Dependents: Telecommande_prologue
TFT_4DGL.h
00001 // 00002 // TFT_4DGL is a class to drive 4D Systems TFT touch screens 00003 // 00004 // Copyright (C) <2010> Stephane ROCHON <stephane.rochon at free.fr> 00005 // 00006 // TFT_4DGL is free software: you can redistribute it and/or modify 00007 // it under the terms of the GNU General Public License as published by 00008 // the Free Software Foundation, either version 3 of the License, or 00009 // (at your option) any later version. 00010 // 00011 // TFT_4DGL is distributed in the hope that it will be useful, 00012 // but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 // MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 // GNU General Public License for more details. 00015 // 00016 // You should have received a copy of the GNU General Public License 00017 // along with TFT_4DGL. If not, see <http://www.gnu.org/licenses/>. 00018 00019 // @author Stephane Rochon 00020 00021 #include "mbed.h" 00022 00023 // Debug Verbose on terminal enabled 00024 #ifndef DEBUGMODE 00025 #define DEBUGMODE 1 00026 #endif 00027 00028 // Common WAIT value in millisecond 00029 #define TEMPO 5 00030 00031 // 4DGL Functions values 00032 #define AUTOBAUD '\x55' 00033 #define CLS '\x45' 00034 #define BAUDRATE '\x51' 00035 #define VERSION '\x56' 00036 #define BCKGDCOLOR '\x42' 00037 #define DISPCONTROL '\x59' 00038 #define SETVOLUME '\x76' 00039 #define CIRCLE '\x43' 00040 #define TRIANGLE '\x47' 00041 #define LINE '\x4C' 00042 #define RECTANGLE '\x72' 00043 #define ELLIPSE '\x65' 00044 #define PIXEL '\x50' 00045 #define READPIXEL '\x52' 00046 #define SCREENCOPY '\x63' 00047 #define PENSIZE '\x70' 00048 #define SETFONT '\x46' 00049 #define TEXTMODE '\x4F' 00050 #define TEXTCHAR '\x54' 00051 #define GRAPHCHAR '\x74' 00052 #define TEXTSTRING '\x73' 00053 #define GRAPHSTRING '\x53' 00054 #define TEXTBUTTON '\x62' 00055 #define GETTOUCH '\x6F' 00056 #define WAITTOUCH '\x77' 00057 #define SETTOUCH '\x75' 00058 00059 00060 // Screen answers 00061 #define ACK '\x06' 00062 #define NAK '\x15' 00063 00064 // Screen states 00065 #define OFF '\x00' 00066 #define ON '\x01' 00067 00068 // Graphics modes 00069 #define SOLID '\x00' 00070 #define WIREFRAME '\x01' 00071 00072 // Text modes 00073 #define TRANSPARENT '\x00' 00074 #define OPAQUE '\x01' 00075 00076 // Fonts Sizes 00077 #define FONT_5X7 '\x00' 00078 #define FONT_8X8 '\x01' 00079 #define FONT_8X12 '\x02' 00080 #define FONT_12X16 '\x03' 00081 00082 // Touch Values 00083 #define WAIT '\x00' 00084 #define PRESS '\x01' 00085 #define RELEASE '\x02' 00086 #define MOVE '\x03' 00087 #define STATUS '\x04' 00088 #define GETPOSITION '\x05' 00089 00090 // Data speed 00091 #define BAUD_110 '\x00' 00092 #define BAUD_300 '\x01' 00093 #define BAUD_600 '\x02' 00094 #define BAUD_1200 '\x03' 00095 #define BAUD_2400 '\x04' 00096 #define BAUD_4800 '\x05' 00097 #define BAUD_9600 '\x06' 00098 #define BAUD_14400 '\x07' 00099 #define BAUD_19200 '\x09' 00100 #define BAUD_31250 '\x09' 00101 #define BAUD_38400 '\x0A' 00102 #define BAUD_56000 '\x0B' 00103 #define BAUD_57600 '\x0C' 00104 #define BAUD_115200 '\x0D' 00105 #define BAUD_128000 '\x0E' 00106 #define BAUD_256000 '\x0F' 00107 00108 // Defined Colors 00109 #define WHITE 0xFFFFFF 00110 #define BLACK 0x000000 00111 #define RED 0xFF0000 00112 #define GREEN 0x00FF00 00113 #define BLUE 0x0000FF 00114 #define LGREY 0xBFBFBF 00115 #define DGREY 0x5F5F5F 00116 00117 // Mode data 00118 #define BACKLIGHT '\x00' 00119 #define DISPLAY '\x01' 00120 #define CONTRAST '\x02' 00121 #define POWER '\x03' 00122 #define ORIENTATION '\x04' 00123 #define TOUCH_CTRL '\x05' 00124 #define IMAGE_FORMAT '\x06' 00125 #define PROTECT_FAT '\x08' 00126 00127 // change this to your specific screen (newer versions) if needed 00128 // Startup orientation is PORTRAIT so SIZE_X must be lesser than SIZE_Y 00129 #define SIZE_X 240 00130 #define SIZE_Y 320 00131 00132 #define IS_LANDSCAPE 0 00133 #define IS_PORTRAIT 1 00134 00135 // Screen orientation 00136 #define LANDSCAPE '\x01' 00137 #define LANDSCAPE_R '\x02' 00138 #define PORTRAIT '\x03' 00139 #define PORTRAIT_R '\x04' 00140 00141 // Parameters 00142 #define ENABLE '\x00' 00143 #define DISABLE '\x01' 00144 #define RESET '\x02' 00145 00146 #define NEW '\x00' 00147 #define OLD '\x01' 00148 00149 #define DOWN '\x00' 00150 #define UP '\x01' 00151 00152 #define PROTECT '\x00' 00153 #define UNPROTECT '\x02' 00154 00155 //************************************************************************** 00156 // \class TFT_4DGL TFT_4DGL.h 00157 // \brief This is the main class. It shoud be used like this : TFT_4GDL myLCD(p9,p10,p11); 00158 /** 00159 Example: 00160 * @code 00161 * // Display a white circle on the screen 00162 * #include "mbed.h" 00163 * #include " TFT_4DGL.h" 00164 * 00165 * TFT_4GDL myLCD(p9,p10,p11); 00166 * 00167 * int main() { 00168 * myLCD.circle(120, 160, 80, WHITE); 00169 * } 00170 * @endcode 00171 */ 00172 00173 class TFT_4DGL { 00174 00175 public : 00176 00177 TFT_4DGL(PinName tx, PinName rx, PinName rst); 00178 00179 // General Commands ******************************************************************************* 00180 00181 /** Clear the entire screen using the current background colour */ 00182 void cls(); 00183 00184 /** Reset screen */ 00185 void reset(); 00186 00187 /** Launch Autobaud for serial communication. This function is automatically called at startup */ 00188 void autobaud(); 00189 /** Set serial Baud rate (both sides : screen and mbed) 00190 * @param Speed Correct BAUD value (see TFT_4DGL.h) 00191 */ 00192 void baudrate(int speed); 00193 00194 /** Set background colour to the specified value 00195 * @param color in HEX RGB like 0xFF00FF 00196 */ 00197 void background_color(int color); 00198 00199 /** Set screen display mode to specific values 00200 * @param mode See 4DGL documentation 00201 * @param value See 4DGL documentation 00202 */ 00203 void display_control(char mode, char value); 00204 00205 /** Set internal speaker to specified value 00206 * @param value Correct range is 8 - 127 00207 */ 00208 void set_volume(char value); 00209 00210 // Graphics Commands ******************************************************************************* 00211 00212 /** Draw a circle centered at x,y with a radius and a colour. It uses Pen Size stored value to draw a solid or wireframe circle 00213 * @param x Horizontal position of the circle centre 00214 * @param y Vertical position of the circle centre 00215 * @param radius Radius of the circle 00216 * @param color Circle color in HEX RGB like 0xFF00FF 00217 */ 00218 void circle(int x , int y , int radius, int color); 00219 00220 void triangle(int, int, int, int, int, int, int); 00221 void line(int, int, int, int, int); 00222 void rectangle(int, int, int, int, int); 00223 void ellipse(int, int, int, int, int); 00224 void pixel(int, int, int); 00225 int read_pixel(int, int); 00226 void screen_copy(int, int, int, int, int, int); 00227 void pen_size(char); 00228 void SD_Card_Wav(char[]); 00229 void Set_Volume(char); 00230 void uSD_FAT_Image(char[], int, int, long); 00231 void uSD_Image(int, int, long); 00232 void uSD_Video(int, int, long); 00233 00234 // Texts Commands 00235 void set_font(char); 00236 void text_mode(char); 00237 void text_char(char, char, char, int); 00238 void graphic_char(char, int, int, int, char, char); 00239 void text_string(char *, char, char, char, int); 00240 void graphic_string(char *, int, int, char, int, char, char); 00241 void text_button(char *, char, int, int, int, char, int, char, char); 00242 00243 void locate(char, char); 00244 void color(int); 00245 void putc(char); 00246 void puts(char *); 00247 00248 // Touch Command 00249 void touch_mode(char); 00250 void get_touch(int *, int *); 00251 void wait_touch(int); 00252 void set_touch(int, int, int, int); 00253 int touch_status(void); 00254 void image_touch( int x, int y, int width, int height, long sector, TFT_4DGL Screen); 00255 00256 // Screen Data 00257 int type; 00258 int revision; 00259 int firmware; 00260 int reserved1; 00261 int reserved2; 00262 00263 // Text data 00264 char current_col; 00265 char current_row; 00266 int current_color; 00267 char current_font; 00268 char current_orientation; 00269 char max_col; 00270 char max_row; 00271 00272 protected : 00273 00274 Serial _cmd; 00275 DigitalOut _rst; 00276 00277 void freeBUFFER (void); 00278 void writeBYTE (char); 00279 int writeCOMMAND(char *, int); 00280 int readVERSION (char *, int); 00281 void getTOUCH (char *, int, int *,int *); 00282 int getSTATUS (char *, int); 00283 void version (void); 00284 }; 00285 00286 typedef unsigned char BYTE;
Generated on Tue Jul 26 2022 21:37:35 by
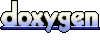