Library for H-Bridge Motor Driver Using Bipolar Transistors
Embed:
(wiki syntax)
Show/hide line numbers
motorlib.cpp
00001 /* mbed motorlib Library for H-Bridge Motor Driver Using Bipolar Transistors 00002 * Copyright (c) 2007-2012, Prabhu Desai http://mbed.org 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a copy 00005 * of this software and associated documentation files (the "Software"), to deal 00006 * in the Software without restriction, including without limitation the rights 00007 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 * copies of the Software, and to permit persons to whom the Software is 00009 * furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included in 00012 * all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 * THE SOFTWARE. 00021 */ 00022 00023 #include "motorlib.h" 00024 #include "mbed.h" 00025 00026 #ifdef LM293D_MOTOR_CTRL 00027 Motor::Motor(PinName r1, PinName r4, MCtrlType mctype): 00028 _r1(r1), _r4(r4),_mctype(mctype) { 00029 00030 _r1=0 ;_r4=0; 00031 00032 } 00033 00034 void Motor::coast(void) 00035 { 00036 if((_mctype == L293D_DISCRETE) || (_mctype == L293D_PWM)){ 00037 _r1=1 ;_r4=1; 00038 } 00039 } 00040 00041 void Motor::forward(void) 00042 { 00043 if(_mctype == L293D_PWM){ 00044 _r1=0 ; 00045 for(float p = 0.0f; p < 1.0f; p += 0.1f) { 00046 _r4 = p; 00047 wait(0.1); 00048 } 00049 }else if (_mctype == L293D_DISCRETE) { 00050 _r1=0 ;_r4 = 1; 00051 } 00052 00053 } 00054 void Motor::backward(void) 00055 { 00056 if (_mctype == L293D_PWM){ 00057 _r4=0 ; 00058 for(float p = 0.0f; p < 1.0f; p += 0.1f) { 00059 _r1 = p; 00060 wait(0.1); 00061 } 00062 }else if (_mctype == L293D_DISCRETE){ 00063 _r4=0 ;_r1 =1; 00064 } 00065 } 00066 00067 void Motor::stop(void) 00068 { 00069 _r1=1; _r4=1; 00070 } 00071 00072 #else 00073 Motor::Motor(PinName r1, PinName r2, PinName r3, PinName r4, MCtrlType mctype): 00074 _r1(r1), _r2(r2), _r3(r3), _r4(r4),_mctype(mctype) { 00075 _r1=1 ; _r2=1 ;_r3=1 ;_r4=1; 00076 } 00077 00078 void Motor::coast(void) 00079 { 00080 _r1=0 ; _r2=1 ;_r3=0;_r4=1; 00081 } 00082 00083 void Motor::forward(void) 00084 { 00085 _r1=0 ; _r2=0 ;_r3=1 ;_r4=1; 00086 00087 } 00088 00089 void Motor::backward(void) 00090 { 00091 _r1=1 ; _r2=1 ;_r3=0 ;_r4=0; 00092 } 00093 00094 void Motor::stop(void) 00095 { 00096 _r1=1 ; _r2=1 ;_r3=1 ;_r4=1; 00097 00098 } 00099 00100 #endif 00101 00102 /* 00103 //Test code for driving h-Bridge 00104 // Assume there are two motors connected to a chasis 00105 // a right and a left motor. 00106 00107 00108 Motor left_motor(LED1,LED2,LED3,LED4); 00109 Motor right_motor(LED1,LED2,LED3,LED4); 00110 00111 void main(){ 00112 //To drive the robot forward 00113 left_motor.forward(); 00114 right_motor.backward(); 00115 00116 // To drive the robot backward 00117 left_motor.backward(); 00118 right_motor.forward(); 00119 00120 // To turn the robot LEFT 00121 left_motor.stop(); 00122 right_motor.forward(); 00123 00124 // To turn the robot RIGHT 00125 left_motor.forward(); 00126 right_motor.stop(); 00127 00128 } 00129 00130 */
Generated on Tue Jul 12 2022 20:46:04 by
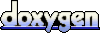