Smart coffee machine with facial recognition and remote control
Dependencies: Camera_LS_Y201 EthernetInterface EthernetNetIf HTTPClient SRF05 TextLCD mbed-rtos mbed-src
camera.cpp
00001 #include "camera.h" 00002 00003 LocalFileSystem local("local"); 00004 Camera_LS_Y201 camera(p13, p14); 00005 00006 typedef struct work 00007 { 00008 FILE *fp; 00009 } work_t; 00010 00011 work_t work; 00012 00013 void acallback_func(int done, int total, uint8_t *buf, size_t siz, char *reponse) 00014 { 00015 // Fonction de callback pour écriture de l'image 00016 // buf : pointeur sur un buffer 00017 // siz : taille tu buffer 00018 //printf("callback : buffer de taille %d\r\n", siz); 00019 //fwrite(buf, siz, 1, work.fp); 00020 00021 00022 envoyerChaineSocket((char*)buf, siz, reponse, 30); 00023 //printf("callback response : %s\r\n", reponse); 00024 00025 00026 //static int n = 0; 00027 //int tmp = done * 100 / total; 00028 00029 //if(n != tmp) 00030 //{ 00031 // n = tmp; 00032 // printf("Writing...: %3d%%\r\n", n); 00033 //} 00034 } 00035 00036 int acapture(Camera_LS_Y201 *cam, char *reponse) 00037 { 00038 // Prise de l'image 00039 if(cam->takePicture() != 0) 00040 return -1; 00041 00042 printf("\tPhoto prise\r\n"); 00043 //afficherAuCentreDeLEcran("Photo prise", "wesh"); 00044 00045 // Ouverture du fichier 00046 //work.fp = fopen(nom_fichier, "wb"); 00047 //if(work.fp == NULL) 00048 // return -2; 00049 00050 // Lecture du contenu 00051 //printf("%s\r\n", nom_fichier); 00052 00053 if(cam->readJpegFileContent(acallback_func, envoyerChaineSocket, reponse) != 0) 00054 { 00055 //fclose(work.fp); 00056 return -3; 00057 } 00058 00059 //fclose(work.fp); 00060 wait(1); 00061 00062 // Fin de la prise d'image 00063 cam->stopTakingPictures(); 00064 00065 return 0; // Retourne donc 0 en cas de succès 00066 } 00067 00068 bool initialiserCamera() 00069 { 00070 int initCam; 00071 00072 do 00073 { 00074 printf("Reset CAMERA\r\n"); 00075 initCam = camera.reset(); 00076 } while(initCam != 0); 00077 00078 if(initCam != 0) 00079 { 00080 printf("Echec de l'initialisation caméra %d\r\n", initCam); 00081 afficherAuCentreDeLEcran("Echec init", "camera"); 00082 return false; 00083 } 00084 00085 else 00086 { 00087 printf("Initialisation caméra OK.\r\n"); 00088 afficherAuCentreDeLEcran("Initialisation", "camera : ok"); 00089 return true; 00090 } 00091 } 00092 00093 bool detecterUtilisateur(bool *utilisateur_reconnu, char* nom_utilisateur, int* preference_intensite, int *preference_longueur) 00094 { 00095 printf("\tDébut détection utilisateur\r\n"); 00096 char reponse[30], requete[10]; 00097 int taille_reponse = 0; 00098 00099 if(connexionSocket()) 00100 { 00101 sprintf(requete,"PHOTO"); 00102 00103 // Envoi de "RECO". Réception de "RECO OK" 00104 envoyerChaineSocket(requete, sizeof(requete), reponse, 30); 00105 //printf("Reponse %s : %s\r\n\r", requete, reponse); 00106 00107 int r = acapture(&camera, reponse); 00108 00109 if(r != 0) 00110 printf("IMG_TEST:NG. (code=%d)\r\n", r); 00111 00112 /*else 00113 printf("IMG_TEST:OK.\r\n");*/ 00114 00115 // Parsing des parametres depuis la réponse 00116 00117 /* CHAINES A RECEVOIR : 00118 USER=Leo 2 3 // cas Léo reconnu 00119 USER=Simon 3 4 // cas Simon reconnu 00120 USER- // cas aucun visage 00121 USER?userTest // cas visage non reconnu 00122 */ 00123 00124 //sprintf(reponse, "USER?userTest"); 00125 //sprintf(reponse, "USER=Simon 3 4"); 00126 printf("Chaine d'identification : [%s]\n\r", reponse); 00127 00128 if(reponse[4] == '-') 00129 { 00130 deconnexionSocket(); 00131 return false; 00132 } 00133 00134 *utilisateur_reconnu = (reponse[4] != '?'); 00135 taille_reponse = strlen(reponse); 00136 00137 int j; 00138 for(j = 5 ; j < taille_reponse && reponse[j] != ' ' ; j++) 00139 nom_utilisateur[j - 5] = reponse[j]; 00140 nom_utilisateur[j - 5] = '\0'; 00141 00142 *preference_intensite = reponse[taille_reponse - 3] - '0'; 00143 *preference_longueur = reponse[taille_reponse - 1] - '0'; 00144 00145 deconnexionSocket(); 00146 return true; 00147 } 00148 00149 else 00150 { 00151 printf("\tErreur d'ouverture du socket\r\n\r"); 00152 return false; 00153 } 00154 }
Generated on Wed Jul 13 2022 03:22:24 by
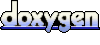