
hey
Dependencies: SDFileSystem mbed-rtos mbed
Fork of CDMS_BAE by
hk.cpp
00001 #include "hk.h" 00002 SPI spi(PTD6, PTD7, PTD5); 00003 DigitalOut cs(D6); 00004 00005 SDFileSystem sd(PTD6, PTD7, PTD5, D7,"sd"); 00006 00007 //Timer t1; 00008 //DigitalOut interrupt(D9); 00009 //const int addr = 0x20; 00010 bool ack0; 00011 //I2C master (D14,D15); 00012 00013 void spiwrite(int a) 00014 { 00015 cs=1; 00016 cs=0; 00017 spi.write(a); 00018 spi.write(0x01); 00019 } 00020 00021 int spiread(int a) 00022 { 00023 cs=1; 00024 cs=0; 00025 spi.write(a); 00026 return(spi.write(0x00)); 00027 } 00028 char* getname(int year1,int month1,int date1,int day1,int hours1,int minutes1,int seconds1) 00029 { 00030 year1= hexint(year1); 00031 month1=hexint(month1); 00032 date1=hexint(date1); 00033 day1=hexint(day1); 00034 hours1=hexint(hours1); 00035 minutes1=hexint(minutes1); 00036 seconds1=hexint(seconds1); 00037 char time[15]; 00038 sprintf(time,"%02d%02d%02d%02d%02d%02d%02d",year1,month1,date1,day1,hours1,minutes1,seconds1); 00039 00040 return(time); 00041 00042 } 00043 00044 int hexint(int a) 00045 { 00046 a=(a/16)*10+(a%16); 00047 return a; 00048 } 00049 //storedata stores the dummy structure in the file with timestamp as the filename in HK directory 00050 void init_rtc(void) 00051 { 00052 spi.format(8,3); 00053 spi.frequency(1000000); 00054 spiwrite(0x80); //write seconds to 01 00055 spiwrite(0x81); //write minutes t0 01 00056 spiwrite(0x82); //write hours to 01 00057 spiwrite(0x83); //write day of week to 01 00058 spiwrite(0x84); //write day of month to 01 00059 spiwrite(0x85); //write month to 01 00060 spiwrite(0x86); //write year to 01 00061 printf("RTC initialized"); 00062 } 00063 void storedata(char * data) 00064 { 00065 00066 printf("ready to store"); 00067 spi.format(8,3); 00068 spi.frequency(1000000); 00069 00070 int seconds=spiread(0x00); //read seconds 00071 int minutes =spiread(0x01); //read minutes 00072 int hours =spiread(0x02); //read hours 00073 int day =spiread(0x03); //read day of the week 00074 int date =spiread(0x04); //read day of the month 00075 int month =spiread(0x05); //read month 00076 int year =spiread(0x06); //read year 00077 cs = 1; 00078 00079 //Assigning dummy values to the structure 00080 00081 /* SensorData Sensor; 00082 00083 printf("Writing dummy values\n"); 00084 strcpy( Sensor.Voltage, "49"); 00085 strcpy( Sensor.Current, "83"); 00086 Sensor.Temperature ='5'; 00087 strcpy( Sensor.PanelTemperature, "4"); 00088 Sensor.Vcell_soc='9'; 00089 Sensor.alerts= '4'; 00090 Sensor.crate='7'; 00091 Sensor.BatteryTemperature='6'; 00092 Sensor.faultpoll='4'; 00093 Sensor.faultir='g'; 00094 Sensor.power_mode='k'; 00095 strcpy( Sensor.AngularSpeed, "9"); 00096 strcpy(Sensor.Bnewvalue,"6"); 00097 printf("Done writing dummy values\n"); */ 00098 mkdir("/sd/hk", 0777); 00099 char date2[100]="/sd/hk/"; 00100 strcat(date2,getname(year,month,date,day,hours,minutes,seconds)); 00101 strcat(date2,".txt"); 00102 FILE *fp ; 00103 fp= fopen(date2, "w"); 00104 if(fp == NULL) 00105 { 00106 error("Could not open file for write\n"); 00107 } 00108 else 00109 { 00110 fprintf(fp, "%s ", data); 00111 fclose(fp); 00112 printf("File created and data entered successfully"); 00113 } 00114 }
Generated on Wed Aug 3 2022 14:14:45 by
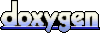