
I am no longer actively working on the ppCANOpen library, however, I want to publish this project so that anyone who wants to pick up any of the pieces can have a good example. This is a a project I was working on using the ppCANOpen library. It has a pretty in deep use of the object dictionary structure. And a number of functions to control high voltage pinball drivers, if you're into that sort of thing.
Dependencies: CANnucleo mbed ppCANOpen
Node_pin0808.h
00001 /** 00002 ****************************************************************************** 00003 * @file 00004 * @author Paul Paterson 00005 * @version 00006 * @date 2015-12-14 00007 * @brief CANOpen implementation library 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 Paul Paterson 00012 * 00013 * All rights reserved. 00014 00015 This program is free software: you can redistribute it and/or modify 00016 it under the terms of the GNU General Public License as published by 00017 the Free Software Foundation, either version 3 of the License, or 00018 (at your option) any later version. 00019 00020 This program is distributed in the hope that it will be useful, 00021 but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00023 GNU General Public License for more details. 00024 00025 You should have received a copy of the GNU General Public License 00026 along with this program. If not, see <http://www.gnu.org/licenses/>. 00027 */ 00028 00029 #ifndef PPCAN_NODE_PIN0808_H 00030 #define PPCAN_NODE_PIN0808_H 00031 00032 #include "Node.h" 00033 00034 namespace ppCANOpen 00035 { 00036 00037 /** Custom Object Dictionary for pinball io_0808 device. 00038 */ 00039 class Node_pin0808 : public Node 00040 { 00041 00042 public: 00043 Node_pin0808(int id, ServiceProvider * provider, int bLoop = 0); 00044 virtual ~Node_pin0808(void); 00045 00046 private: 00047 /* constants */ 00048 /** milliseconds necessary to debounce all input */ 00049 static const uint8_t DEBOUNCE_TIME = 3; 00050 00051 /* IO details */ 00052 uint8_t prevInputBuffers[1]; 00053 uint8_t inputDebounce[8]; 00054 00055 uint8_t prevOutputBuffers[1]; 00056 int scheduleIndexTime; 00057 00058 /************************************************************************** 00059 * Implementation Overrides 00060 ************************************************************************** 00061 */ 00062 00063 /* Application run */ 00064 virtual void OnFixedUpdate(void); 00065 virtual void OnUpdate(void); 00066 00067 /* SYNC */ 00068 virtual void OnSync (uint8_t counter); 00069 00070 /* NMT Control */ 00071 virtual void OnInitialize (void); 00072 virtual void OnPreoperational (void); 00073 virtual void OnOperational (void); 00074 virtual void OnStopped (void); 00075 00076 00077 /************************************************************************** 00078 * Object Dictionary 00079 ************************************************************************** 00080 */ 00081 00082 /* Communication --------------------------------------------------------*/ 00083 /* index 0x1200 : SDO Server */ 00084 ObjectData Obj1200; 00085 SubIndexSize Obj1200_highestSubIndex; 00086 uint32_t Obj1200_ReceiveCobId; 00087 uint32_t Obj1200_TransmitCobId; 00088 EntryData Obj1200_entries[3]; 00089 00090 /* index 0x1400 : Receive PDO 1 Parameter (Write Digital Output) */ 00091 ObjectData Obj1400; 00092 SubIndexSize Obj1400_highestSubIndex; 00093 uint32_t Obj1400_CobId; 00094 uint8_t Obj1400_TransmissionType; 00095 uint16_t Obj1400_InhibitTime; 00096 uint8_t Obj1400_CompatibilityEntry; 00097 uint16_t Obj1400_EventTimer; 00098 EntryData Obj1400_entries[6]; 00099 00100 /* index 0x1401 : Receive PDO 2 Parameter (Write Output Configuration) */ 00101 ObjectData Obj1401; 00102 SubIndexSize Obj1401_highestSubIndex; 00103 uint32_t Obj1401_CobId; 00104 uint8_t Obj1401_TransmissionType; 00105 uint16_t Obj1401_InhibitTime; 00106 uint8_t Obj1401_CompatibilityEntry; 00107 uint16_t Obj1401_EventTimer; 00108 EntryData Obj1401_entries[6]; 00109 00110 /* index 0x1402 : Receive PDO 3 Parameter (Write/Clear Autotrigger Rule) */ 00111 ObjectData Obj1402; 00112 SubIndexSize Obj1402_highestSubIndex; 00113 uint32_t Obj1402_CobId; 00114 uint8_t Obj1402_TransmissionType; 00115 uint16_t Obj1402_InhibitTime; 00116 uint8_t Obj1402_CompatibilityEntry; 00117 uint16_t Obj1402_EventTimer; 00118 EntryData Obj1402_entries[6]; 00119 00120 /* index 0x1403 : Receive PDO 5 Parameter (input changes) */ 00121 ObjectData Obj1403; 00122 SubIndexSize Obj1403_highestSubIndex; 00123 uint32_t Obj1403_CobId; 00124 uint8_t Obj1403_TransmissionType; 00125 uint16_t Obj1403_InhibitTime; 00126 uint8_t Obj1403_CompatibilityEntry; 00127 uint16_t Obj1403_EventTimer; 00128 EntryData Obj1403_entries[6]; 00129 00130 /* index 0x1600 : Receive PDO 1 Mapping */ 00131 ObjectData Obj1600; 00132 SubIndexSize Obj1600_highestSubIndex; 00133 uint32_t Obj1600_Map; 00134 EntryData Obj1600_entries[2]; 00135 00136 /* index 0x1601 : Transmit PDO 1 Mapping */ 00137 ObjectData Obj1601; 00138 SubIndexSize Obj1601_highestSubIndex; 00139 uint32_t Obj1601_Map; 00140 EntryData Obj1601_entries[2]; 00141 00142 /* index 0x1602 : Transmit PDO 1 Mapping */ 00143 ObjectData Obj1602; 00144 SubIndexSize Obj1602_highestSubIndex; 00145 uint32_t Obj1602_Map; 00146 EntryData Obj1602_entries[2]; 00147 00148 /* index 0x1603 : Receive PDO 2 Mapping */ 00149 ObjectData Obj1603; 00150 SubIndexSize Obj1603_highestSubIndex; 00151 uint32_t Obj1603_Map; 00152 EntryData Obj1603_entries[2]; 00153 00154 00155 /* index 0x1800 : Transmit PDO 1 Parameter */ 00156 ObjectData Obj1800; 00157 SubIndexSize Obj1800_highestSubIndex; 00158 uint32_t Obj1800_CobId; 00159 uint8_t Obj1800_TransmissionType; 00160 uint16_t Obj1800_InhibitTime; 00161 uint8_t Obj1800_CompatibilityEntry; 00162 uint16_t Obj1800_EventTimer; 00163 EntryData Obj1800_entries[6]; 00164 00165 /* index 0x1A00 : Transmit PDO 1 Mapping */ 00166 ObjectData Obj1A00; 00167 SubIndexSize Obj1A00_highestSubIndex; 00168 uint32_t Obj1A00_MapInput; 00169 uint32_t Obj1A00_MapVoid16; 00170 uint32_t Obj1A00_MapChange; 00171 uint32_t Obj1A00_MapSourceId; 00172 EntryData Obj1A00_entries[6]; 00173 00174 00175 /* Manufacturer Specific ------------------------------------------------*/ 00176 /* index 0x2001 : Pin Output Configurations */ 00177 struct OutputConfiguration { 00178 static const uint8_t PULSE = 0x01; 00179 static const uint8_t PATTER = 0x02; 00180 static const uint8_t PULSED_PATTER = 0x04; 00181 //static const uint8_t SCHEDULED = 0x08; 00182 00183 uint8_t type; 00184 uint8_t pulse_ms; 00185 uint8_t pwm_on; 00186 uint8_t pwm_off; 00187 uint32_t writeData; 00188 }; 00189 00190 ObjectData Obj2001; 00191 SubIndexSize Obj2001_highestSubIndex; 00192 OutputConfiguration writeOutputConfig; 00193 OutputConfiguration outputConfigs[8]; 00194 OutputConfiguration outputTimers[8]; /* not actually part of dictionary */ 00195 EntryData Obj2001_entries[10]; 00196 00197 /* index 0x2002 : Output Schedule Configuration */ 00198 ObjectData Obj2002; 00199 SubIndexSize Obj2002_highestSubIndex; 00200 uint32_t scheduleConfig; 00201 uint32_t schedules[8]; 00202 EntryData Obj2002_entries[10]; 00203 00204 /* index 0x2100 : Input change data */ 00205 ObjectData Obj2100; 00206 SubIndexSize Obj2100_highestSubIndex; 00207 uint8_t inputChangeMask[1]; 00208 /* uint8_t nodeId; */ 00209 EntryData Obj2100_entries[3]; 00210 00211 /* index 0x2200 : Autotrigger Rules */ 00212 struct AutotriggerRule { 00213 uint8_t sourceId; 00214 uint8_t input; /* 0-4: inputNum (0-23) 00215 5-7: 0 on inactive 00216 1 on active 00217 2 on any change 00218 3 ??? 00219 */ 00220 uint8_t setMask[3]; 00221 uint8_t clearMask[3]; 00222 }; 00223 00224 struct AutotriggerMessage { 00225 uint8_t input[3]; 00226 uint8_t change[3]; 00227 uint8_t sourceId; 00228 }; 00229 00230 ObjectData Obj2200; 00231 SubIndexSize Obj2200_highestSubIndex; 00232 AutotriggerRule writeRule; 00233 AutotriggerMessage autotriggerMessage; 00234 AutotriggerRule rules[24]; 00235 EntryData Obj2200_entries[27]; 00236 00237 00238 /* Device Specific ------------------------------------------------------*/ 00239 /* index 0x6000 : Mapped variable "inputs" */ 00240 ObjectData Obj6000; 00241 SubIndexSize Obj6000_highestSubIndex; 00242 uint8_t readInputBuffers[1]; 00243 EntryData Obj6000_entries[2]; 00244 00245 /* index 0x6005 : Global Input Interrupt Enable */ 00246 ObjectData Obj6005; 00247 SubIndexSize Obj6005_highestSubIndex; 00248 uint8_t bInputInterruptEnable; 00249 EntryData Obj6005_entries[2]; 00250 00251 /* index 0x6006 : Any Change Interrupt Mask */ 00252 ObjectData Obj6006; 00253 SubIndexSize Obj6006_highestSubIndex; 00254 uint8_t inputInterruptMask[1]; 00255 EntryData Obj6006_entries[2]; 00256 00257 /* index 0x6200 : Mapped variable "outputs" */ 00258 ObjectData Obj6200; 00259 SubIndexSize Obj6200_highestSubIndex; 00260 uint8_t writeOutputBuffers[1]; 00261 EntryData Obj6200_entries[2]; 00262 00263 /* Scan Method */ 00264 virtual ObjectData * ScanIndex(IndexSize index); 00265 }; 00266 00267 } /* namespace ppCANOpen */ 00268 00269 #endif // PPCAN_NODE_PIN0808_H
Generated on Thu Jul 14 2022 21:26:01 by
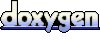