library for C++ CANOpen implementation. mbed independant, but is easy to attach into with mbed.
Dependents: ppCANOpen_Example DISCO-F746NG_rtos_test
CanOpenMessage.h
00001 /** 00002 ****************************************************************************** 00003 * @file 00004 * @author Paul Paterson 00005 * @version 00006 * @date 2015-12-14 00007 * @brief CANOpen implementation library 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2015 Paul Paterson 00012 * 00013 * All rights reserved. 00014 00015 This program is free software: you can redistribute it and/or modify 00016 it under the terms of the GNU General Public License as published by 00017 the Free Software Foundation, either version 3 of the License, or 00018 (at your option) any later version. 00019 00020 This program is distributed in the hope that it will be useful, 00021 but WITHOUT ANY WARRANTY; without even the implied warranty of 00022 MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00023 GNU General Public License for more details. 00024 00025 You should have received a copy of the GNU General Public License 00026 along with this program. If not, see <http://www.gnu.org/licenses/>. 00027 */ 00028 00029 #ifndef PPCAN_CANOPEN_MESSAGE_H 00030 #define PPCAN_CANOPEN_MESSAGE_H 00031 00032 #include "stdint.h" 00033 00034 #ifdef __cplusplus 00035 extern "C" { 00036 #endif 00037 00038 /*========================================================================= 00039 * CANOpen Message Structure 00040 *========================================================================= 00041 */ 00042 00043 /** CANOpen Message Format */ 00044 typedef enum { 00045 CANOPEN_FORMAT_STANDARD = 0, 00046 CANOPEN_FORMAT_EXTENDED, 00047 CANOPEN_FORMAT_ANY 00048 } CanOpenFormat; 00049 00050 /** CANOpen Message Data Type */ 00051 typedef enum { 00052 CANOPEN_TYPE_DATA = 0, 00053 CANOPEN_TYPE_REMOTE 00054 } CanOpenType; 00055 00056 00057 /** CANOpen Message */ 00058 typedef struct CanOpenMessage { 00059 uint32_t id; 00060 uint8_t data[8]; 00061 CanOpenFormat format; 00062 CanOpenType type; 00063 uint8_t dataCount; 00064 } CanOpenMessage; 00065 00066 00067 /*========================================================================= 00068 * CANOpen Function Codes 00069 *========================================================================= 00070 */ 00071 00072 /** CANOpen Function Codes */ 00073 typedef enum { 00074 CANOPEN_FUNCTION_CODE_NMT = 0x00, 00075 CANOPEN_FUNCTION_CODE_SYNC = 0x01, 00076 CANOPEN_FUNCTION_CODE_TIME = 0x02, 00077 CANOPEN_FUNCTION_CODE_PDO1T = 0x03, 00078 CANOPEN_FUNCTION_CODE_PDO1R = 0x04, 00079 CANOPEN_FUNCTION_CODE_PDO2T = 0x05, 00080 CANOPEN_FUNCTION_CODE_PDO2R = 0x06, 00081 CANOPEN_FUNCTION_CODE_PDO3T = 0x07, 00082 CANOPEN_FUNCTION_CODE_PDO3R = 0x08, 00083 CANOPEN_FUNCTION_CODE_PDO4T = 0x09, 00084 CANOPEN_FUNCTION_CODE_PDO4R = 0x0A, 00085 CANOPEN_FUNCTION_CODE_SDOT = 0x0B, 00086 CANOPEN_FUNCTION_CODE_SDOR = 0x0C, 00087 CANOPEN_FUNCTION_CODE_NODE_GUARD = 0x0E, 00088 CANOPEN_FUNCTION_CODE_LSS = 0x0F 00089 } CanOpenFunctionCodes; 00090 00091 /* Message Constants */ 00092 #define MESSAGE_NODEID_BITS 0b00001111111 00093 #define MESSAGE_COMMAND_BITS 0b11110000000 00094 00095 /* Message Macros -----------------------------------------------------------*/ 00096 #define MESSAGE_GET_NODEID(cobId) (cobId & MESSAGE_NODEID_BITS) 00097 #define MESSAGE_GET_COMMAND(cobId) ((cobId & MESSAGE_COMMAND_BITS) >> 7) 00098 00099 /*========================================================================= 00100 * SDO MESSAGE PARAMETERS 00101 *========================================================================= 00102 */ 00103 00104 /** SDO initiate protocol command specifiers */ 00105 typedef enum { 00106 SDO_CCS_DOWNLOAD_SEGMENT_REQUEST = 0x00, 00107 SDO_CCS_INITIATE_DOWNLOAD_REQUEST = 0x01, 00108 SDO_CCS_INITIATE_UPLOAD_REQUEST = 0x02, 00109 } SdoClientCommandSpecifier; 00110 00111 /** SDO segment protocol command specifiers */ 00112 typedef enum { 00113 SDO_SCS_DOWNLOAD_SEGMENT_RESPONSE = 0x01, 00114 SDO_SCS_INITIATE_UPLOAD_RESPONSE = 0x02, 00115 SDO_SCS_INITIATE_DOWNLOAD_RESPONSE = 0x03, 00116 } SdoServerCommandSpecifier; 00117 00118 /* SDO constants --------------------------------------------------------*/ 00119 #define SDO_SIZE_INDICATOR_BIT 0b00000001 00120 #define SDO_TRANSFER_TYPE_BIT 0b00000010 00121 #define SDO_DATA_COUNT_BITS 0b00001100 00122 #define SDO_TOGGLE_BIT 0b00010000 00123 #define SDO_CS_BITS 0b11100000 00124 00125 /* SDO macros -----------------------------------------------------------*/ 00126 #define SDO_GET_CS(data0) ((data0 & SDO_CS_BITS) >> 5) 00127 #define SDO_GET_DATA_COUNT(data0) ((data0 & SDO_DATA_COUNT_BITS) >> 2) 00128 00129 00130 /*========================================================================= 00131 * NMT MESSAGE PARAMETERS 00132 *========================================================================= 00133 */ 00134 00135 /** NMT node control protocol command specifiers */ 00136 typedef enum { 00137 NMT_CS_START = 0x01, 00138 NMT_CS_STOP = 0x02, 00139 NMT_CS_ENTER_PREOP = 0x80, 00140 NMT_CS_RESET_NODE = 0x81, 00141 NMT_CS_RESET_COM = 0x82 00142 } NmtCommandSpecifier; 00143 00144 #ifdef __cplusplus 00145 }; 00146 #endif 00147 00148 #endif /* PPCAN_CANOPEN_MESSAGE_H */
Generated on Sun Jul 17 2022 07:51:19 by
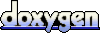