
Remote monitoring IoTHub device sample Added real SHTx sensor to get temperature and humidity Added new command to set temperature scale change Removed "mock" commands to set temperature and humidity values It's configured to work on FRDM-K64F board
Dependencies: EthernetInterface NTPClient SHTx iothub_amqp_transport iothub_client mbed-rtos mbed proton-c-mbed serializer wolfSSL
Fork of remote_monitoring by
main.cpp
00001 // Copyright (c) Microsoft. All rights reserved. 00002 // Licensed under the MIT license. See LICENSE file in the project root for full license information. 00003 00004 #include <stdio.h> 00005 #include "EthernetInterface.h" 00006 #include "mbed/logging.h" 00007 #include "mbed/mbedtime.h" 00008 #include "remote_monitoring.h" 00009 #include "NTPClient.h" 00010 00011 int setupRealTime(void) 00012 { 00013 int result; 00014 00015 (void)printf("setupRealTime begin\r\n"); 00016 if (EthernetInterface::connect()) 00017 { 00018 (void)printf("Error initializing EthernetInterface.\r\n"); 00019 result = __LINE__; 00020 } 00021 else 00022 { 00023 (void)printf("setupRealTime NTP begin\r\n"); 00024 NTPClient ntp; 00025 if (ntp.setTime("0.pool.ntp.org") != 0) 00026 { 00027 (void)printf("Failed setting time.\r\n"); 00028 result = __LINE__; 00029 } 00030 else 00031 { 00032 (void)printf("set time correctly!\r\n"); 00033 result = 0; 00034 } 00035 (void)printf("setupRealTime NTP end\r\n"); 00036 EthernetInterface::disconnect(); 00037 } 00038 (void)printf("setupRealTime end\r\n"); 00039 00040 return result; 00041 } 00042 00043 int main(void) 00044 { 00045 (void)printf("Initializing mbed specific things...\r\n"); 00046 00047 /* These are needed in order to initialize the time provider for Proton-C */ 00048 mbed_log_init(); 00049 mbedtime_init(); 00050 00051 if (EthernetInterface::init()) 00052 { 00053 (void)printf("Error initializing EthernetInterface.\r\n"); 00054 return -1; 00055 } 00056 00057 if (setupRealTime() != 0) 00058 { 00059 (void)printf("Failed setting up real time clock\r\n"); 00060 return -1; 00061 } 00062 00063 if (EthernetInterface::connect()) 00064 { 00065 (void)printf("Error connecting EthernetInterface.\r\n"); 00066 return -1; 00067 } 00068 00069 remote_monitoring_run(); 00070 00071 (void)EthernetInterface::disconnect(); 00072 00073 return 0; 00074 }
Generated on Fri Jul 15 2022 22:00:23 by
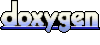