00065 CHAR_HANDLE = 0, 00066 CHAR_VALUE_HANDLE, 00067 CHAR_DESC_HANDLE 00068 }; 00069 00070 /* Functions that must be implemented from GattServer */ 00071 // <<<ANDREA>>> 00072 virtual ble_error_t addService(GattService &); 00073 virtual ble_error_t read(GattAttribute::Handle_t attributeHandle, uint8_t buffer[], uint16_t *lengthP); 00074 virtual ble_error_t read(Gap::Handle_t connectionHandle, GattAttribute::Handle_t attributeHandle, uint8_t buffer[], uint16_t *lengthP); 00075 virtual ble_error_t write(GattAttribute::Handle_t, const uint8_t[], uint16_t, bool localOnly = false); 00076 virtual ble_error_t write(Gap::Handle_t connectionHandle, GattAttribute::Handle_t, const uint8_t[], uint16_t, bool localOnly = false); 00077 virtual ble_error_t initializeGATTDatabase(void); 00078 00079 virtual bool isOnDataReadAvailable() const { 00080 return true; 00081 } 00082 // <<<ANDREA>>> 00083 00084 /* BlueNRG Functions */ 00085 void eventCallback(void); 00086 //void hwCallback(void *pckt); 00087 ble_error_t Read_Request_CB(uint16_t handle); 00088 GattCharacteristic* getCharacteristicFromHandle(uint16_t charHandle); 00089 void HCIDataWrittenEvent(const GattWriteCallbackParams *params); 00090 void HCIDataReadEvent(const GattReadCallbackParams *params); 00091 void HCIEvent(GattServerEvents::gattEvent_e type, uint16_t charHandle); 00092 void HCIDataSentEvent(unsigned count); 00093 00094 private: 00095 static const int MAX_SERVICE_COUNT = 10; 00096 uint8_t serviceCount; 00097 uint8_t characteristicCount; 00098 uint16_t servHandle, charHandle; 00099 00100 std::map<uint16_t, uint16_t> bleCharHanldeMap; // 1st argument is characteristic, 2nd argument is service 00101 GattCharacteristic *p_characteristics[BLE_TOTAL_CHARACTERISTICS]; 00102 uint16_t bleCharacteristicHandles[BLE_TOTAL_CHARACTERISTICS]; 00103 00104 BlueNRGGattServer() { 00105 serviceCount = 0; 00106 characteristicCount = 0; 00107 }; 00108 00109 BlueNRGGattServer(BlueNRGGattServer const &); 00110 void operator=(BlueNRGGattServer const &); 00111 00112 static const int CHAR_DESC_TYPE_16_BIT=0x01; 00113 static const int CHAR_DESC_TYPE_128_BIT=0x02; 00114 static const int CHAR_DESC_SECURITY_PERMISSION=0x00; 00115 static const int CHAR_DESC_ACCESS_PERMISSION=0x03; 00116 static const int CHAR_ATTRIBUTE_LEN_IS_FIXED=0x00; 00117 }; 00118 00119 #endif
Generated on Tue Jul 12 2022 19:27:28 by
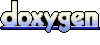