
adding resources firmware and 1/0/8
Dependencies: Beep C12832_lcd EthernetInterface EthernetNetIf HTTPClient LM75B MMA7660 mbed-rtos mbed nsdl_lib
Fork of LWM2M_NanoService_Ethernet by
main.cpp
00001 //Hello Pascal, 00002 00003 //to be able to support MDM in a clustered environment and permanent tcp connection with devices, we had to restrict the supported lwm2m scenario’s to the queued mode only. 00004 //This because jobs launched via the MDM console could be executed by a MDM node of the cluster that doesn’t have a tcp connection with the device. 00005 //With the latest lwm2m plugin, it is up to the device to come to the plugin with a lwm2m Register Update on one of the node and the Job waiting in shared MDM queue will execute. 00006 00007 //To show demo’s this is a bit embarrassing since updates are sent every time device lifetime expires and this could be long. 00008 00009 //So what we have introduced is the ability for the lwm2m plugin to send a Registration Update Trigger to the device if it has an available connection to it. 00010 //In a single node environment this is working fine. 00011 00012 //Can you please check if this registration update trigger can be implemented in the NXP and the freescale so we could upgrade our plugin when required. 00013 //All what is needed is to send a registration update message when then device receive an execute on resource 1/0/8. 00014 00015 00016 00017 00018 #include "mbed.h" 00019 #include "EthernetInterface.h" 00020 #include "C12832_lcd.h" 00021 #include "nsdl_support.h" 00022 #include "dbg.h" 00023 // Include various resources 00024 #include "temperature.h" 00025 #include "light.h" 00026 #include "gps.h" 00027 #include "relay.h" 00028 #include "rgb.h" 00029 00030 #include "firmware.h" 00031 #include "firmware_result.h" 00032 #include "firmware_status.h" 00033 00034 #include "register_upd_trigger.h" 00035 00036 static C12832_LCD lcd; 00037 static PwmOut led1(LED1); 00038 static PwmOut led2(LED2); 00039 static PwmOut led3(LED3); 00040 static PwmOut led4(LED4); 00041 00042 Serial pc(USBTX, USBRX); // tx, rx 00043 00044 // **************************************************************************** 00045 // Configuration section 00046 00047 // Ethernet configuration 00048 /* Define this to enable DHCP, otherwise manual address configuration is used */ 00049 #define DHCP 1 00050 00051 // Define this to enable the RGB LED resource 00052 //#define USE_RGBLED 1 00053 00054 /* Manual IP configurations, if DHCP not defined */ 00055 #define IP "10.45.0.206" 00056 #define MASK "255.255.255.0" 00057 #define GW "10.45.0.1" 00058 00059 // UDM configuration 00060 /* Change this IP address to that of your NanoService Platform installation */ 00061 //static const char* UDM_ADDRESS = "137.135.13.28"; /* demo UDM, web interface at http://red-hat-summit.cloudapp.net*/ 00062 //static const char* UDM_ADDRESS = "137.117.164.225"; /* m2mcc02 */ 00063 static const char* UDM_ADDRESS = "68.235.31.32"; 00064 static const int UDM_PORT = 5684; 00065 //char endpoint_name[20] = "ntcmbed-ethernet-"; 00066 char endpoint_name[20] = "NXP1768-"; 00067 char mbed_uid[33]; // for creating unique name for the board 00068 uint8_t ep_type[] = {"mbed_lpc1768_appboard"}; 00069 uint8_t lifetime_ptr[] = {"60"}; 00070 00071 static const char* FIRMWARE_VER = "18"; // Committed revision number 00072 char* mac; 00073 char* ipAddr; 00074 char* gateway; 00075 char* nmask; 00076 00077 // **************************************************************************** 00078 // Ethernet initialization 00079 00080 EthernetInterface eth; 00081 00082 static void ethernet_init() 00083 { 00084 00085 /* Initialize network */ 00086 #ifdef DHCP 00087 NSDL_DEBUG("DHCP in use"); 00088 eth.init(); 00089 #else 00090 eth.init(IP, MASK, GW); 00091 #endif 00092 if(eth.connect(60000) == 0) 00093 NSDL_DEBUG("Ethernet up"); 00094 00095 mac = eth.getMACAddress(); 00096 ipAddr = eth.getIPAddress(); 00097 gateway = eth.getGateway(); 00098 nmask = eth.getNetworkMask(); 00099 NSDL_DEBUG("Network: mac=%s, ip=%s, gateway=%s, mask=%s", mac, ipAddr, gateway, nmask); 00100 00101 mbed_interface_uid(mbed_uid); 00102 mbed_uid[32] = '\0'; 00103 00104 NSDL_DEBUG("Full interface uid=%s", mbed_uid); 00105 strncat(endpoint_name, mbed_uid + 27, 20 - strlen(endpoint_name)); 00106 00107 // EP_NAME = (char*) malloc(strlen(endpoint_name)+1); 00108 // strcpy(EP_NAME, endpoint_name); 00109 00110 lcd.locate(0,11); 00111 lcd.printf("IP:%s", eth.getIPAddress()); 00112 00113 } 00114 00115 // **************************************************************************** 00116 // UDM initialization 00117 00118 UDPSocket server; 00119 Endpoint udm; 00120 00121 static void udm_init() 00122 { 00123 server.init(); 00124 server.bind(UDM_PORT); 00125 00126 udm.set_address(UDM_ADDRESS, UDM_PORT); 00127 00128 NSDL_DEBUG("name: %s", endpoint_name); 00129 NSDL_DEBUG("UDM=%s - port %d\n", UDM_ADDRESS, UDM_PORT); 00130 00131 lcd.locate(0,22); 00132 lcd.printf("EP:%s\n", endpoint_name); 00133 } 00134 00135 // **************************************************************************** 00136 // Resource creation 00137 00138 static int create_resources() 00139 { 00140 sn_nsdl_resource_info_s *resource_ptr = NULL; 00141 sn_nsdl_ep_parameters_s *endpoint_ptr = NULL; 00142 00143 NSDL_DEBUG("Creating resources"); 00144 00145 /* Create resources */ 00146 resource_ptr = (sn_nsdl_resource_info_s*)nsdl_alloc(sizeof(sn_nsdl_resource_info_s)); 00147 if(!resource_ptr) 00148 return 0; 00149 memset(resource_ptr, 0, sizeof(sn_nsdl_resource_info_s)); 00150 00151 resource_ptr->resource_parameters_ptr = (sn_nsdl_resource_parameters_s*)nsdl_alloc(sizeof(sn_nsdl_resource_parameters_s)); 00152 if(!resource_ptr->resource_parameters_ptr) 00153 { 00154 nsdl_free(resource_ptr); 00155 return 0; 00156 } 00157 memset(resource_ptr->resource_parameters_ptr, 0, sizeof(sn_nsdl_resource_parameters_s)); 00158 00159 // Static resources 00160 nsdl_create_static_resource(resource_ptr, sizeof("3/0/0")-1, (uint8_t*) "3/0/0", 0, 0, (uint8_t*) "ARM", sizeof("ARM")-1); 00161 nsdl_create_static_resource(resource_ptr, sizeof("3/0/1")-1, (uint8_t*) "3/0/1", 0, 0, (uint8_t*) "LPC1768 App Board", sizeof("LPC1768 App Board")-1); 00162 nsdl_create_static_resource(resource_ptr, sizeof("3/0/2")-1, (uint8_t*) "3/0/2", 0, 0, (uint8_t*) mbed_uid, sizeof(mbed_uid)-1); 00163 nsdl_create_static_resource(resource_ptr, sizeof("3/0/3")-1, (uint8_t*) "3/0/3", 0, 0, (uint8_t*) FIRMWARE_VER, strlen(FIRMWARE_VER)); 00164 #ifdef DHCP 00165 nsdl_create_static_resource(resource_ptr, sizeof("4/0/0")-1, (uint8_t*) "4/0/0", 0, 0, (uint8_t*) "Ethernet DHCP", sizeof("Ethernet DHCP")-1); 00166 #else 00167 nsdl_create_static_resource(resource_ptr, sizeof("4/0/0")-1, (uint8_t*) "4/0/0", 0, 0, (uint8_t*) "Ethernet Static", sizeof("Ethernet Static")-1); 00168 #endif 00169 nsdl_create_static_resource(resource_ptr, sizeof("4/0/1")-1, (uint8_t*) "4/0/1", 0, 0, (uint8_t*) "Ethernet (Static, DHCP)", sizeof("Ethernet (Static, DHCP)")-1); 00170 nsdl_create_static_resource(resource_ptr, sizeof("4/0/4")-1, (uint8_t*) "4/0/4", 0, 0, (uint8_t*) ipAddr, strlen(ipAddr)); 00171 nsdl_create_static_resource(resource_ptr, sizeof("4/0/5")-1, (uint8_t*) "4/0/5", 0, 0, (uint8_t*) gateway, strlen(gateway)); 00172 nsdl_create_static_resource(resource_ptr, sizeof("3/0/16")-1, (uint8_t*) "3/0/16", 0, 0, (uint8_t*) "UDP", sizeof("UDP")-1); 00173 00174 // Dynamic resources 00175 create_temperature_resource(resource_ptr); 00176 create_light_resource(resource_ptr); 00177 create_gps_resource(resource_ptr); 00178 create_relay_resource(resource_ptr); 00179 create_register_upd_trigger_resource(resource_ptr); 00180 00181 // Dynamic Firmware resources 00182 create_firmware_resource(resource_ptr); 00183 create_firmware_result_resource(resource_ptr); 00184 create_firmware_status_resource(resource_ptr); 00185 00186 #ifdef USE_RGBLED 00187 NSDL_DEBUG("Enabling RGB LED due to USE_RGBLED=%d\n", USE_RGBLED); 00188 create_rgb_resource(resource_ptr); 00189 #else 00190 NSDL_DEBUG("Skipped RGB LED resource, change USE_RGBLED to 1 in main.cpp to test"); 00191 #endif 00192 00193 /* Register with UDM */ 00194 endpoint_ptr = nsdl_init_register_endpoint(endpoint_ptr, (uint8_t*)endpoint_name, ep_type, lifetime_ptr); 00195 if(sn_nsdl_register_endpoint(endpoint_ptr) != 0) 00196 pc.printf("UDM registering failed\r\n"); 00197 else 00198 pc.printf("UDM registering OK\r\n"); 00199 nsdl_clean_register_endpoint(&endpoint_ptr); 00200 00201 nsdl_free(resource_ptr->resource_parameters_ptr); 00202 nsdl_free(resource_ptr); 00203 return 1; 00204 } 00205 00206 // **************************************************************************** 00207 // Program entry point 00208 00209 int main() 00210 { 00211 // strcpy((char*) ep_type, "mbed_lpc1768_appboard"); 00212 strcpy((char*) lifetime_ptr, "60"); 00213 00214 lcd.cls(); 00215 lcd.locate(0,0); 00216 lcd.printf("NanoService LWM2M v%s", FIRMWARE_VER); 00217 NSDL_DEBUG("NanoService LWM2M Demo for LPC1768 App Board\n"); 00218 NSDL_DEBUG("NanoService LWM2M version %s\n", FIRMWARE_VER); 00219 00220 // Initialize Ethernet interface first 00221 ethernet_init(); 00222 00223 // Initialize UDM node 00224 udm_init(); 00225 00226 // Initialize NSDL stack 00227 nsdl_init(); 00228 00229 // Create NSDL resources 00230 create_resources(); 00231 00232 //Version 18 00233 led3.write(0.1); 00234 00235 //Version 19 00236 // led2.write(0.5); 00237 // led3.write(0.5); 00238 00239 // Run the NSDL event loop (never returns) 00240 nsdl_event_loop(); 00241 }
Generated on Sat Jul 16 2022 02:03:09 by
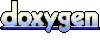