
Code for Player 2 of Rock Paper Scissors
Dependencies: 4DGL-uLCD-SE PinDetect SDFileSystem mbed wave_player
main.cpp
00001 //ECE 4180 Mini Project 00002 //Prachi Kulkarni and Kendra Dodson 00003 00004 //This code is for Player 2 00005 00006 #include "mbed.h" 00007 #include "uLCD_4DGL.h" 00008 #include "SDFileSystem.h" 00009 #include "wave_player.h" 00010 00011 //Leds to show status of sending and receiving messages 00012 DigitalOut myled(LED1); 00013 DigitalOut myled2(LED2); 00014 DigitalOut myled3(LED3); 00015 00016 SDFileSystem sd(p5, p6, p7, p8, "sd"); 00017 AnalogOut DACout(p18); 00018 wave_player waver(&DACout); // Wave Player 00019 00020 uLCD_4DGL lcd(p28, p27, p29); //uLCD 00021 DigitalIn pb1(p17); //Pushbutton 1 00022 DigitalIn pb2(p19); //Pushbutton 2 00023 DigitalIn pb3(p20); //Pushbutton 3 00024 00025 //Shiftbrite 00026 DigitalOut latch(p15); 00027 DigitalOut enable(p16); 00028 SPI spi(p11, p12, p13); 00029 00030 //Choice 00031 //Choice = 1 for rock 00032 //Choice = 2 for paper 00033 //Choice = 3 for scissors 00034 char choice1; //Player 1's choice 00035 char choice2; //Player 2's choice 00036 00037 //Points 00038 int p1 = 0; //Player 1's points 00039 int p2 = 0; //Player 2's points 00040 00041 //RGB function for Shiftbrite 00042 void RGB_LED(int red, int green, int blue); 00043 void RGB_LED(int red, int green, int blue) 00044 { 00045 unsigned int low_color=0; 00046 unsigned int high_color=0; 00047 high_color=(blue<<4)|((red&0x3C0)>>6); 00048 low_color=(((red&0x3F)<<10)|(green)); 00049 spi.write(high_color); 00050 spi.write(low_color); 00051 latch=1; 00052 latch=0; 00053 } 00054 00055 //Serial 00056 Serial player2(p9,p10); 00057 00058 int main() { 00059 00060 //Serial 00061 player2.baud(9600); 00062 00063 spi.format(16,0); 00064 spi.frequency(500000); 00065 enable=0; 00066 latch=0; 00067 pb1.mode(PullUp); 00068 wait(0.001); 00069 pb2.mode(PullUp); 00070 wait(0.001); 00071 pb3.mode(PullUp); 00072 wait(0.001); 00073 00074 //Display 00075 00076 lcd.locate(1,2); 00077 //Screen 1 shows the name of the game 00078 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00079 lcd.color(BLUE); //Set font color to blue 00080 lcd.text_bold(ON); //Bold the text 00081 lcd.text_width(2); 00082 lcd.text_height(2); 00083 lcd.printf(" Rock\n"); 00084 lcd.printf(" Paper\n\n"); 00085 lcd.printf(" Scissor\n\n"); 00086 //DACout.beep(1000,0.5); 00087 wait(5); 00088 00089 //Screen 2 shows pushbutton options 00090 L1: 00091 lcd.cls(); 00092 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00093 lcd.color(GREEN); //Set font color to green 00094 lcd.locate(0,2); 00095 lcd.printf(" Button Options\n\n"); 00096 lcd.locate(0,5); 00097 lcd.text_underline(OFF); 00098 lcd.printf(" 1 for Rocks"); 00099 lcd.locate(0,7); 00100 lcd.printf(" 2 for Paper"); 00101 lcd.locate(0,9); 00102 lcd.printf(" 3 for Scissors\n\n\n"); 00103 lcd.text_bold(ON); //Bold the text 00104 lcd.printf(" Press 1 to start!"); 00105 wait(1); 00106 00107 while(1){ 00108 //If push button 1 is pressed, start the game! 00109 if(pb1 != 1){ 00110 00111 00112 //Play button select 00113 FILE *wave_file; 00114 printf("\n\n\nHello, wave world!\n"); 00115 wave_file=fopen("/sd/mydir/select.wav","r"); 00116 waver.play(wave_file); 00117 wait(1); 00118 printf("ok!!"); 00119 fclose(wave_file); 00120 00121 00122 L3: 00123 lcd.cls(); //Clear screen 00124 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00125 //Tell player 1 that she/he has 5 seconds to chose between rock,paper and scissors 00126 lcd.locate(0,4); 00127 lcd.color(BLUE); //Set font color to yellow 00128 lcd.printf(" Player 2 \n\n\n"); 00129 lcd.locate(0,6); 00130 lcd.color(GREEN); //Set font color to green 00131 lcd.printf("You have 5 seconds"); 00132 lcd.printf(" to choose\n"); 00133 lcd.printf(" an option!\n"); 00134 wait(5); 00135 00136 00137 //Start timer for 5 seconds 00138 int i = 5;; 00139 int red = 1; 00140 //for(i = 5; i > -1 ; i--){ 00141 while(i > -1){ 00142 00143 lcd.cls(); 00144 lcd.locate(4,4); //Place text in the center of the screen 00145 lcd.text_width(8); //Set tet width 00146 lcd.text_height(8); //Set text height 00147 lcd.text_bold(ON); //Set text style to 'bold' 00148 RGB_LED(red*25,0,0);//Increase intensity of light on shiftbrite as time decreases 00149 red = red + 5; 00150 lcd.printf("%d",i); //Display time left on LCD 00151 wait(1); 00152 //Press pushbutton 1 to choose rock 00153 if(pb1 != 1) { 00154 00155 lcd.cls(); 00156 choice2 = 'R'; 00157 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00158 lcd.locate(0,1); 00159 lcd.printf(" You chose rock"); 00160 00161 //Play button select 00162 FILE *wave_file; 00163 printf("\n\n\nHello, wave world!\n"); 00164 wave_file=fopen("/sd/mydir/select.wav","r"); 00165 waver.play(wave_file); 00166 wait(1); 00167 printf("ok!!"); 00168 fclose(wave_file); 00169 00170 lcd.circle(60, 60, 30,WHITE); 00171 wait(3); 00172 lcd.cls(); 00173 break; 00174 } 00175 //Press pushbutton 2 to choose paper 00176 else if(pb2 != 1) { 00177 lcd.cls(); 00178 choice2 = 'P'; 00179 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00180 00181 lcd.locate(0,1); 00182 lcd.printf(" You chose paper"); 00183 00184 //Play button select 00185 FILE *wave_file; 00186 printf("\n\n\nHello, wave world!\n"); 00187 wave_file=fopen("/sd/mydir/select.wav","r"); 00188 waver.play(wave_file); 00189 00190 printf("ok!!"); 00191 fclose(wave_file); 00192 00193 lcd.filled_rectangle(50, 45, 80,90,WHITE); 00194 wait(3); 00195 lcd.cls(); 00196 break; 00197 } 00198 //Press pushbutton 3 to choose scissor 00199 else if(pb3 != 1) { 00200 lcd.cls(); 00201 choice2 = 'S'; 00202 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00203 lcd.locate(0,1); 00204 lcd.printf("You chose scissors"); 00205 //Play button select 00206 FILE *wave_file; 00207 printf("\n\n\nHello, wave world!\n"); 00208 wave_file=fopen("/sd/mydir/select.wav","r"); 00209 waver.play(wave_file); 00210 wait(1); 00211 printf("ok!!"); 00212 fclose(wave_file); 00213 lcd.circle(50, 60, 10,WHITE); 00214 lcd.circle(50, 80, 10,WHITE); 00215 lcd.line(62, 61, 90, 85, WHITE); 00216 lcd.line(62, 81, 90, 55, WHITE); 00217 wait(3); 00218 lcd.cls(); 00219 break; 00220 } 00221 i--; 00222 00223 } 00224 RGB_LED(0,0,0); // Reset shitbrite color 00225 00226 //------------------------------------------------------------------------------------------------------------------------------------- 00227 // TIME-OUT 00228 //------------------------------------------------------------------------------------------------------------------------------------ 00229 00230 //If user doesn't choose an option, show "Time Out" message and restart game 00231 if(i == -1){ 00232 lcd.cls(); 00233 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00234 lcd.locate(0,5); 00235 lcd.color(RED); 00236 lcd.printf(" Your time is up!"); 00237 lcd.color(GREEN); 00238 //Play time up tune 00239 FILE *wave_file; 00240 printf("\n\n\nHello, wave world!\n"); 00241 wave_file=fopen("/sd/mydir/timeup.wav","r"); 00242 waver.play(wave_file); 00243 00244 printf("ok!!"); 00245 fclose(wave_file); 00246 wait(3); 00247 goto L1; //Starts the game again 00248 } 00249 00250 //-------------------------------------------------------------------------------------------------------------------------------------- 00251 00252 //Send player 2's choice to player 1 00253 player2.printf("%c",choice2); 00254 myled2 = 1; 00255 wait(1); 00256 00257 //Receive Player 1's choice 00258 while(1){ 00259 lcd.locate(1,4); 00260 lcd.printf(" Waiting for \n the other player"); 00261 if(player2.readable()){ 00262 choice1 = player2.getc(); 00263 myled = 1; 00264 break; 00265 } 00266 } 00267 00268 00269 //------------------------------------------------------------------------------------------------------------------------------------- 00270 //Check to see who won 00271 //-------------------------------------------------------------------------------------------------------------------------- 00272 //Case 1 : Both players choose same option 00273 if(choice1 == 'R' && choice2 == 'R' || 00274 choice1== 'P' && choice2 == 'P' || 00275 choice1 == 'S' && choice2 == 'S' ) { 00276 lcd.cls(); 00277 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00278 lcd.locate(1,2); 00279 lcd.printf(" It's a draw!"); 00280 wait(4); 00281 } 00282 //Case 2: 00283 //Player 1 : Rock 00284 //Player 2 : Paper 00285 else if(choice1 == 'R' && choice2 == 'P'){ 00286 00287 lcd.cls(); 00288 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00289 00290 lcd.locate(1,2); 00291 lcd.printf(" P1 chose Rock\n"); 00292 lcd.printf(" P2 chose Paper\n\n\n\n"); 00293 lcd.locate(1,5); 00294 lcd.printf(" Player 2 wins!"); 00295 lcd.filled_rectangle(50, 60, 80,90,WHITE); 00296 p2++; 00297 wait(4); 00298 } 00299 //Case 3: 00300 //Player 1 : Paper 00301 //Player 2 : Rock 00302 else if(choice2== 'P' && choice1== 'R'){ 00303 00304 lcd.cls(); 00305 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00306 lcd.locate(1,2); 00307 lcd.printf("P1 chose Paper\n"); 00308 lcd.printf(" P2 chose Rock\n\n"); 00309 lcd.locate(1,5); 00310 lcd.printf("P1 wins!"); 00311 lcd.filled_rectangle(50, 60, 80,90,WHITE); 00312 p1++; 00313 wait(5); 00314 } 00315 00316 //Case 4: 00317 //Player 1 : Paper 00318 //Player 2 : Scissor 00319 00320 else if(choice1 == 'P' && choice2 == 'S'){ 00321 00322 lcd.cls(); 00323 lcd.locate(1,1); 00324 lcd.printf("P1 chose Paper\n"); 00325 lcd.printf(" P2 chose Scissors\n\n"); 00326 lcd.locate(1,4); 00327 lcd.printf("P2 wins!"); 00328 p2++; 00329 lcd.circle(50, 60, 10,WHITE); 00330 lcd.circle(50, 80, 10,WHITE); 00331 lcd.line(62, 61, 90, 85, WHITE); 00332 lcd.line(62, 81, 90, 55, WHITE); 00333 wait(5); 00334 00335 } 00336 //Case 5: 00337 //Player 1 : Scissor 00338 //Player 2 : Paper 00339 else if(choice1 == 'S' && choice2 == 'P'){ 00340 00341 lcd.cls(); 00342 lcd.locate(1,1); 00343 lcd.printf("P1 chose Scissors\n"); 00344 lcd.printf(" P2 chose Paper\n\n"); 00345 lcd.locate(1,4); 00346 lcd.printf("P1 wins!"); 00347 p1++; 00348 lcd.circle(50, 60, 10,WHITE); 00349 lcd.circle(50, 80, 10,WHITE); 00350 lcd.line(62, 61, 90, 85, WHITE); 00351 lcd.line(62, 81, 90, 55, WHITE); 00352 wait(5); 00353 00354 } 00355 //Case 6: 00356 //Player 1 : Rock 00357 //Player 2 : Scissors 00358 else if(choice1 == 'R' && choice2 == 'S'){ 00359 00360 lcd.cls(); 00361 lcd.locate(1,1); 00362 lcd.printf("P1 chose Rock\n"); 00363 lcd.printf(" P2 chose Scissors\n\n"); 00364 lcd.locate(1,3); 00365 lcd.printf("P1 wins!"); 00366 p1++; 00367 lcd.circle(60, 70, 30,WHITE); 00368 wait(5); 00369 } 00370 //Case 7: 00371 //Player 1 : Scissors 00372 //Player 2 : Rock 00373 00374 else if(choice1 == 'S' && choice2 == 'R'){ 00375 00376 lcd.cls(); 00377 lcd.locate(1,1); 00378 lcd.printf("P1 chose Scissors "); 00379 lcd.printf(" P2 chose Rock\n\n"); 00380 lcd.locate(1,3); 00381 lcd.printf("P2 wins!"); 00382 p2++; 00383 lcd.circle(60, 70, 30,WHITE); 00384 wait(5); 00385 } 00386 00387 lcd.cls(); 00388 lcd.locate(1,4); 00389 lcd.rectangle(0,0,125,125,WHITE); //Draw white border 00390 lcd.printf(" P1 Score : %d\n",p1); 00391 lcd.printf(" P2 Score : %d\n\n\n\n",p2); 00392 lcd.printf(" 1 to continue\n\n"); 00393 lcd.printf(" 2 to end\n\n"); 00394 00395 char continue1 = 'N'; 00396 char continue2 = 'N'; 00397 //char cont; 00398 00399 00400 while(1){ 00401 if(pb1 != 1) { 00402 lcd.cls(); 00403 continue2 = 'Y'; 00404 break; 00405 //goto L3; 00406 } 00407 else if(pb2 != 1) { 00408 lcd.cls(); 00409 continue2 = 'N'; 00410 break; 00411 } 00412 } 00413 00414 00415 player2.printf("%c",continue2); 00416 myled3 = 1; 00417 wait(1); 00418 00419 while(1){ 00420 lcd.locate(1,4); 00421 lcd.printf(" Waiting for \n the other player"); 00422 if(player2.readable()){ 00423 continue1 = player2.getc(); 00424 myled = 1; 00425 break; 00426 } 00427 } 00428 00429 //If both say continue then you need to go back to L3 00430 if(continue1 == 'Y' && continue2 == 'Y') goto L3; 00431 else goto L2; 00432 } } 00433 00434 00435 //If game ends : 00436 L2: 00437 lcd.cls(); 00438 //Create animation of two bouncing balls 00439 float fx=50.0,fy=21.0,vx=5.0,vy=0.4,vx2=2,fx2=40.0; 00440 int x=50,y=21,radius=4; 00441 int x2 = 30, y2 = 10; 00442 //lcd.rectangle(0,0,125,125,GREEN); //Draw green border 00443 //draw walls 00444 wait(2); 00445 //If Player 1's score is higher than Player 2's score 00446 if(p1 > p2) 00447 lcd.text_string("Player 1 Wins!", 2, 4, FONT_7X8, WHITE); 00448 //If Player 1's score is equal to Player 2's score 00449 else if(p1 == p2) 00450 lcd.text_string("It's a draw!", 2, 4, FONT_7X8, WHITE); 00451 //If Player 2's score is higher than Player 1's score 00452 else if(p2 > p1) 00453 lcd.text_string("Player 2 Wins!", 2, 4, FONT_7X8, WHITE); 00454 00455 // Play winning tune 00456 FILE *wave_file; 00457 printf("\n\n\nHello, wave world!\n"); 00458 wave_file=fopen("/sd/mydir/win.wav","r"); 00459 waver.play(wave_file); 00460 00461 printf("ok!!"); 00462 fclose(wave_file); 00463 00464 for (int i=0; i<100; i++) { 00465 //draw ball 00466 //lcd.cls(); 00467 if(p1 > p2) 00468 //lcd.printf("Player 1 wins!"); 00469 lcd.text_string("Player 1 Wins!", 2, 4, FONT_7X8, WHITE); 00470 00471 else if(p1 == p2) 00472 //lcd.printf("It's a draw!"); 00473 lcd.text_string("It's a draw!", 2, 4, FONT_7X8, WHITE); 00474 00475 else if(p2 > p1) 00476 //lcd.printf("Player 2 wins!"); 00477 lcd.text_string("Player 2 Wins!", 2, 4, FONT_7X8, WHITE); 00478 00479 lcd.filled_circle(x, y, radius, RED); 00480 lcd.filled_circle(x2,y2,radius,BLUE); 00481 RGB_LED(x,y,x); 00482 //bounce off edge walls and slow down a bit 00483 if ((x<=radius+1) || (x>=126-radius)) vx = -.90*vx; 00484 if ((y<=radius+1) || (y>=126-radius)) vy = -.90*vy; 00485 if ((x2<=radius+1) || (x2>=126-radius)) vx2 = -.90*vx2; 00486 if ((y2<=radius+1) || (y2>=126-radius)) vy = -.90*vy; 00487 //erase old ball location 00488 lcd.filled_circle(x, y, radius, BLACK); 00489 lcd.filled_circle(x2, y2, radius, BLACK); 00490 //move ball 00491 fx=fx+vx; 00492 fx2=fx2+vx2; 00493 fy=fy+vy; 00494 x=(int)fx; 00495 y=(int)fy; 00496 x2=(int)fx2; 00497 y2=(int)fy; 00498 } 00499 lcd.cls(); 00500 00501 }
Generated on Sat Jul 16 2022 19:34:15 by
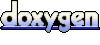