
sd 32 update
Dependencies: FreescaleIAP mbed-rtos mbed
Fork of COM_MNG_TMTC_SIMPLE by
Compression.h
00001 int disk_write(const uint8_t *, uint64_t); 00002 uint64_t RTC_TIME; //need to be changed to uint_64 00003 unsigned char SDcard_lastWritten[512] = {0}; 00004 00005 namespace Science_TMframe { 00006 00007 #define OUTLENGTH 360 //length of the output frame after convolution 00008 #define SDcard_block 512 //block size of the sd card 00009 00010 Convolution ConvObj; //object which stores the frame after convolution 00011 bool fresh[3] = {true,true,true}; // True only for the first time 00012 unsigned char frames[3][134] = {0}; // "frame" stores the address of the current frame...."first_frame_address" stores the address of the first node made. 00013 unsigned int FCN[4] = {0}; //frame count number 00014 unsigned int data_starting_point[3] = {8,5,10}; 00015 unsigned int max_data[3] = {124,127,122}; //number of bytes in each frame excluding TMID,FCN,first_header_point,crc 00016 unsigned char TM_convoluted_data[270] = {0}; //270 bytes is the size after convolution of 1072 bits 00017 unsigned char complete_frame[SDcard_block] = {0}; 00018 uint64_t SDC_address = 200; 00019 bool SCH_FCCH_FLAG = true; 00020 00021 00022 void add_SCH_FCCH(){ 00023 int i = 0; 00024 complete_frame[0] = 0x0a; 00025 complete_frame[1] = 0x3f;; 00026 complete_frame[2] = 0x46; 00027 complete_frame[3] = 0xb4; 00028 complete_frame[4] = 0x00; 00029 00030 for(i = 149 ; i < 159 ; i ++){ 00031 complete_frame[i] = 0 ; 00032 } 00033 00034 complete_frame[159] = 0x0a; 00035 complete_frame[160] = 0x3f;; 00036 complete_frame[161] = 0x46; 00037 complete_frame[162] = 0xb4; 00038 complete_frame[163] = 0x00; 00039 00040 for(i = 308 ; i < 318 ; i ++){ 00041 complete_frame[i] = 0 ; 00042 } 00043 00044 } 00045 00046 void making_frameHeader(unsigned char TMID){ 00047 00048 unsigned char frame_type_identifier = 0; // not conform about the values , yet to be done 00049 frames[TMID][0] = (frame_type_identifier<<7) + ( (TMID + 1)<<3 ) + ( (FCN[TMID]>>24) & 0x7 ); //frame number should be less than 2^23 since 23 bits are assigned for that 00050 frames[TMID][1] = ((FCN[TMID]>>16) & 0xff ); 00051 frames[TMID][2] = ( (FCN[TMID]>>8 )& 0xff ); 00052 frames[TMID][3] = ( FCN[TMID] & 0xff ); // first bit for (frame identifier), next 4 for (TMID) and next 27 for FCN 00053 00054 if(TMID == 0){ 00055 frames[TMID][5] =( (RTC_TIME>>29) & 0xff ); 00056 frames[TMID][6] =( (RTC_TIME>>21) & 0xff ); 00057 frames[TMID][7] =( (RTC_TIME>>13) & 0xff ); 00058 00059 }else if(TMID == 2){ 00060 frames[TMID][5] =( (RTC_TIME>>32) & 0xff ); 00061 frames[TMID][6] =( (RTC_TIME>>24) & 0xff ); 00062 frames[TMID][7] =( (RTC_TIME>>16) & 0xff ); 00063 frames[TMID][8] =( (RTC_TIME>>8 ) & 0xff ); 00064 frames[TMID][9] =( (RTC_TIME ) & 0xff ); 00065 } 00066 00067 } 00068 00069 void convolution (unsigned char * ptr){ 00070 00071 ConvObj.convolutionEncode(ptr , TM_convoluted_data); 00072 ConvObj.convolutionEncode(ptr + 67, TM_convoluted_data + 135); 00073 00074 } 00075 00076 /* 00077 @brief : take the address of array of LCR or HCR and stores it into a frame 00078 @parameters: type->L or H , deprnding on wheather it is LCR or HCR respectively 00079 @return: nothing 00080 */ 00081 00082 // type 2 yet to be done 00083 void making_frame(unsigned char TMID ,unsigned char type, unsigned char* pointer){ 00084 00085 TMID--; //TMID goes from 1 to 3 , convinient to ue from 0 to 2 00086 static int frame_space_number[3] = {0}; //this variable represents the register number of the frame in which LCR or HCR data to be written not including header 00087 int packet_len = 0; 00088 int copy_count = 0 ; 00089 00090 switch(int(TMID)){ 00091 case 0: //SCP 00092 if(type == 'L'){ //below threshold 00093 packet_len = 22; 00094 } 00095 else if(type == 'H'){ //above threshold 00096 packet_len = 26; 00097 } 00098 break; 00099 00100 case 1: //SFP above threshold 00101 packet_len = 35; 00102 break; 00103 00104 case 2: //SFP below threshold 00105 packet_len = 23; 00106 break; 00107 } 00108 00109 if(SCH_FCCH_FLAG){ 00110 add_SCH_FCCH(); 00111 SCH_FCCH_FLAG = false; 00112 } 00113 00114 if(fresh[TMID]){ 00115 //welcome to first frame 00116 making_frameHeader(TMID); 00117 frames[TMID][4] = 0; 00118 fresh[TMID] = false; 00119 } 00120 00121 00122 while(copy_count < packet_len){ // 22 bytes is the size of the LCR 00123 frames[TMID][ frame_space_number[TMID] + data_starting_point[TMID] ]= *(pointer + copy_count); 00124 frame_space_number[TMID]++; 00125 copy_count++; 00126 if( frame_space_number[TMID] == max_data[TMID] ){ //frame space number can go from 0 to 126 as data is written from 0+5 to 126+5 00127 FCN[TMID]++; 00128 // convolution and save frame in the sd card 00129 00130 // copying crc in 132 and 133 00131 int temp_crc; 00132 temp_crc = crc16_gen(frames[TMID],132); 00133 frames[TMID][132] = temp_crc>>8; 00134 frames[TMID][133] = temp_crc & 0xff; 00135 // xor 00136 for(int j = 0 ; j < 134 ; j++){ 00137 // frames[TMID][j] = frames[TMID][j]^exorThisWithTMFrame[j]; 00138 } 00139 //convolution and interleaving 00140 convolution(frames[TMID]); 00141 interleave(TM_convoluted_data , complete_frame + 5); 00142 interleave(TM_convoluted_data+ 135,complete_frame + 164); 00143 00144 // writing the SDC_address in a buffer , to store it in SDcard at address 5 00145 SDcard_lastWritten[0] = SDC_address>>56; 00146 SDcard_lastWritten[1] = (SDC_address>>48)&0xFF; 00147 SDcard_lastWritten[2] = (SDC_address>>40)&0xFF; 00148 SDcard_lastWritten[3] = (SDC_address>>32)&0xFF; 00149 SDcard_lastWritten[4] = (SDC_address>>24)&0xFF; 00150 SDcard_lastWritten[5] = (SDC_address>>16)&0xFF; 00151 SDcard_lastWritten[6] = (SDC_address>>8)&0xFF; 00152 SDcard_lastWritten[7] = (SDC_address)&0xFF; 00153 00154 SPI_mutex.lock(); 00155 disk_write(complete_frame , SDC_address); 00156 SPI_mutex.unlock(); 00157 SDC_address++; 00158 00159 00160 00161 //now save to the sd card TM_convoluted_data 00162 // std::bitset<8> b; 00163 // printf("\nthis is frame %d\n",TMID); //for printing frame 00164 // for(int j =0; j<134;j++){ 00165 // printf(" %d",frames[TMID][j]); 00166 //// b = frames[TMID][j]; 00167 //// cout<<b; 00168 // } 00169 00170 frame_space_number[TMID] = 0; 00171 making_frameHeader(TMID); 00172 frames[TMID][4]=packet_len - copy_count; 00173 //write time here also 00174 continue; 00175 } 00176 } 00177 00178 // printf("\nthis is frame %d\n",TMID); //for printing frame 00179 // for(int j =0; j<134;j++){ 00180 // printf(" %d",frames[TMID][j]); 00181 // } 00182 00183 } 00184 00185 00186 } 00187 00188 00189 00190 00191 00192 namespace Science_Data_Compression{ 00193 00194 # define PACKET_SEQUENCE_COUNT 1 //1 byte 00195 # define NUM_PROTON_BIN 17 //2 byte each 00196 # define NUM_ELECTRON_BIN 14 //2 byte each 00197 # define VETO 1 //2 byte 00198 # define FASTCHAIN 2 //4 byte each 00199 #define RAW_PACKET_LENGTH 73 //73 bytes 00200 00201 // #define PACKET_SEQ_COUNT 1 00202 // #define PROTON_BIN_SIZE 2 00203 // #define ELECTRON_BIN_SIZE 2 00204 // #define VETO 2 00205 // #define FAST_CHAIN 4 00206 00207 00208 /* 00209 @brief: read one uint16_t equivalent of first two chars from the stream. short int because 16 bits 00210 @param: pointer to the start of the short int 00211 @return: uint16_t 00212 */ 00213 00214 00215 unsigned int read_2byte(unsigned char* ptr){ 00216 unsigned int output = (unsigned int) *(ptr+1); 00217 output += ( (unsigned int)(*ptr) ) << 8; 00218 return output; 00219 } 00220 00221 /* 00222 @brief: read one int equivalent of first four chars from the stream. int because 32 bits 00223 @param: pointer to the start of the short int 00224 @return: unsigned int 00225 */ 00226 00227 unsigned int read_4byte(unsigned char* ptr){ 00228 unsigned int output = (unsigned int) *(ptr+3); 00229 output += (unsigned int)*(ptr+2)<<8; 00230 output += (unsigned int)*(ptr+1)<<16; 00231 output += (unsigned int)*(ptr)<<24; 00232 return output; 00233 } 00234 00235 unsigned int SFP_thresholds[35]={0 ,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100};//threashold values 00236 unsigned int SCP_thresholds[35]={0 ,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,500,0 ,0 ,0 }; 00237 unsigned int SFP_bin[35]; 00238 unsigned int SCP_bin[35]={0}; 00239 unsigned char SFP_outputBT[23]; //BT = below threshold 00240 unsigned char SFP_outputAT[35]; 00241 unsigned char SCP_outputLCR[22]; 00242 unsigned char SCP_outputHCR[26]; 00243 00244 00245 //********************************************************************************************************************************************************************** 00246 //lots of compression functions are listed below 00247 00248 00249 unsigned char SFP_compress4_BT(unsigned int input){ //for veto 00250 int de_4 = 0; 00251 unsigned char output; 00252 00253 if(input<= 3){ 00254 // DE = 0; 00255 output = 0x0; 00256 de_4 = 0; 00257 } 00258 else if(input <= 12){ 00259 // DE = 01; 00260 output = 0x1; 00261 de_4 = 2; 00262 } 00263 else if(input <= 48){ 00264 // DE = 10 00265 output = 0x2; 00266 de_4 = 4; 00267 } 00268 else { 00269 // DE = 11 00270 output = 0x3; 00271 de_4 = 6; 00272 } 00273 00274 unsigned short int temp = input >> de_4; 00275 output += (temp ) << 2; 00276 return output; 00277 } 00278 00279 00280 unsigned char SFP_compress5_BT(unsigned int input){ 00281 int de_4 = 0; //number by which bin value need to be shifted 00282 unsigned char output; 00283 00284 if(input <= 15){ 00285 // D = 0 [0] 00286 output = 0x0; 00287 de_4 = 0; 00288 } 00289 else if(input <= 60){ 00290 // D = 1 00291 output = 0x1; 00292 de_4 = 2; 00293 } 00294 00295 unsigned short int temp = input >> de_4; 00296 output += (temp ) << 1; 00297 00298 return output; 00299 }; 00300 00301 00302 unsigned char SFP_compress6_BT(unsigned int input){ 00303 int de_4 = 0;; 00304 unsigned char output; 00305 00306 if(input <= 31){ 00307 // E = 0 [0] 00308 output = 0x0; 00309 de_4 = 0; 00310 } 00311 else if(input <= 124){ 00312 // E = 1 00313 output = 0x1; 00314 de_4 = 2; 00315 } 00316 00317 00318 unsigned short int temp = input >> de_4; 00319 output += (temp ) << 1; 00320 00321 return output; 00322 }; 00323 00324 unsigned char SFP_compress7_AT(unsigned int input){ 00325 int de_4 = 0; 00326 unsigned char output; 00327 00328 if(input <= 31){ 00329 // DE = 00 [0] 00330 output = 0x0; 00331 de_4 = 0; 00332 } 00333 else if(input <= 124){ 00334 // DE = 01 [1] 00335 output = 0x1; 00336 de_4 = 2; 00337 } 00338 00339 else if(input <= 496){ 00340 // DE = 10 [2] 00341 output = 0x2; 00342 de_4 = 4; 00343 } 00344 00345 else if(input <= 1984){ 00346 // DE = 11 [3] 00347 output = 0x3; 00348 de_4 = 6; 00349 } 00350 00351 unsigned short int temp = input >> de_4; 00352 output += (temp ) << 2; 00353 00354 return output; 00355 00356 }; 00357 00358 00359 unsigned char SFP_compress8_AT(unsigned int input){ 00360 00361 int de_4 = 0;; 00362 unsigned char output; 00363 00364 if(input <= 63){ 00365 // DE = 00 [0] 00366 output = 0x0; 00367 de_4 = 0; 00368 } 00369 else if(input <= 252){ 00370 // DE = 01 [1] 00371 output = 0x1; 00372 de_4 = 2; 00373 } 00374 00375 else if(input <= 1008){ 00376 // DE = 10 [2] 00377 output = 0x2; 00378 de_4 = 4; 00379 } 00380 00381 else { 00382 // DE = 11 [3] 00383 output = 0x3; 00384 de_4 = 6; 00385 } 00386 00387 unsigned short int temp = input >> de_4; 00388 output += (temp ) << 2; 00389 00390 return output; 00391 }; 00392 00393 unsigned char SFP_compress5_AT(unsigned int input){ 00394 int de_4 = 0;; 00395 unsigned char output; 00396 00397 if(input <= 3){ 00398 // DE = 000 [0] 00399 output = 0x0; 00400 de_4 = 0; 00401 } 00402 else if(input <= 12){ 00403 // DE = 001 [1] 00404 output = 0x1; 00405 de_4 = 2; 00406 } 00407 00408 else if(input <= 48){ 00409 // DE = 010 [2] 00410 output = 0x2; 00411 de_4 = 4; 00412 } 00413 00414 else if(input <= 192) { 00415 // DE = 011 [3] 00416 output = 0x3; 00417 de_4 = 6; 00418 } 00419 00420 else if(input <= 768) { 00421 // DE = 100 [4] 00422 output = 0x4; 00423 de_4 = 8; 00424 } 00425 00426 else if(input <= 3072) { 00427 // DE = 101 [5] 00428 output = 0x5; 00429 de_4 = 10; 00430 } 00431 00432 else if(input <= 12288) { 00433 // DE = 110 [6] 00434 output = 0x6; 00435 de_4 = 12; 00436 } 00437 00438 else { 00439 // DE = 111 [7] 00440 output = 0x7; 00441 de_4 = 14; 00442 } 00443 00444 unsigned short int temp = input >> de_4; 00445 output += (temp ) << 3; 00446 00447 return output; 00448 } 00449 00450 unsigned char SFP_compress7FC_AT(unsigned int input){ // for fast chain above threshold 00451 int de_4 = 0;; 00452 unsigned char output; 00453 00454 if(input <= 15){ 00455 // DE = 000 [0] 00456 output = 0x0; 00457 de_4 = 0; 00458 } 00459 else if(input <= 60){ 00460 // DE = 001 [1] 00461 output = 0x1; 00462 de_4 = 2; 00463 } 00464 00465 else if(input <= 240){ 00466 // DE = 010 [2] 00467 output = 0x2; 00468 de_4 = 4; 00469 } 00470 00471 else if(input <= 960) { 00472 // DE = 011 [3] 00473 output = 0x3; 00474 de_4 = 6; 00475 } 00476 00477 else if(input <= 3840) { 00478 // DE = 100 [4] 00479 output = 0x4; 00480 de_4 = 8; 00481 } 00482 00483 else if(input <= 15360) { 00484 // DE = 101 [5] 00485 output = 0x5; 00486 de_4 = 10; 00487 } 00488 00489 else if(input <= 61440) { 00490 // DE = 110 [6] 00491 output = 0x6; 00492 de_4 = 12; 00493 } 00494 00495 else { 00496 // DE = 111 [7] 00497 output = 0x7; 00498 de_4 = 14; 00499 } 00500 00501 unsigned short int temp = input >> de_4; 00502 output += (temp ) << 3; 00503 00504 return output; 00505 } 00506 00507 00508 00509 //++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00510 unsigned char SCP_compress6(unsigned int input){ 00511 int ef_4; 00512 unsigned char output; 00513 00514 if(input <= 15){ 00515 // EF = 00 00516 output = 0x0; 00517 ef_4 = 0; 00518 } 00519 00520 else if(input <= 60 ){ 00521 // EF = 01 [1] 00522 output = 0x01; 00523 ef_4 = 2; 00524 } 00525 else if(input <= 240){ 00526 // EF = 10 [2] 00527 output = 0x02; 00528 ef_4 = 4; 00529 } 00530 else{ 00531 // EF = 11 [3] 00532 output = 0x03; 00533 ef_4 = 6; 00534 } 00535 00536 unsigned short int temp = input >> ef_4; 00537 output += (temp & 0xf) << 2; 00538 00539 return output; 00540 } 00541 00542 unsigned char SCP_compress5(unsigned int input){ 00543 00544 int de_4 = 0;; 00545 unsigned char output; 00546 00547 if(input <= 7){ 00548 // DE = 00 [0] 00549 output = 0x0; 00550 de_4 = 0; 00551 } 00552 00553 else if(input <= 28){ 00554 // DE = 01 [1] 00555 output = 0x01; 00556 de_4 = 2; 00557 } 00558 else if(input <= 112){ 00559 // DE = 10 [2] 00560 output = 0x02; 00561 de_4 = 4; 00562 } 00563 else{ 00564 // DE = 11 [3] 00565 output = 0x03; 00566 de_4 = 6; 00567 } 00568 00569 unsigned short int temp = input >> de_4; 00570 output += (temp & 0x7) << 2; 00571 00572 return output; 00573 } 00574 00575 unsigned char SCP_compress6h(unsigned int input) { 00576 00577 int ef_4; 00578 unsigned char output; 00579 00580 if(input <=7){ 00581 // EF = 000 [0] 00582 output = 0x00; 00583 ef_4 = 0; 00584 } 00585 00586 else if(input <=28){ 00587 // EF = 001 [1] 00588 output = 0x01; 00589 ef_4 = 2; 00590 } 00591 else if(input <= 112){ 00592 00593 // EF = 010 [2] 00594 output = 0x02; 00595 ef_4 = 4; 00596 } 00597 else if(input <= 448){ 00598 // EF = 011 [3] 00599 output = 0x03; 00600 ef_4 = 6; 00601 } 00602 else if(input <= 1792){ 00603 // EF = 100 [4] 00604 output = 0x04; 00605 ef_4 = 8; 00606 00607 } 00608 else if(input <= 7168){ 00609 // EF = 101 [5] 00610 output = 0x05; 00611 ef_4 = 10; 00612 00613 } 00614 else if(input <= 28672){ 00615 // EF = 110 [6] 00616 output = 0x06; 00617 ef_4 = 12; 00618 } 00619 else{ 00620 // EF = 111 [7] 00621 output = 0x07; 00622 ef_4 =14; 00623 } 00624 00625 unsigned short int temp = input >> ef_4; 00626 output += (temp & 0x7) << 3; 00627 00628 return output; 00629 00630 } 00631 00632 00633 unsigned char SCP_compress7h(unsigned int input) { 00634 00635 int fg_4; 00636 unsigned char output; 00637 00638 if(input <= 15){ 00639 // EF = 000 [0] 00640 output = 0x0; 00641 fg_4 = 0; 00642 } 00643 00644 else if(input <= 60){ 00645 // EF = 001 [1] 00646 output = 0x01; 00647 fg_4 = 2; 00648 } 00649 else if(input <= 240){ 00650 00651 // EF = 010 [2] 00652 output = 0x02; 00653 fg_4 = 4; 00654 } 00655 else if(input <= 960){ 00656 // EF = 011 [3] 00657 output = 0x03; 00658 fg_4 = 6; 00659 } 00660 else if(input <= 3840){ 00661 // EF = 100 [4] 00662 output = 0x04; 00663 fg_4 = 8; 00664 00665 } 00666 else if(input <= 15360){ 00667 // EF = 101 [5] 00668 output = 0x05; 00669 fg_4 = 10; 00670 00671 } 00672 else if(input <= 61440){ 00673 // EF = 110 [6] 00674 output = 0x06; 00675 fg_4 = 12; 00676 } 00677 else{ 00678 // EF = 111 [7] 00679 output = 0x07; 00680 fg_4 =14; 00681 } 00682 00683 unsigned short int temp = input >> fg_4; 00684 output += (temp & 0xf) << 3; 00685 00686 return output; 00687 00688 } 00689 //+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++ 00690 void SCP_compress_data(); 00691 void SFP_compress_data(unsigned char* input){ 00692 00693 bool LCR = true; 00694 int i = 0; 00695 static int packet_no = 0; //takes value from 0 to 29 00696 //TRAVERSE THE LIST TO DETERMINE LCR OR HCR and stroing the values in proton_bin and electron bin 00697 SFP_bin[0] = *input; 00698 for(i=1 ; i<= NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO ; ++i){ //storing the bin values into an array name bin 00699 SFP_bin[i]=read_2byte(input+1+((i-1)<<1)); //proton bin and elecron bin are 2 byte, hence read_2byte and 00700 SCP_bin[i]+=SFP_bin[i]; 00701 if(SFP_bin[i] > SFP_thresholds[i]){ //fast cahin is 4 byte hence read_4byte 00702 LCR = false; // if a single value is above threshold then lcr becomes false 00703 i++; 00704 break; 00705 } 00706 } 00707 00708 for( ; i<= NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO ; ++i){ 00709 SCP_bin[i]+=SFP_bin[i]; 00710 SFP_bin[i] = read_2byte(input + 1 + ( (i-1)<<1) ); 00711 } 00712 00713 SFP_bin[i] = read_4byte(input+1+ ((i-1)<<1)) ; 00714 SCP_bin[i]+=SFP_bin[i]; 00715 00716 if(SFP_bin[i]>SFP_thresholds[i]) 00717 LCR = false; //since veto starts from location (input + 65) and (input + 69) 00718 00719 SFP_bin[i+1] = read_4byte(input+69); 00720 SCP_bin[i]+=SFP_bin[i]; 00721 00722 if(SFP_bin[i]>SFP_thresholds[i]) 00723 LCR = false; 00724 00725 00726 // printf("\n"); //for printing the sfp bin 00727 // for (i=0;i<35;i++){ 00728 // printf("sfp[%d] = %d",i,SFP_bin[i]); 00729 // } 00730 // printf("\n"); 00731 00732 if(LCR){ 00733 00734 SFP_outputBT[0] = (packet_no<<3) + ( SFP_compress5_BT(SFP_bin[1])>>2 ); 00735 SFP_outputBT[1] = ( SFP_compress5_BT(SFP_bin[1])<<6 ) + ( SFP_compress5_BT(SFP_bin[2])<<1 ) + ( SFP_compress5_BT(SFP_bin[3])>>4 ); 00736 SFP_outputBT[2] = ( SFP_compress5_BT(SFP_bin[3])<<4 ) + ( SFP_compress5_BT(SFP_bin[4])>>1 ); 00737 SFP_outputBT[3] = ( SFP_compress5_BT(SFP_bin[4])<<7 ) + ( SFP_compress5_BT(SFP_bin[5])<<2 ) + ( SFP_compress5_BT(SFP_bin[6])>>3 ); 00738 SFP_outputBT[4] = ( SFP_compress5_BT(SFP_bin[6])<<5 ) + ( SFP_compress5_BT(SFP_bin[7]) ); 00739 SFP_outputBT[5] = ( SFP_compress5_BT(SFP_bin[8])<<3 ) + ( SFP_compress5_BT(SFP_bin[9])>>2 ); 00740 SFP_outputBT[6] = ( SFP_compress5_BT(SFP_bin[9])<<6 ) + ( SFP_compress5_BT(SFP_bin[10])<<1 ) + ( SFP_compress5_BT(SFP_bin[11])>>4 ); 00741 SFP_outputBT[7] = ( SFP_compress5_BT(SFP_bin[11])<<4 ) + ( SFP_compress5_BT(SFP_bin[12])>>1 ); 00742 SFP_outputBT[8] = ( SFP_compress5_BT(SFP_bin[12])<<7 ) + ( SFP_compress5_BT(SFP_bin[13])<<2 ) + ( SFP_compress5_BT(SFP_bin[14])>>3 ); 00743 SFP_outputBT[9] = ( SFP_compress5_BT(SFP_bin[14])<<5 ) + ( SFP_compress5_BT(SFP_bin[15]) ); 00744 SFP_outputBT[10] = ( SFP_compress5_BT(SFP_bin[16])<<3 ) + ( SFP_compress5_BT(SFP_bin[17])>>2 ); 00745 SFP_outputBT[11] = ( SFP_compress5_BT(SFP_bin[17])<<6 ) + ( SFP_compress6_BT(SFP_bin[18]) ); 00746 SFP_outputBT[12] = ( SFP_compress6_BT(SFP_bin[19])<<2 ) + ( SFP_compress6_BT(SFP_bin[20])>>4 ); 00747 SFP_outputBT[13] = ( SFP_compress6_BT(SFP_bin[20])<<4 ) + ( SFP_compress5_BT(SFP_bin[21])>>1 ); 00748 SFP_outputBT[14] = ( SFP_compress5_BT(SFP_bin[21])<<7 ) + ( SFP_compress5_BT(SFP_bin[22])<<2 ) + ( SFP_compress5_BT(SFP_bin[23])>>3 ); 00749 SFP_outputBT[15] = ( SFP_compress5_BT(SFP_bin[23])<<5 ) + ( SFP_compress5_BT(SFP_bin[24]) ); 00750 SFP_outputBT[16] = ( SFP_compress5_BT(SFP_bin[25])<<3 ) + ( SFP_compress5_BT(SFP_bin[26])>>2 ); 00751 SFP_outputBT[17] = ( SFP_compress5_BT(SFP_bin[26])<<6 ) + ( SFP_compress5_BT(SFP_bin[27])<<1 ) + ( SFP_compress5_BT(SFP_bin[28])>>4); 00752 SFP_outputBT[18] = ( SFP_compress5_BT(SFP_bin[28])<<4 ) + ( SFP_compress5_BT(SFP_bin[29])>>1) ; 00753 SFP_outputBT[19] = ( SFP_compress5_BT(SFP_bin[29])<<7 ) + ( SFP_compress5_BT(SFP_bin[30])<<2 ) + ( SFP_compress5_BT(SFP_bin[31])>>3) ; 00754 SFP_outputBT[20] = ( SFP_compress5_BT(SFP_bin[31])<<5 ) + ( SFP_compress4_BT(SFP_bin[32])<<1) + ( SCP_compress5(SFP_bin[33])>>4 ) ; // 00755 SFP_outputBT[21] = ( SCP_compress5(SFP_bin[33])<<4 ) + ( SCP_compress5(SFP_bin[34])>>1 ); //here intentionally SCP_compress is used instead of SCP_compress5 is different than SFP_compress5 00756 SFP_outputBT[22] = ( SCP_compress5(SFP_bin[34])<<7 ); //7 bits are spare 00757 00758 Science_TMframe::making_frame(3,'L',SFP_outputBT); 00759 if(++packet_no == 30){ 00760 packet_no=0; 00761 SCP_compress_data(); 00762 for(i=0;i<PACKET_SEQUENCE_COUNT + NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO + FASTCHAIN;i++){ 00763 if(packet_no==0){ 00764 SCP_bin[i]=0; 00765 } 00766 } 00767 } 00768 00769 00770 } 00771 00772 else { 00773 00774 00775 SFP_outputAT[0] = (RTC_TIME>>27)&(0xff); 00776 SFP_outputAT[1] = (RTC_TIME>>19)&(0xff); 00777 SFP_outputAT[2] = (RTC_TIME>>11)&(0xff); 00778 SFP_outputAT[3] = (RTC_TIME>>3 )&(0xff); 00779 SFP_outputAT[4] = (RTC_TIME<<5 )&(0xff) + (packet_no); 00780 SFP_outputAT[5] = ( SFP_compress7_AT(SFP_bin[1])<<1 ) + ( SFP_compress7_AT(SFP_bin[2])>>6 ); 00781 SFP_outputAT[6] = ( SFP_compress7_AT(SFP_bin[2])<<2 ) + ( SFP_compress7_AT(SFP_bin[3])>>5 ); 00782 SFP_outputAT[7] = ( SFP_compress7_AT(SFP_bin[3])<<3 ) + ( SFP_compress7_AT(SFP_bin[4])>>4 ); 00783 SFP_outputAT[8] = ( SFP_compress7_AT(SFP_bin[4])<<4 ) + ( SFP_compress7_AT(SFP_bin[5])>>3 ); 00784 SFP_outputAT[9] = ( SFP_compress7_AT(SFP_bin[5])<<5 ) + ( SFP_compress7_AT(SFP_bin[6])>>2 ); 00785 SFP_outputAT[10] = ( SFP_compress7_AT(SFP_bin[6])<<6 ) + ( SFP_compress7_AT(SFP_bin[7])>>1 ); 00786 SFP_outputAT[11] = ( SFP_compress7_AT(SFP_bin[7])<<7 ) + ( SFP_compress7_AT(SFP_bin[8]) ); 00787 SFP_outputAT[12] = ( SFP_compress7_AT(SFP_bin[9])<<1 ) + ( SFP_compress7_AT(SFP_bin[10])>>6); 00788 SFP_outputAT[13] = ( SFP_compress7_AT(SFP_bin[10])<<2 ) + ( SFP_compress7_AT(SFP_bin[11])>>5); 00789 SFP_outputAT[14] = ( SFP_compress7_AT(SFP_bin[11])<<3 ) + ( SFP_compress7_AT(SFP_bin[12])>>4); 00790 SFP_outputAT[15] = ( SFP_compress7_AT(SFP_bin[12])<<4 ) + ( SFP_compress7_AT(SFP_bin[13])>>3); 00791 SFP_outputAT[16] = ( SFP_compress7_AT(SFP_bin[13])<<5 ) + ( SFP_compress7_AT(SFP_bin[14])>>2); 00792 SFP_outputAT[17] = ( SFP_compress7_AT(SFP_bin[14])<<6 ) + ( SFP_compress7_AT(SFP_bin[15])>>1); 00793 SFP_outputAT[18] = ( SFP_compress7_AT(SFP_bin[15])<<7 ) + ( SFP_compress7_AT(SFP_bin[16])); 00794 SFP_outputAT[19] = ( SFP_compress7_AT(SFP_bin[17])<<1 ) + ( SFP_compress8_AT(SFP_bin[18])>>7 ); 00795 SFP_outputAT[20] = ( SFP_compress8_AT(SFP_bin[18])<<1 ) + ( SFP_compress8_AT(SFP_bin[19])>>7 ); 00796 SFP_outputAT[21] = ( SFP_compress8_AT(SFP_bin[19])<<1 ) + ( SFP_compress8_AT(SFP_bin[20])>>7 ); 00797 SFP_outputAT[22] = ( SFP_compress8_AT(SFP_bin[20])<<1 ) + ( SFP_compress7_AT(SFP_bin[21])>>6 ); 00798 SFP_outputAT[23] = ( SFP_compress7_AT(SFP_bin[21])<<2 ) + ( SFP_compress7_AT(SFP_bin[22])>>5 ); 00799 SFP_outputAT[24] = ( SFP_compress7_AT(SFP_bin[22])<<3 ) + ( SFP_compress7_AT(SFP_bin[23])>>4 ); 00800 SFP_outputAT[25] = ( SFP_compress7_AT(SFP_bin[23])<<4 ) + ( SFP_compress7_AT(SFP_bin[24])>>3 ); 00801 SFP_outputAT[26] = ( SFP_compress7_AT(SFP_bin[24])<<5 ) + ( SFP_compress7_AT(SFP_bin[25])>>2 ); 00802 SFP_outputAT[27] = ( SFP_compress7_AT(SFP_bin[25])<<6 ) + ( SFP_compress7_AT(SFP_bin[26])>>1 ); 00803 SFP_outputAT[28] = ( SFP_compress7_AT(SFP_bin[26])<<7 ) + ( SFP_compress7_AT(SFP_bin[27]) ); 00804 SFP_outputAT[29] = ( SFP_compress7_AT(SFP_bin[28])<<1 ) + ( SFP_compress7_AT(SFP_bin[29])>>6); 00805 SFP_outputAT[30] = ( SFP_compress7_AT(SFP_bin[29])<<2 ) + ( SFP_compress7_AT(SFP_bin[30])>>5); 00806 SFP_outputAT[31] = ( SFP_compress7_AT(SFP_bin[30])<<3 ) +( SFP_compress7_AT(SFP_bin[31])>>4); 00807 SFP_outputAT[32] = ( SFP_compress7_AT(SFP_bin[31])<<4 ) +( SFP_compress5_AT(SFP_bin[32])>>1); 00808 SFP_outputAT[33] = ( SFP_compress5_AT(SFP_bin[32])<<7 ) +( SFP_compress7FC_AT(SFP_bin[33])); 00809 SFP_outputAT[34] = ( SFP_compress7FC_AT(SFP_bin[34])<<1 ); // 1 bit is spare 00810 00811 Science_TMframe::making_frame(2,'H',SFP_outputAT); 00812 if(++packet_no == 30){ 00813 packet_no=0; 00814 SCP_compress_data(); 00815 for(i=0;i<PACKET_SEQUENCE_COUNT + NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO + FASTCHAIN;i++){ 00816 if(packet_no==0){ 00817 SCP_bin[i]=0; 00818 } 00819 } 00820 } 00821 00822 } 00823 00824 } 00825 00826 00827 /* 00828 brief: takes the pointer of the raw data string and return the address of the array which stores the address of 30 packets. 00829 input: pointer to the raw data. 00830 output : void 00831 */ 00832 00833 void complete_compression(unsigned char *SRP,uint64_t x){ 00834 RTC_TIME = x; 00835 int i; //30 times because 3 second data 00836 00837 for(i=0;i<30;i++){ 00838 SFP_compress_data(SRP + 73*i); 00839 } 00840 00841 } 00842 00843 00844 00845 ///////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////////// 00846 00847 00848 00849 /* 00850 @brief: compresses the given input stream and return output packet 00851 @param: pointer to input stream. Input stream always has the fixed size of RAW_PACKET_LENGTH 00852 @return: pointer to output stream. Output stream has the size of 22 or 26 bytes 00853 */ 00854 void SCP_compress_data(){ 00855 00856 bool LCR = true; 00857 int i = 0; 00858 00859 for(i=1;i<=PACKET_SEQUENCE_COUNT + NUM_PROTON_BIN + NUM_ELECTRON_BIN + VETO + FASTCHAIN ;i++){ 00860 if(SCP_bin[i]>SCP_thresholds[i]){ 00861 LCR = false; 00862 break; 00863 } 00864 } 00865 // printf("\n"); //for printing the scp bin 00866 // for (i=0;i<35;i++){ 00867 // printf(" scp[%d] = %d ",i,SCP_bin[i]); 00868 // } 00869 // printf("\n"); 00870 // compressing the data 00871 if(LCR){ 00872 SCP_outputLCR[0] = (RTC_TIME>>5)&(0xff); //first 13 bits for time tagging 00873 SCP_outputLCR[1] = (RTC_TIME<<3)&(0xff); //then 4 bits for attitude tag 00874 SCP_outputLCR[2] = 0x00; //only attitude tag is left 00875 SCP_outputLCR[2] += ( SCP_compress5(SCP_bin[0])<<1 ) + ( SCP_compress5(SCP_bin[1])>>4 ); 00876 SCP_outputLCR[3] = ( SCP_compress5(SCP_bin[1])<<4 ) + ( SCP_compress5(SCP_bin[2])>>1 ); 00877 SCP_outputLCR[4] = ( SCP_compress5(SCP_bin[2])<<7 ) + ( SCP_compress5(SCP_bin[3])<<2 ) + ( SCP_compress5(SCP_bin[4])>>3 ); 00878 SCP_outputLCR[5] = ( SCP_compress5(SCP_bin[4])<<5 ) + ( SCP_compress5(SCP_bin[5]) ); 00879 SCP_outputLCR[6] = ( SCP_compress5(SCP_bin[6])<<3 ) + ( SCP_compress5(SCP_bin[7])>>2 ); 00880 SCP_outputLCR[7] = ( SCP_compress5(SCP_bin[7])<<6 ) + ( SCP_compress5(SCP_bin[8])<<1 ) + ( SCP_compress5(SCP_bin[9])>>4 ); 00881 SCP_outputLCR[8] = ( SCP_compress5(SCP_bin[9])<<4 ) + ( SCP_compress5(SCP_bin[10])>>1 ); 00882 SCP_outputLCR[9] = ( SCP_compress5(SCP_bin[10])<<7 ) + ( SCP_compress5(SCP_bin[11])<<2) + ( SCP_compress5(SCP_bin[12])>>3 ); 00883 SCP_outputLCR[10] = ( SCP_compress5(SCP_bin[12])<<5 ) + ( SCP_compress5(SCP_bin[13]) ); 00884 SCP_outputLCR[11] = ( SCP_compress5(SCP_bin[14])<<3 ) + ( SCP_compress5(SCP_bin[15])>>2 ); 00885 SCP_outputLCR[12] = ( SCP_compress5(SCP_bin[15])<<6 ) + ( SCP_compress5(SCP_bin[16])<<1) + ( SCP_compress6(SCP_bin[17])>>5 ); 00886 SCP_outputLCR[13] = ( SCP_compress6(SCP_bin[17])<<3 ) + ( SCP_compress6(SCP_bin[18])>>3 ); 00887 SCP_outputLCR[14] = ( SCP_compress5(SCP_bin[18])<<5 ) + ( SCP_compress6(SCP_bin[19])>>1 ); 00888 SCP_outputLCR[15] = ( SCP_compress6(SCP_bin[19])<<7 ) + ( SCP_compress5(SCP_bin[20])<<2 ) + ( SCP_compress5(SCP_bin[21])>>3 ); 00889 SCP_outputLCR[16] = ( SCP_compress5(SCP_bin[21])<<5 ) + ( SCP_compress5(SCP_bin[22]) ); 00890 SCP_outputLCR[17] = ( SCP_compress5(SCP_bin[23])<<3 ) + ( SCP_compress5(SCP_bin[24])>>2 ); 00891 SCP_outputLCR[18] = ( SCP_compress5(SCP_bin[24])<<6 ) + ( SCP_compress5(SCP_bin[25])<<1) + ( SCP_compress5(SCP_bin[26])>>4 ); 00892 SCP_outputLCR[19] = ( SCP_compress5(SCP_bin[26])<<4 ) + ( SCP_compress5(SCP_bin[27])>>1 ); 00893 SCP_outputLCR[20] = ( SCP_compress5(SCP_bin[27])<<7 ) + ( SCP_compress5(SCP_bin[28])<<2 ) + ( SCP_compress5(SCP_bin[29])>>3 ); 00894 SCP_outputLCR[21] = ( SCP_compress5(SCP_bin[29])<<5 ) + ( SCP_compress5(SCP_bin[30]) ); 00895 00896 Science_TMframe::making_frame(1,'L',SCP_outputLCR); 00897 } 00898 else{ 00899 00900 SCP_outputLCR[0] = (RTC_TIME>>5)&(0xff); //first 13 bits for time tagging 00901 SCP_outputLCR[1] = (RTC_TIME<<3)&(0xff); 00902 SCP_outputHCR[2] = 0x40; 00903 SCP_outputHCR[2] += ( SCP_compress6h(SCP_bin[0])<<6 ) ; 00904 SCP_outputHCR[3] = ( SCP_compress6h(SCP_bin[1])<<2 ) + ( SCP_compress6h(SCP_bin[2])>>4) ; 00905 SCP_outputHCR[4] = ( SCP_compress6h(SCP_bin[2])<<4 ) + ( SCP_compress6h(SCP_bin[3])>>2) ; 00906 SCP_outputHCR[5] = ( SCP_compress6h(SCP_bin[3])<<6 ) + ( SCP_compress6h(SCP_bin[4])) ; 00907 SCP_outputHCR[6] = ( SCP_compress6h(SCP_bin[5])<<2 ) + ( SCP_compress6h(SCP_bin[6])>>4) ; 00908 SCP_outputHCR[7] = ( SCP_compress6h(SCP_bin[6])<<4 ) + ( SCP_compress6h(SCP_bin[7])>>2) ; 00909 SCP_outputHCR[8] = ( SCP_compress6h(SCP_bin[7])<<6 ) + ( SCP_compress6h(SCP_bin[8])) ; 00910 SCP_outputHCR[9] = ( SCP_compress6h(SCP_bin[9])<<2 ) + ( SCP_compress6h(SCP_bin[10])>>4) ; 00911 SCP_outputHCR[10] = ( SCP_compress6h(SCP_bin[10])<<4 ) + ( SCP_compress6h(SCP_bin[11])>>2); 00912 SCP_outputHCR[11] = ( SCP_compress6h(SCP_bin[11])<<6 ) + ( SCP_compress6h(SCP_bin[12])) ; 00913 SCP_outputHCR[12] = ( SCP_compress6h(SCP_bin[13])<<2 ) + ( SCP_compress6h(SCP_bin[14])>>4) ; 00914 SCP_outputHCR[13] = ( SCP_compress6h(SCP_bin[14])<<4 ) + ( SCP_compress6h(SCP_bin[15])>>2) ; 00915 SCP_outputHCR[14] = ( SCP_compress6h(SCP_bin[15])<<6 ) + ( SCP_compress6h(SCP_bin[16])) ; 00916 SCP_outputHCR[15] = ( SCP_compress7h(SCP_bin[17])<<1 ) + ( SCP_compress7h(SCP_bin[18])>>6) ; 00917 SCP_outputHCR[16] = ( SCP_compress7h(SCP_bin[18])<<2 ) + ( SCP_compress7h(SCP_bin[19])>>5) ; 00918 SCP_outputHCR[17] = ( SCP_compress7h(SCP_bin[19])<<3 ) + ( SCP_compress6h(SCP_bin[20])>>3) ; 00919 SCP_outputHCR[18] = ( SCP_compress6h(SCP_bin[20])<<5 ) + ( SCP_compress6h(SCP_bin[21])>>1) ; 00920 SCP_outputHCR[19] = ( SCP_compress6h(SCP_bin[21])<<7 ) + ( SCP_compress6h(SCP_bin[22])<<1) + ( SCP_compress6h(SCP_bin[23])>>5) ; 00921 SCP_outputHCR[20] = ( SCP_compress6h(SCP_bin[23])<<3 ) + ( SCP_compress6h(SCP_bin[24])>>3) ; 00922 SCP_outputHCR[21] = ( SCP_compress6h(SCP_bin[24])<<5 ) + ( SCP_compress6h(SCP_bin[25])>>1) ; 00923 SCP_outputHCR[22] = ( SCP_compress6h(SCP_bin[25])<<7 ) + ( SCP_compress6h(SCP_bin[26])<<1) + ( SCP_compress6h(SCP_bin[27])>>5) ; 00924 SCP_outputHCR[23] = ( SCP_compress6h(SCP_bin[27])<<3 ) + ( SCP_compress6h(SCP_bin[28])>>3) ; 00925 SCP_outputHCR[24] = ( SCP_compress6h(SCP_bin[28])<<5 ) + ( SCP_compress6h(SCP_bin[29])>>1) ; 00926 SCP_outputHCR[25] = ( SCP_compress6h(SCP_bin[29])<<7 ) + ( SCP_compress6h(SCP_bin[30])<<1) ; //last bit is empty 00927 00928 /* for (i=0;i<26;i++){ 00929 printf("\nscp[%d] = %d",i,SCP_outputHCR[i]); 00930 }*/ 00931 Science_TMframe::making_frame(1,'H',SCP_outputHCR); 00932 } 00933 } 00934 00935 } 00936
Generated on Tue Jul 12 2022 10:59:21 by
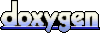