mbed library sources. Supersedes mbed-src.
Fork of mbed-dev by
Timer Class Reference
[Drivers]
A general purpose timer. More...
#include <Timer.h>
Inherits NonCopyable< Timer >.
Inherited by LowPowerTimer.
Public Member Functions | |
void | start () |
Start the timer. | |
void | stop () |
Stop the timer. | |
void | reset () |
Reset the timer to 0. | |
float | read () |
Get the time passed in seconds. | |
int | read_ms () |
Get the time passed in milli-seconds. | |
int | read_us () |
Get the time passed in micro-seconds. | |
operator float () | |
An operator shorthand for read() | |
us_timestamp_t | read_high_resolution_us () |
Get in a high resolution type the time passed in micro-seconds. | |
Private Member Functions | |
MBED_DEPRECATED ("Invalid copy construction of a NonCopyable resource.") NonCopyable(const NonCopyable &) | |
NonCopyable copy constructor. | |
MBED_DEPRECATED ("Invalid copy assignment of a NonCopyable resource.") NonCopyable &operator | |
NonCopyable copy assignment operator. |
Detailed Description
A general purpose timer.
- Note:
- Synchronization level: Interrupt safe
Example:
// Count the time to toggle a LED #include "mbed.h" Timer timer; DigitalOut led(LED1); int begin, end; int main() { timer.start(); begin = timer.read_us(); led = !led; end = timer.read_us(); printf("Toggle the led takes %d us", end - begin); }
Definition at line 51 of file Timer.h.
Member Function Documentation
float read | ( | void | ) |
us_timestamp_t read_high_resolution_us | ( | ) |
int read_ms | ( | ) |
int read_us | ( | ) |
void reset | ( | ) |
Generated on Tue Jul 12 2022 20:37:48 by
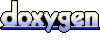