
yuppie2
Dependencies: SDFileSystem mbed
Fork of rtcfinalcodehet by
rtsc.cpp
00001 #include "rtsc.h" 00002 SPI spi(PTD6, PTD7, PTD5); 00003 DigitalOut cs(PTD2); 00004 00005 SDFileSystem sd(PTD6, PTD7, PTD5, D10,"sd"); 00006 00007 void spiwrite(int a) 00008 { 00009 cs=1; 00010 cs=0; 00011 spi.write(a); 00012 spi.write(0x01); 00013 } 00014 00015 int spiread(int a) 00016 { 00017 cs=1; 00018 cs=0; 00019 spi.write(a); 00020 return(spi.write(0x00)); 00021 } 00022 char* getname(int year1,int month1,int date1,int day1,int hours1,int minutes1,int seconds1) 00023 { 00024 year1= hexint(year1); 00025 month1=hexint(month1); 00026 date1=hexint(date1); 00027 day1=hexint(day1); 00028 hours1=hexint(hours1); 00029 minutes1=hexint(minutes1); 00030 seconds1=hexint(seconds1); 00031 char time[15]; 00032 sprintf(time,"%02d%02d%02d%02d%02d%02d%02d",year1,month1,date1,day1,hours1,minutes1,seconds1); 00033 00034 return(time); 00035 00036 } 00037 00038 int hexint(int a) 00039 { 00040 a=(a/16)*10+(a%16); 00041 return a; 00042 } 00043 //storedata stores the dummy structure in the file with timestamp as the filename in HK directory 00044 void storedata(void) 00045 { 00046 spi.format(8,3); 00047 spi.frequency(1000000); 00048 spiwrite(0x80); //write seconds to 01 00049 spiwrite(0x81); //write minutes t0 01 00050 spiwrite(0x82); //write hours to 01 00051 spiwrite(0x83); //write day of week to 01 00052 spiwrite(0x84); //write day of month to 01 00053 spiwrite(0x85); //write month to 01 00054 spiwrite(0x86); //write year to 01 00055 for(int i=0;i<1000000;i++){ 00056 int seconds=spiread(0x00); //read seconds 00057 int minutes =spiread(0x01); //read minutes 00058 int hours =spiread(0x02); //read hours 00059 int day =spi.write(0x03); //read day of the week 00060 int date =spiread(0x04); //read day of the month 00061 int month =spiread(0x05); //read month 00062 int year =spiread(0x06); //read year 00063 cs = 1; 00064 00065 //Assigning dummy values to the structure 00066 00067 SensorData Sensor; 00068 00069 printf("Writing dummy values\n"); 00070 strcpy( Sensor.Voltage, "49"); 00071 strcpy( Sensor.Current, "83"); 00072 Sensor.Temperature ='5'; 00073 strcpy( Sensor.PanelTemperature, "4"); 00074 Sensor.Vcell_soc='9'; 00075 Sensor.alerts= '4'; 00076 Sensor.crate='7'; 00077 Sensor.BatteryTemperature='6'; 00078 Sensor.faultpoll='4'; 00079 Sensor.faultir='g'; 00080 Sensor.power_mode='k'; 00081 strcpy( Sensor.AngularSpeed, "9"); 00082 strcpy(Sensor.Bnewvalue,"6"); 00083 printf("Done writing dummy values\n"); 00084 mkdir("/sd/hk", 0777); 00085 char date2[100]="/sd/hk/"; 00086 strcat(date2,getname(year,month,date,day,hours,minutes,seconds)); 00087 strcat(date2,".txt"); 00088 FILE *fp ; 00089 fp= fopen(date2, "w"); 00090 if(fp == NULL) { 00091 error("Could not open file for write\n"); 00092 } 00093 else 00094 { 00095 fprintf(fp, "%s -", Sensor.Voltage); 00096 fprintf(fp,"%s -",Sensor.Current); 00097 fprintf(fp,"%c -",Sensor.Temperature); 00098 fprintf(fp,"%s -",Sensor.PanelTemperature); 00099 fprintf(fp,"%c -",Sensor.Vcell_soc); //printing the contents of the strucure in a single line in the file 00100 fprintf(fp,"%c -",Sensor.alerts); 00101 fprintf(fp,"%c -",Sensor.crate); 00102 fprintf(fp,"%c -",Sensor.BatteryTemperature); 00103 fprintf(fp,"%c -",Sensor.faultpoll); 00104 fprintf(fp,"%c -",Sensor.faultir); 00105 fprintf(fp,"%c -",Sensor.power_mode); 00106 fprintf(fp,"%s -",Sensor.AngularSpeed); 00107 fprintf(fp,"%s",Sensor.Bnewvalue); 00108 fclose(fp); 00109 printf("%s",getname(year,month,date,day,hours,minutes,seconds)); 00110 wait(10); 00111 00112 00113 } 00114 }}
Generated on Wed Jul 20 2022 01:03:49 by
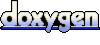