Fork from Dynamixel AX12 Servo for MX64 use and not-finishi now
Dependents: 2014-Mx64 2014-mx64-test
Fork of AX12 by
MX64.cpp
00001 /* mbed MX-64 Servo Library 00002 * 00003 * Copyright (c) 2010, cstyles (http://mbed.org) 00004 * 00005 * Permission is hereby granted, free of charge, to any person obtaining a copy 00006 * of this software and associated documentation files (the "Software"), to deal 00007 * in the Software without restriction, including without limitation the rights 00008 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00009 * copies of the Software, and to permit persons to whom the Software is 00010 * furnished to do so, subject to the following conditions: 00011 * 00012 * The above copyright notice and this permission notice shall be included in 00013 * all copies or substantial portions of the Software. 00014 * 00015 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00016 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00017 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00018 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00019 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00020 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00021 * THE SOFTWARE. 00022 */ 00023 00024 #include "MX64.h" 00025 #include "mbed.h" 00026 00027 MX64::MX64(PinName tx, PinName rx, int ID, int baud) 00028 : _mx64(tx,rx) { 00029 _baud = baud; 00030 _ID = ID; 00031 _mx64.baud(_baud); 00032 00033 } 00034 00035 // Set the mode of the servo 00036 // 0 = Positional (0-300 degrees) 00037 // 1 = Rotational -1 to 1 speed 00038 int MX64::SetMode(int mode) { 00039 00040 if (mode == 1) { // set CR 00041 SetCWLimit(0); 00042 SetCCWLimit(0); 00043 SetCRSpeed(0.0); 00044 } else { 00045 SetCWLimit(0); 00046 SetCCWLimit(300); 00047 SetCRSpeed(0.0); 00048 } 00049 return(0); 00050 } 00051 00052 00053 // if flag[0] is set, were blocking 00054 // if flag[1] is set, we're registering 00055 // they are mutually exclusive operations 00056 int MX64::SetGoal(int degrees, int flags) { 00057 00058 char reg_flag = 0; 00059 char data[2]; 00060 00061 // set the flag is only the register bit is set in the flag 00062 if (flags == 0x2) { 00063 reg_flag = 1; 00064 } 00065 00066 // 1023 / 300 * degrees 00067 short goal = (4095 * degrees) / 360; 00068 #ifdef MX64_DEBUG 00069 printf("SetGoal to 0x%x\n",goal); 00070 #endif 00071 00072 data[0] = goal & 0xff; // bottom 8 bits 00073 data[1] = goal >> 8; // top 8 bits 00074 00075 // write the packet, return the error code 00076 int rVal = write(_ID, MX64_REG_GOAL_POSITION, 2, data, reg_flag); 00077 00078 if (flags == 1) { 00079 // block until it comes to a halt 00080 while (isMoving()) {} 00081 } 00082 return(rVal); 00083 } 00084 00085 // if flag[0] is set, were blocking 00086 // if flag[1] is set, we're registering 00087 // they are mutually exclusive operations 00088 int MX64::SetGoalSpeed(int degrees, int speed, int flags) { 00089 00090 char reg_flag = 0; 00091 char data[4]; 00092 00093 // set the flag is only the register bit is set in the flag 00094 if (flags == 0x2) { 00095 reg_flag = 1; 00096 } 00097 00098 // 1023 / 300 * degrees 00099 short goal = (1023 * degrees) / 360; 00100 short sp = (1023 * speed) / 100; 00101 00102 #ifdef AX12_DEBUG 00103 printf("SetGoalSpeed to 0x%x with speed 0x%x\n",goal,sp); 00104 #endif 00105 00106 data[0] = goal & 0xff; // bottom 8 bits 00107 data[1] = goal >> 8; // top 8 bits 00108 00109 data[2] = sp & 0xff; 00110 data[3] = sp >> 8; 00111 00112 // write the packet, return the error code 00113 int rVal = write(_ID, MX64_REG_GOAL_POSITION, 4, data, reg_flag); 00114 00115 if (flags == 1) { 00116 // block until it comes to a halt 00117 while (isMoving()) {} 00118 } 00119 return(rVal); 00120 } 00121 00122 // Set continuous rotation speed from -1 to 1 00123 int MX64::SetCRSpeed(float speed) { 00124 00125 // bit 10 = direction, 0 = CCW, 1=CW 00126 // bits 9-0 = Speed 00127 char data[2]; 00128 00129 int goal = (0x3ff * abs(speed)); 00130 00131 // Set direction CW if we have a negative speed 00132 if (speed < 0) { 00133 goal |= (0x1 << 10); 00134 } 00135 00136 data[0] = goal & 0xff; // bottom 8 bits 00137 data[1] = goal >> 8; // top 8 bits 00138 00139 // write the packet, return the error code 00140 int rVal = write(_ID, 0x20, 2, data); 00141 00142 return(rVal); 00143 } 00144 00145 00146 int MX64::SetCWLimit (int degrees) { 00147 00148 char data[2]; 00149 00150 // 1023 / 300 * degrees 00151 short limit = (4095 * degrees) / 360; 00152 00153 #ifdef MX64_DEBUG 00154 printf("SetCWLimit to 0x%x\n",limit); 00155 #endif 00156 00157 data[0] = limit & 0xff; // bottom 8 bits 00158 data[1] = limit >> 8; // top 8 bits 00159 00160 // write the packet, return the error code 00161 return (write(_ID, MX64_REG_CW_LIMIT, 2, data)); 00162 00163 } 00164 00165 int MX64::SetCCWLimit (int degrees) { 00166 00167 char data[2]; 00168 00169 // 1023 / 300 * degrees 00170 short limit = (4095 * degrees) / 360; 00171 00172 #ifdef MX64_DEBUG 00173 printf("SetCCWLimit to 0x%x\n",limit); 00174 #endif 00175 00176 data[0] = limit & 0xff; // bottom 8 bits 00177 data[1] = limit >> 8; // top 8 bits 00178 00179 // write the packet, return the error code 00180 return (write(_ID, MX64_REG_CCW_LIMIT, 2, data)); 00181 } 00182 00183 00184 int MX64::SetID (int CurrentID, int NewID) { 00185 00186 char data[1]; 00187 data[0] = NewID; 00188 00189 #ifdef MX64_DEBUG 00190 printf("Setting ID from 0x%x to 0x%x\n",CurrentID,NewID); 00191 #endif 00192 00193 return (write(CurrentID, MX64_REG_ID, 1, data)); 00194 00195 } 00196 00197 00198 int MX64::SetBaud (int baud) { 00199 00200 char data[1]; 00201 data[0] = baud; 00202 00203 #ifdef MX64_DEBUG 00204 printf("Setting Baud rate to %d\n",baud); 00205 #endif 00206 00207 return (write(0xFE, MX64_REG_BAUD, 1, data)); 00208 00209 } 00210 00211 00212 00213 // return 1 is the servo is still in flight 00214 int MX64::isMoving(void) { 00215 00216 char data[1]; 00217 read(_ID,MX64_REG_MOVING,1,data); 00218 return(data[0]); 00219 } 00220 00221 00222 void MX64::trigger(void) { 00223 00224 char TxBuf[16]; 00225 char sum = 0; 00226 00227 #ifdef MX64_TRIGGER_DEBUG 00228 // Build the TxPacket first in RAM, then we'll send in one go 00229 printf("\nTriggered\n"); 00230 printf("\nTrigger Packet\n Header : 0xFF, 0xFF\n"); 00231 #endif 00232 00233 TxBuf[0] = 0xFF; 00234 TxBuf[1] = 0xFF; 00235 00236 // ID - Broadcast 00237 TxBuf[2] = 0xFE; 00238 sum += TxBuf[2]; 00239 00240 #ifdef MX64_TRIGGER_DEBUG 00241 printf(" ID : %d\n",TxBuf[2]); 00242 #endif 00243 00244 // Length 00245 TxBuf[3] = 0x02; 00246 sum += TxBuf[3]; 00247 00248 #ifdef MX64_TRIGGER_DEBUG 00249 printf(" Length %d\n",TxBuf[3]); 00250 #endif 00251 00252 // Instruction - ACTION 00253 TxBuf[4] = 0x04; 00254 sum += TxBuf[4]; 00255 00256 #ifdef MX64_TRIGGER_DEBUG 00257 printf(" Instruction 0x%X\n",TxBuf[5]); 00258 #endif 00259 00260 // Checksum 00261 TxBuf[5] = 0xFF - sum; 00262 #ifdef MX64_TRIGGER_DEBUG 00263 printf(" Checksum 0x%X\n",TxBuf[5]); 00264 #endif 00265 00266 // Transmit the packet in one burst with no pausing 00267 for (int i = 0; i < 6 ; i++) { 00268 _mx64.putc(TxBuf[i]); 00269 } 00270 00271 // This is a broadcast packet, so there will be no reply 00272 return; 00273 } 00274 00275 00276 float MX64::GetPosition(void) { 00277 00278 #ifdef MX64_DEBUG 00279 printf("\nGetPosition(%d)",_ID); 00280 #endif 00281 00282 char data[2]; 00283 00284 int ErrorCode = read(_ID, MX64_REG_POSITION, 2, data); 00285 short position = data[0] + (data[1] << 8); 00286 float angle = (position * 360)/4096; 00287 00288 return (angle); 00289 } 00290 00291 00292 float MX64::GetTemp (void) { 00293 00294 #ifdef MX64_DEBUG 00295 printf("\nGetTemp(%d)",_ID); 00296 #endif 00297 00298 char data[1]; 00299 int ErrorCode = read(_ID, MX64_REG_TEMP, 1, data); 00300 float temp = data[0]; 00301 return(temp); 00302 } 00303 00304 00305 float MX64::GetVolts (void) { 00306 00307 #ifdef MX64_DEBUG 00308 printf("\nGetVolts(%d)",_ID); 00309 #endif 00310 00311 char data[1]; 00312 int ErrorCode = read(_ID, MX64_REG_VOLTS, 1, data); 00313 float volts = data[0]/10.0; 00314 return(volts); 00315 } 00316 00317 00318 int MX64::read(int ID, int start, int bytes, char* data) { 00319 00320 char PacketLength = 0x4; 00321 char TxBuf[16]; 00322 char sum = 0; 00323 char Status[16]; 00324 00325 Status[4] = 0xFE; // return code 00326 00327 #ifdef MX64_READ_DEBUG 00328 printf("\nread(%d,0x%x,%d,data)\n",ID,start,bytes); 00329 #endif 00330 00331 // Build the TxPacket first in RAM, then we'll send in one go 00332 #ifdef MX64_READ_DEBUG 00333 printf("\nInstruction Packet\n Header : 0xFF, 0xFF\n"); 00334 #endif 00335 00336 TxBuf[0] = 0xff; 00337 TxBuf[1] = 0xff; 00338 00339 // ID 00340 TxBuf[2] = ID; 00341 sum += TxBuf[2]; 00342 00343 #ifdef MX64_READ_DEBUG 00344 printf(" ID : %d\n",TxBuf[2]); 00345 #endif 00346 00347 // Packet Length 00348 TxBuf[3] = PacketLength; // Length = 4 ; 2 + 1 (start) = 1 (bytes) 00349 sum += TxBuf[3]; // Accululate the packet sum 00350 00351 #ifdef MX64_READ_DEBUG 00352 printf(" Length : 0x%x\n",TxBuf[3]); 00353 #endif 00354 00355 // Instruction - Read 00356 TxBuf[4] = 0x2; 00357 sum += TxBuf[4]; 00358 00359 #ifdef MX64_READ_DEBUG 00360 printf(" Instruction : 0x%x\n",TxBuf[4]); 00361 #endif 00362 00363 // Start Address 00364 TxBuf[5] = start; 00365 sum += TxBuf[5]; 00366 00367 #ifdef MX64_READ_DEBUG 00368 printf(" Start Address : 0x%x\n",TxBuf[5]); 00369 #endif 00370 00371 // Bytes to read 00372 TxBuf[6] = bytes; 00373 sum += TxBuf[6]; 00374 00375 #ifdef MX64_READ_DEBUG 00376 printf(" No bytes : 0x%x\n",TxBuf[6]); 00377 #endif 00378 00379 // Checksum 00380 TxBuf[7] = 0xFF - sum; 00381 #ifdef MX64_READ_DEBUG 00382 printf(" Checksum : 0x%x\n",TxBuf[7]); 00383 #endif 00384 00385 // Transmit the packet in one burst with no pausing 00386 for (int i = 0; i<8 ; i++) { 00387 _mx64.putc(TxBuf[i]); 00388 } 00389 00390 // Wait for the bytes to be transmitted 00391 wait (0.00002); 00392 00393 // Skip if the read was to the broadcast address 00394 if (_ID != 0xFE) { 00395 00396 00397 00398 // response packet is always 6 + bytes 00399 // 0xFF, 0xFF, ID, Length Error, Param(s) Checksum 00400 // timeout is a little more than the time to transmit 00401 // the packet back, i.e. (6+bytes)*10 bit periods 00402 00403 int timeout = 0; 00404 int plen = 0; 00405 while ((timeout < ((6+bytes)*10)) && (plen<(6+bytes))) { 00406 00407 if (_mx64.readable()) { 00408 Status[plen] = _mx64.getc(); 00409 plen++; 00410 timeout = 0; 00411 } 00412 00413 // wait for the bit period 00414 wait (1.0/_baud); 00415 timeout++; 00416 } 00417 00418 if (timeout == ((6+bytes)*10) ) { 00419 return(-1); 00420 } 00421 00422 // Copy the data from Status into data for return 00423 for (int i=0; i < Status[3]-2 ; i++) { 00424 data[i] = Status[5+i]; 00425 } 00426 00427 #ifdef MX64_READ_DEBUG 00428 printf("\nStatus Packet\n"); 00429 printf(" Header : 0x%x\n",Status[0]); 00430 printf(" Header : 0x%x\n",Status[1]); 00431 printf(" ID : 0x%x\n",Status[2]); 00432 printf(" Length : 0x%x\n",Status[3]); 00433 printf(" Error Code : 0x%x\n",Status[4]); 00434 00435 for (int i=0; i < Status[3]-2 ; i++) { 00436 printf(" Data : 0x%x\n",Status[5+i]); 00437 } 00438 00439 printf(" Checksum : 0x%x\n",Status[5+(Status[3]-2)]); 00440 #endif 00441 00442 } // if (ID!=0xFE) 00443 00444 return(Status[4]); 00445 } 00446 00447 00448 int MX64::write(int ID, int start, int bytes, char* data, int flag) { 00449 // 0xff, 0xff, ID, Length, Intruction(write), Address, Param(s), Checksum 00450 00451 char TxBuf[16]; 00452 char sum = 0; 00453 char Status[6]; 00454 00455 #ifdef MX64_WRITE_DEBUG 00456 printf("\nwrite(%d,0x%x,%d,data,%d)\n",ID,start,bytes,flag); 00457 #endif 00458 00459 // Build the TxPacket first in RAM, then we'll send in one go 00460 #ifdef MX64_WRITE_DEBUG 00461 printf("\nInstruction Packet\n Header : 0xFF, 0xFF\n"); 00462 #endif 00463 00464 TxBuf[0] = 0xff; 00465 TxBuf[1] = 0xff; 00466 00467 // ID 00468 TxBuf[2] = ID; 00469 sum += TxBuf[2]; 00470 00471 #ifdef MX64_WRITE_DEBUG 00472 printf(" ID : %d\n",TxBuf[2]); 00473 #endif 00474 00475 // packet Length 00476 TxBuf[3] = 3+bytes; 00477 sum += TxBuf[3]; 00478 00479 #ifdef MX64_WRITE_DEBUG 00480 printf(" Length : %d\n",TxBuf[3]); 00481 #endif 00482 00483 // Instruction 00484 if (flag == 1) { 00485 TxBuf[4]=0x04; 00486 sum += TxBuf[4]; 00487 } else { 00488 TxBuf[4]=0x03; 00489 sum += TxBuf[4]; 00490 } 00491 00492 #ifdef MX64_WRITE_DEBUG 00493 printf(" Instruction : 0x%x\n",TxBuf[4]); 00494 #endif 00495 00496 // Start Address 00497 TxBuf[5] = start; 00498 sum += TxBuf[5]; 00499 00500 #ifdef MX64_WRITE_DEBUG 00501 printf(" Start : 0x%x\n",TxBuf[5]); 00502 #endif 00503 00504 // data 00505 for (char i=0; i<bytes ; i++) { 00506 TxBuf[6+i] = data[i]; 00507 sum += TxBuf[6+i]; 00508 00509 #ifdef MX64_WRITE_DEBUG 00510 printf(" Data : 0x%x\n",TxBuf[6+i]); 00511 #endif 00512 00513 } 00514 00515 // checksum 00516 TxBuf[6+bytes] = 0xFF - sum; 00517 00518 #ifdef MX64_WRITE_DEBUG 00519 printf(" Checksum : 0x%x\n",TxBuf[6+bytes]); 00520 #endif 00521 00522 // Transmit the packet in one burst with no pausing 00523 for (int i = 0; i < (7 + bytes) ; i++) { 00524 _mx64.putc(TxBuf[i]); 00525 } 00526 00527 // Wait for data to transmit 00528 wait (0.00002); 00529 00530 // make sure we have a valid return 00531 Status[4]=0x00; 00532 00533 // we'll only get a reply if it was not broadcast 00534 if (_ID!=0xFE) { 00535 00536 00537 // response packet is always 6 bytes 00538 // 0xFF, 0xFF, ID, Length Error, Param(s) Checksum 00539 // timeout is a little more than the time to transmit 00540 // the packet back, i.e. 60 bit periods, round up to 100 00541 int timeout = 0; 00542 int plen = 0; 00543 while ((timeout < 100) && (plen<6)) { 00544 00545 if (_mx64.readable()) { 00546 Status[plen] = _mx64.getc(); 00547 plen++; 00548 timeout = 0; 00549 } 00550 00551 // wait for the bit period 00552 wait (1.0/_baud); 00553 timeout++; 00554 } 00555 00556 00557 // Build the TxPacket first in RAM, then we'll send in one go 00558 #ifdef MX64_WRITE_DEBUG 00559 printf("\nStatus Packet\n Header : 0x%X, 0x%X\n",Status[0],Status[1]); 00560 printf(" ID : %d\n",Status[2]); 00561 printf(" Length : %d\n",Status[3]); 00562 printf(" Error : 0x%x\n",Status[4]); 00563 printf(" Checksum : 0x%x\n",Status[5]); 00564 #endif 00565 00566 00567 } 00568 00569 return(Status[4]); // return error code 00570 }
Generated on Fri Jul 22 2022 04:49:02 by
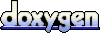