
cdms_update
Dependencies: FreescaleIAP mbed-rtos mbed
Fork of CDMS_SD_MNG_OVERDRIVE by
structure.h
00001 #include "mbed.h" 00002 //SIZE 00003 #define TC_SHORT_SIZE 11 00004 #define TC_LONG_SIZE 135 00005 #define TM_LONG_SIZE 134 00006 #define TM_SHORT_SIZE 13 00007 00008 //TELECOMMAND: 00009 00010 // exec_status: 00011 // 0: unexecuted 00012 // 1: successfully executed 00013 // 2: Execution Failed 00014 // 3: Disabled 00015 // 4: Marked For retry 00016 00017 //MASKS 00018 #define SHORT_LONG_TC_MASK 0x10 00019 #define CRC_MASK 0x08 00020 #define EXEC_STATUS_MASK 0x07 00021 00022 //USE ONLY THE BELOW MACROS TO MODIFY 'flags' VARIABLE 00023 //x should be a Base_tc pointer 00024 #define GETshort_or_long_tc(x) ( ( (x->flags) & SHORT_LONG_TC_MASK ) >> 4 ) 00025 #define GETcrc_pass(x) ( ( (x->flags) & CRC_MASK ) >> 3 ) 00026 #define GETabort_on_nack(x) ( ( (x->TC_string[1]) & 0x08 ) >> 3 ) 00027 #define GETapid(x) ( ( (x->TC_string[1]) & 0xC0 ) >> 6 ) 00028 #define GETexec_status(x) ( (x->flags) & EXEC_STATUS_MASK ) 00029 #define GETpacket_seq_count(x) (x->TC_string[0]) 00030 #define GETservice_type(x) ( (x->TC_string[2]) & 0xF0 ) 00031 #define GETservice_subtype(x) ( (x->TC_string[2]) & 0x0F ) 00032 #define GETpid(x) (x->TC_string[3]) 00033 00034 //x should be a Base_tc pointer 00035 //y should be a 16-bit number with relevant data in LSB 00036 //use in a seperate line with ; at the end: similar to a function 00037 #define PUTshort_or_long(x,y) x->flags = ( (x->flags) & ~(SHORT_LONG_TC_MASK)) | ( (y << 4) & SHORT_LONG_TC_MASK ) 00038 #define PUTcrc_pass(x,y) x->flags = ( (x->flags) & ~(CRC_MASK)) | ( (y << 3) & CRC_MASK) 00039 #define PUTexec_status(x,y) x->flags = ( (x->flags) & ~(EXEC_STATUS_MASK)) | ( y & EXEC_STATUS_MASK) 00040 00041 //PARENT TELECOMMAND CLASS 00042 class Base_tc{ 00043 public: 00044 uint8_t flags; 00045 uint8_t *TC_string; 00046 Base_tc *next_TC; 00047 00048 virtual ~Base_tc(){} 00049 }; 00050 00051 //DERIVED CLASS - SHORT TC 00052 class Short_tc : public Base_tc{ 00053 private: 00054 uint8_t fix_str[TC_SHORT_SIZE]; 00055 public: 00056 Short_tc(){ 00057 TC_string = fix_str; 00058 flags = 0; 00059 } 00060 00061 ~Short_tc(){} 00062 }; 00063 00064 //DERIVED CLASS - LONG TC 00065 class Long_tc : public Base_tc{ 00066 private: 00067 uint8_t fix_str[TC_LONG_SIZE]; 00068 public: 00069 Long_tc(){ 00070 TC_string = fix_str; 00071 flags = 0; 00072 } 00073 00074 ~Long_tc(){} 00075 }; 00076 00077 // TELEMETRY: 00078 // MASKS 00079 #define SHORT_LONG_TM_MASK 0x10 00080 #define TMID_MASK 0x0F 00081 00082 //x should be 'fields' variable defined in the Base_tm 00083 #define GETshort_or_long_tm(x) ((x & SHORT_LONG_TM_MASK) >> 4) 00084 #define GETtmid(x) (x & TMID_MASK) 00085 00086 //x should be 'fields' variable defines in the Base_tm 00087 //y should be an 8-bit number with relevent data at LSB 00088 #define PUTshort_or_long_tm(x,y) x = (x & ~(SHORT_LONG_TM_MASK)) | ((y<<4) & SHORT_LONG_TM_MASK) 00089 #define PUTtmid(x,y) x = (x & ~(TMID_MASK)) | (y & TMID_MASK) 00090 00091 // PARENT TELEMETRY CLASS 00092 class Base_tm{ 00093 public: 00094 uint8_t fields; 00095 uint8_t *TM_string; 00096 Base_tm *next_TM; 00097 00098 virtual ~Base_tm(){} 00099 }; 00100 00101 // DERIVED CLASS : Long tm [type 0] 00102 // type 0 00103 class Long_tm : public Base_tm{ 00104 private: 00105 uint8_t fix_str[TM_LONG_SIZE]; 00106 public: 00107 Long_tm(){ 00108 TM_string = fix_str; 00109 // type 0 00110 fields = 0; 00111 } 00112 00113 ~Long_tm(){} 00114 }; 00115 00116 // DERIVED CLASS : Short tm [type 1] 00117 // type 1 00118 class Short_tm : public Base_tm{ 00119 private: 00120 uint8_t fix_str[TM_SHORT_SIZE]; 00121 public: 00122 Short_tm(){ 00123 TM_string = fix_str; 00124 // type 1 00125 fields = 0x10; 00126 } 00127 00128 ~Short_tm(){} 00129 }; 00130 00131 //////////////////////////////END 00132 00133 00134 00135 00136 00137 00138 00139 00140 00141 00142 00143 00144 00145 00146 00147 00148 /*typedef struct TC_list{ 00149 // received from the RCV_TC 00150 unsigned char *TC_string; 00151 bool short_or_long; //'true' for short 00152 bool crc_pass; 00153 00154 // updated info - updated in MNG_TC 00155 unsigned char packet_seq_count; 00156 unsigned char apid; 00157 bool abort_on_nack; 00158 bool enabled; 00159 // bool valid_execution; 00160 unsigned char exec_status; 00161 00162 struct TC_list *next_TC; 00163 00164 ~TC_list(){} 00165 }TC_list; 00166 00167 typedef struct TM_list{ 00168 00169 unsigned char *TM_string; 00170 // bool short_or_long; // true for short 00171 // pass while calling the function 00172 unsigned char tmid; 00173 struct TM_list *next_TM; 00174 00175 ~TM_list(){} 00176 }TM_List;*/ 00177 00178 00179 00180 00181 00182 00183 00184 00185 00186 00187 00188 00189 00190 00191 00192 00193 00194 00195 00196 00197 00198 00199 ////PARENT CLASS 00200 //class Base_tc{ 00201 //public: 00202 // uint16_t fields; 00203 // uint8_t *TC_string; 00204 // Base_tc *next_node; 00205 00206 // short = 0, long = 1 00207 // bool GETshort_or_long(void){ 00208 // return (fields & SHORT_LONG_TC_MASK); 00209 // } 00210 // void PUTshort_or_long(bool input){ 00211 // if(input){ 00212 // fields |= SHORT_LONG_TC_MASK; 00213 // } 00214 // else{ 00215 // fields &= ~(SHORT_LONG_TC_MASK); 00216 // } 00217 // } 00218 // 00219 // bool inline GETcrc_pass(){ 00220 // return (fields & CRC_MASK); 00221 // } 00222 // void inline PUTcrc_pass(bool input){ 00223 // if(input){ 00224 // fields |= CRC_MASK; 00225 // } 00226 // else{ 00227 // fields &= ~(CRC_MASK); 00228 // } 00229 // } 00230 // 00231 // bool inline GETabort_on_nack(){ 00232 // return (fields & ABORT_ON_NACK_MASK); 00233 // } 00234 // void inline PUTabort_on_nack(bool input){ 00235 // if(input){ 00236 // fields |= ABORT_ON_NACK_MASK; 00237 // } 00238 // else{ 00239 // fields &= ~(ABORT_ON_NACK_MASK); 00240 // } 00241 // } 00242 // 00243 // uint8_t inline GETapid(){ 00244 // uint16_t temp = fields & APID_MASK; 00245 // temp = temp >> 10; 00246 // return (temp & 0xFF); 00247 // } 00248 // void inline PUTapid(uint8_t input){ 00249 // uint16_t temp = input; 00250 // temp = temp << 10; 00251 // fields &= ~(APID_MASK); 00252 // fields |= (temp & APID_MASK); 00253 // } 00254 // 00255 // uint8_t inline GETexec_status(){ 00256 // uint16_t temp = fields & EXEC_STATUS_MASK; 00257 // temp = temp >> 8; 00258 // return (temp & 0xFF); 00259 // } 00260 // void inline PUTexec_status(uint8_t input){ 00261 // uint16_t temp = input; 00262 // temp = temp << 8; 00263 // fields &= ~(EXEC_STATUS_MASK); 00264 // fields |= (temp & EXEC_STATUS_MASK); 00265 // } 00266 // 00267 // uint8_t inline GETpacket_seq_count(){ 00268 // uint16_t temp = fields & PACKET_SEQ_COUNT_MASK; 00269 // return (temp & 0xFF); 00270 // } 00271 // void inline PUTpacket_seq_count(uint8_t input){ 00272 // uint16_t temp = input; 00273 // fields &= ~(PACKET_SEQ_COUNT_MASK); 00274 // fields |= (temp & PACKET_SEQ_COUNT_MASK); 00275 // } 00276 // 00277 //// update everything other than short_or_long, and crc_pass from TC_string 00278 // void update_fields(){ 00279 //// abort on nack 00280 // uint8_t temp = TC_string[1]; 00281 // uint16_t t16 = 0; 00282 // if(temp & 0x10){ 00283 // fields |= ABORT_ON_NACK_MASK; 00284 // } 00285 // else{ 00286 // fields &= ~(ABORT_ON_NACK_MASK); 00287 // } 00288 // 00289 // // apid 00290 // t16 = temp; 00291 // t16 = t16 << 4; 00292 // fields &= ~(APID_MASK); 00293 // fields |= (t16 & APID_MASK); 00294 // 00295 // // exec_status : default value of exec status 00296 // fields &= ~(EXEC_STATUS_MASK); 00297 // 00298 // // packet seq count 00299 // temp = TC_string[0]; 00300 // t16 = temp; 00301 // fields &= ~(PACKET_SEQ_COUNT_MASK); 00302 // fields |= (t16 & PACKET_SEQ_COUNT_MASK); 00303 // } 00304 // 00305 // virtual ~Base_tc(){} 00306 //}; 00307 00308 ////DERIVED CLASS - SHORT TC 00309 //class Short_tc : public Base_tc{ 00310 //private: 00311 // uint8_t fix_str[TC_SHORT_SIZE]; 00312 //public: 00313 // Short_tc(){ 00314 // TC_string = fix_str; 00315 // fields = 0; 00316 // } 00317 // 00318 // ~Short_tc(){} 00319 //}; 00320 // 00321 ////DERIVED CLASS - LONG TC 00322 //class Long_tc : public Base_tc{ 00323 //private: 00324 // uint8_t fix_str[TC_LONG_SIZE]; 00325 //public: 00326 // Long_tc(){ 00327 // TC_string = fix_str; 00328 // fields = 0; 00329 // } 00330 // 00331 // ~Long_tc(){} 00332 //}; 00333 00334 //// TELEMETRY CLASS : 00335 // 00336 //// MASKS 00337 //#define SHORT_LONG_TM_MASK 0x10 00338 //#define TMID_MASK 0x0F 00339 // 00340 //// PARENT CLASS 00341 //class Base_tm{ 00342 //protected: 00343 // uint8_t fields; 00344 //public: 00345 // uint8_t *TM_string; 00346 // Base_tm *next_node; 00347 // 00348 // short = 0, long = 1 00349 // bool GETshort_or_long(){ 00350 // return (fields & SHORT_LONG_TM_MASK); 00351 // } 00352 // void PUTshort_or_long(bool input){ 00353 // if(input){ 00354 // fields |= SHORT_LONG_TM_MASK; 00355 // } 00356 // else{ 00357 // fields &= ~(SHORT_LONG_TM_MASK); 00358 // } 00359 // } 00360 // 00361 // uint8_t GETtmid(){ 00362 // return (fields & TMID_MASK); 00363 // } 00364 // void PUTtmid(uint8_t input){ 00365 // fields &= ~(TMID_MASK); 00366 // fields |= (input & TMID_MASK); 00367 // } 00368 // 00369 // virtual ~Base_tm(){} 00370 //}; 00371 00372 00373 //// DERIVED CLASS : Long tc [type 0] 00374 //// type 0 00375 //class Long_tm : public Base_tm{ 00376 //private: 00377 // uint8_t fix_str[TM_LONG_SIZE]; 00378 //public: 00379 // Long_tm(){ 00380 // TM_string = fix_str; 00381 // // type 0 00382 // fields = 0; 00383 // } 00384 // 00385 // ~Long_tm(){} 00386 //}; 00387 // 00388 //// DERIVED CLASS : Short tc [type 1] 00389 //// type 1 00390 //class Short_tm : public Base_tm{ 00391 //private: 00392 // uint8_t fix_str[TM_SHORT_SIZE]; 00393 //public: 00394 // Short_tm(){ 00395 // TM_string = fix_str; 00396 // // type 1 00397 // fields = 0x10; 00398 // } 00399 // 00400 // ~Short_tm(){} 00401 //};
Generated on Thu Jul 14 2022 03:00:44 by
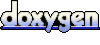