
hey
Dependencies: SDFileSystem mbed-rtos mbed
Fork of CDMS_BAE by
i2c.cpp
00001 #include "mbed.h" 00002 #include "rtos.h" 00003 #include "i2c.h" 00004 DigitalOut interrupt(D9); 00005 const int addr = 0x20; //slave address 00006 I2C master (D14,D15); //configure pins p27,p28 as I2C master 00007 Serial pc (USBTX,USBRX); 00008 //DigitalOut interrupt(D9); 00009 //InterruptIn data_ready(D10); 00010 00011 int reset; 00012 Timer t; 00013 Timer t1; 00014 Timer t2; 00015 Timer t3; 00016 00017 typedef struct 00018 { 00019 char data; // To avoid dynamic memory allocation 00020 int length; 00021 }i2c_data; 00022 00023 //Mail<i2c_data,16> i2c_data_receive; 00024 Mail<i2c_data,16> i2c_data_send; 00025 00026 //Thread * ptr_t_i2c; 00027 void FUNC_I2C_MASTER_FSLAVE(char * data,int length) 00028 { 00029 00030 00031 bool ack0 =true; 00032 00033 interrupt = 1; 00034 t1.start(); 00035 //wait_ms(20); 00036 ack0 = master.read(addr|1,data,length); 00037 t1.stop(); 00038 00039 00040 if(!ack0) 00041 { 00042 printf("\n master has read %s from slave\n\r",data); 00043 00044 } 00045 //master.stop(); 00046 00047 printf("\n%d\n\r",t1.read_us()); 00048 t1.reset(); 00049 storedata(data); 00050 interrupt=0; 00051 00052 } 00053 00054 typedef struct { 00055 char Voltage[9]; 00056 char Current[5]; 00057 char Temperature[2]; 00058 char PanelTemperature[3];//read by the 4 thermistors on solar panels 00059 char BatteryTemperature; //to be populated 00060 char faultpoll; //polled faults 00061 char faultir; //interrupted faults 00062 char power_mode; //power modes 00063 char AngularSpeed[3]; 00064 char Bnewvalue[3]; 00065 00066 //float magnetometer,gyro=>to be addes 00067 } hk_data; 00068 hk_data decode_data; 00069 00070 void TC_DECODE(char *data_hk) //getting the structure back from hk data sent by bae 00071 { 00072 for(int i=0;i<=7;i++) 00073 { 00074 decode_data.Voltage[i] = data_hk[i]; 00075 decode_data.Voltage[8] = '\0'; 00076 } 00077 for(int i=0;i<=3;i++) 00078 { 00079 decode_data.Current[i] = data_hk[8+i]; 00080 decode_data.Current[4] = '\0'; 00081 } 00082 decode_data.Temperature[0] = data_hk[12]; 00083 decode_data.Temperature[1] = '\0'; 00084 for(int i=0;i<=1;i++) 00085 { 00086 decode_data.PanelTemperature[i] = data_hk[13+i]; 00087 decode_data.PanelTemperature[2] = '\0'; 00088 } 00089 decode_data.BatteryTemperature = data_hk[15]; 00090 decode_data.faultpoll = data_hk[16]; 00091 decode_data.faultir = data_hk[17]; 00092 decode_data.power_mode = data_hk[18]; 00093 for(int i=0;i<=1;i++) 00094 { 00095 decode_data.AngularSpeed[i] = data_hk[19+i]; 00096 decode_data.AngularSpeed[2] = '\0'; 00097 } 00098 for(int i=0;i<=1;i++) 00099 { 00100 decode_data.Bnewvalue[i] = data_hk[21+i]; 00101 decode_data.Bnewvalue[2] = '\0'; 00102 } 00103 printf("\n voltage %s\n\r",decode_data.Voltage); 00104 printf("\n current %s\n\r",decode_data.Current); 00105 printf("\n faultpoll %c\n\r",decode_data.faultpoll); 00106 } 00107 00108 00109 00110 char writedata; 00111 bool write2slave; 00112 bool master_status_write; 00113 void FUNC_MASTER_WRITE() 00114 { //wait(1); 00115 write2slave=true; 00116 00117 00118 00119 char data = pc.getc(); 00120 interrupt = 1; 00121 t.start(); 00122 t3.start(); 00123 wait_ms(20); 00124 i2c_data * i2c_data_s = i2c_data_send.alloc(); 00125 i2c_data_s->data = data; 00126 i2c_data_s->length = 1; 00127 i2c_data_send.put(i2c_data_s); 00128 master_status_write = true; 00129 00130 // interrupt = 1; 00131 00132 osEvent evt = i2c_data_send.get(); 00133 if (evt.status == osEventMail) 00134 { 00135 i2c_data *i2c_data_s = (i2c_data*)evt.value.p; 00136 writedata = i2c_data_s -> data; 00137 t.stop(); 00138 //t3.start(); 00139 master_status_write = (bool) master.write(addr|0x00,&writedata,1); 00140 t3.stop(); 00141 if(master_status_write==0) 00142 { 00143 printf("master has written %c to slave\n\r",writedata); 00144 write2slave=false; 00145 } 00146 i2c_data_send.free(i2c_data_s); 00147 printf("\n%d\n",t.read_us()); 00148 t.reset(); 00149 printf("\n%d\n",t3.read_us()); 00150 t3.reset(); 00151 } 00152 interrupt = 0; 00153 } 00154 00155 00156 00157
Generated on Wed Aug 3 2022 14:14:45 by
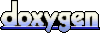