Dependents: Telecommande_prologue
TFT_4DGL_Touch.cpp
00001 // 00002 // TFT_4DGL is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // TFT_4DGL is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // TFT_4DGL is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with TFT_4DGL. If not, see <http://www.gnu.org/licenses/>. 00018 00019 #include "mbed.h" 00020 #include "TFT_4DGL.h" 00021 00022 //****************************************************************************************************** 00023 void TFT_4DGL :: touch_mode(char mode) { // Send touch mode (WAIT, PRESS, RELEASE or MOVE) 00024 00025 char command[2]= ""; 00026 00027 command[0] = GETTOUCH; 00028 command[1] = mode; 00029 00030 writeCOMMAND(command, 2); 00031 } 00032 00033 //****************************************************************************************************** 00034 void TFT_4DGL :: get_touch(int *x, int *y) { // Get the touch coordinates 00035 00036 char command[2] = ""; 00037 00038 command[0] = GETTOUCH; 00039 command[1] = GETPOSITION; 00040 00041 getTOUCH(command, 2, x, y); 00042 } 00043 00044 //****************************************************************************************************** 00045 int TFT_4DGL :: touch_status(void) { // Get the touch screen status 00046 00047 char command[2] = ""; 00048 00049 command[0] = GETTOUCH; 00050 command[1] = STATUS; 00051 00052 return getSTATUS(command, 2); 00053 } 00054 00055 00056 //****************************************************************************************************** 00057 void TFT_4DGL :: wait_touch(int delay) { // wait until touch within a delay in milliseconds 00058 00059 char command[3]= ""; 00060 00061 command[0] = WAITTOUCH; 00062 00063 command[1] = (delay >> 8) & 0xFF; 00064 command[2] = delay & 0xFF; 00065 00066 writeCOMMAND(command, 3); 00067 } 00068 00069 //****************************************************************************************************** 00070 void TFT_4DGL :: set_touch(int x1, int y1 , int x2, int y2) { // define touch area 00071 00072 char command[9]= ""; 00073 00074 command[0] = SETTOUCH; 00075 00076 command[1] = (x1 >> 8) & 0xFF; 00077 command[2] = x1 & 0xFF; 00078 00079 command[3] = (y1 >> 8) & 0xFF; 00080 command[4] = y1 & 0xFF; 00081 00082 command[5] = (x2 >> 8) & 0xFF; 00083 command[6] = x2 & 0xFF; 00084 00085 command[7] = (y2 >> 8) & 0xFF; 00086 command[8] = y2 & 0xFF; 00087 00088 writeCOMMAND(command, 9); 00089 } 00090 00091 void TFT_4DGL :: image_touch( int x, int y, int width, int height, long sector, TFT_4DGL Screen){ 00092 00093 char command[1000]= ""; 00094 00095 command[0] = TEXTBUTTON; 00096 00097 command[1] = UP; 00098 00099 command[2] = (x >> 8) & 0xFF; 00100 command[3] = x & 0xFF; 00101 00102 command[4] = (y >> 8) & 0xFF; 00103 command[5] = y & 0xFF; 00104 00105 00106 command[6] = TRANSPARENT; 00107 command[7] = TRANSPARENT; 00108 00109 command[8] = TRANSPARENT; 00110 00111 00112 command[9] = TRANSPARENT; 00113 command[10] = TRANSPARENT; 00114 00115 command[11] = width; 00116 00117 command[12] = height; 00118 00119 command[13] = NULL; 00120 00121 Screen.uSD_Image(x, y, sector); 00122 writeCOMMAND(command, 14); 00123 }
Generated on Tue Jul 26 2022 21:37:35 by
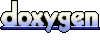