
I am no longer actively working on the ppCANOpen library, however, I want to publish this project so that anyone who wants to pick up any of the pieces can have a good example. This is a a project I was working on using the ppCANOpen library. It has a pretty in deep use of the object dictionary structure. And a number of functions to control high voltage pinball drivers, if you're into that sort of thing.
Dependencies: CANnucleo mbed ppCANOpen
SerialBuffered.cpp
00001 #include "mbed.h" 00002 #include "SerialBuffered.h" 00003 00004 /** 00005 * Create a buffered serial class. 00006 * 00007 * @param tx A pin for transmit. 00008 * @param rx A pin for receive. 00009 */ 00010 SerialBuffered::SerialBuffered(PinName tx, PinName rx) : Serial(tx, rx) { 00011 indexContentStart = 0; 00012 indexContentEnd = 0; 00013 timeout = 1; 00014 attach(this, &SerialBuffered::handleInterrupt); 00015 } 00016 00017 /** 00018 * Destroy. 00019 */ 00020 SerialBuffered::~SerialBuffered() { 00021 } 00022 00023 /** 00024 * Set timeout for getc(). 00025 * 00026 * @param ms milliseconds. (-1:Disable timeout) 00027 */ 00028 void SerialBuffered::setTimeout(int ms) { 00029 timeout = ms; 00030 } 00031 00032 /** 00033 * Read requested bytes. 00034 * 00035 * @param bytes A pointer to a buffer. 00036 * @param requested Length. 00037 * 00038 * @return Readed byte length. 00039 */ 00040 size_t SerialBuffered::readBytes(uint8_t *bytes, size_t requested) { 00041 int i = 0; 00042 while (i < requested) { 00043 int c = getc(); 00044 if (c < 0) { 00045 break; 00046 } 00047 bytes[i] = c; 00048 i++; 00049 } 00050 return i; 00051 } 00052 00053 /** 00054 * Get a character. 00055 * 00056 * @return A character. (-1:timeout) 00057 */ 00058 int SerialBuffered::getc() { 00059 timer.reset(); 00060 timer.start(); 00061 while (indexContentStart == indexContentEnd) { 00062 wait_ms(1); 00063 if ((timeout > 0) && (timer.read_ms() > timeout)) { 00064 /* 00065 * Timeout occured. 00066 */ 00067 // printf("Timeout occured.\n"); 00068 return EOF; 00069 } 00070 } 00071 timer.stop(); 00072 00073 uint8_t result = buffer[indexContentStart++]; 00074 indexContentStart = indexContentStart % BUFFERSIZE; 00075 00076 return result; 00077 } 00078 00079 /** 00080 * Returns 1 if there is a character available to read, 0 otherwise. 00081 */ 00082 int SerialBuffered::readable() { 00083 return indexContentStart != indexContentEnd; 00084 } 00085 00086 void SerialBuffered::handleInterrupt() { 00087 while (Serial::readable()) { 00088 if (indexContentStart == ((indexContentEnd + 1) % BUFFERSIZE)) { 00089 /* 00090 * Buffer overrun occured. 00091 */ 00092 // printf("Buffer overrun occured.\n"); 00093 Serial::getc(); 00094 } else { 00095 buffer[indexContentEnd++] = Serial::getc(); 00096 indexContentEnd = indexContentEnd % BUFFERSIZE; 00097 } 00098 } 00099 }
Generated on Thu Jul 14 2022 21:26:01 by
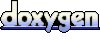