
The final project of Embedde class.
Dependencies: C12832 LM75B ESP-call MMA7660
Talkie.cpp
00001 // Talkie library 00002 // Copyright 2011 Peter Knight 00003 // This code is released under GPLv2 license. 00004 00005 #include "Talkie.h" 00006 00007 Timeout timeout; 00008 PwmOut speaker(D6); 00009 00010 #define FS 8000 // Speech engine sample rate 00011 00012 uint8_t synthPeriod; 00013 uint16_t synthEnergy; 00014 int16_t synthK1,synthK2; 00015 int8_t synthK3,synthK4,synthK5,synthK6,synthK7,synthK8,synthK9,synthK10; 00016 00017 uint8_t tmsEnergy[0x10] = {0x00,0x02,0x03,0x04,0x05,0x07,0x0a,0x0f,0x14,0x20,0x29,0x39,0x51,0x72,0xa1,0xff}; 00018 uint8_t tmsPeriod[0x40] = {0x00,0x10,0x11,0x12,0x13,0x14,0x15,0x16,0x17,0x18,0x19,0x1A,0x1B,0x1C,0x1D,0x1E,0x1F,0x20,0x21,0x22,0x23,0x24,0x25,0x26,0x27,0x28,0x29,0x2A,0x2B,0x2D,0x2F,0x31,0x33,0x35,0x36,0x39,0x3B,0x3D,0x3F,0x42,0x45,0x47,0x49,0x4D,0x4F,0x51,0x55,0x57,0x5C,0x5F,0x63,0x66,0x6A,0x6E,0x73,0x77,0x7B,0x80,0x85,0x8A,0x8F,0x95,0x9A,0xA0}; 00019 int16_t tmsK1[0x20] = {-32064, -31872, -31808, -31680, -31552, -31424, -31232, -30848, -30592, -30336, -30016, -29696, -29376, -28928, -28480, -27968, -26368, -24256, -21632, -18368, -14528, -10048, -5184, 0, 5184, 10048, 14528, 18368, 21632, 24256, 26368, 27968}; 00020 int16_t tmsK2[0x20] = {-20992, -19328, -17536, -15552, -13440, -11200, -8768, -6272, -3712, -1088, 1536, 4160, 6720, 9216, 11584, 13824, 15936, 17856, 19648, 21248, 22656, 24000, 25152, 26176, 27072, 27840, 28544, 29120, 29632, 30080, 30464, 32384}; 00021 int8_t tmsK3[0x10] = {-110, -97, -83, -70, -56, -43, -29, -16, -2, 11, 25, 38, 52, 65, 79, 92}; 00022 int8_t tmsK4[0x10] = {-82, -68, -54, -40, -26, -12, 1, 15}; 00023 int8_t tmsK5[0x10] = {-82, -70, -59, -47, -35, -24, -12, -1, 11, 23, 34, 46, 57, 69, 81, 92}; 00024 int8_t tmsK6[0x10] = {-64, -53, -42, -31, -20, -9, 3, 14, 25, 36, 47, 58, 69, 80, 91, 102}; 00025 int8_t tmsK7[0x10] = {-77, -65, -53, -41, -29, -17, -5, 7, 19, 31, 43, 55, 67, 79, 90, 102}; 00026 int8_t tmsK8[0x08] = {-64, -40, -16, 7, 31, 55, 79, 102}; 00027 int8_t tmsK9[0x08] = {-64, -44, -24, -4, 16, 37, 57, 77}; 00028 int8_t tmsK10[0x08] = {-51, -33, -15, 4, 22, 32, 59, 77}; 00029 00030 void TIMEOUT(void); 00031 00032 void Talkie::setPtr(uint8_t* addr) 00033 { 00034 ptrAddr = addr; 00035 ptrBit = 0; 00036 } 00037 00038 // The ROMs used with the TI speech were serial, not byte wide. 00039 // Here's a handy routine to flip ROM data which is usually reversed. 00040 uint8_t Talkie::rev(uint8_t a) 00041 { 00042 // 76543210 00043 a = (a>>4) | (a<<4); // Swap in groups of 4 00044 // 32107654 00045 a = ((a & 0xcc)>>2) | ((a & 0x33)<<2); // Swap in groups of 2 00046 // 10325476 00047 a = ((a & 0xaa)>>1) | ((a & 0x55)<<1); // Swap bit pairs 00048 // 01234567 00049 return a; 00050 } 00051 uint8_t Talkie::getBits(uint8_t bits) 00052 { 00053 uint8_t value; 00054 uint16_t data; 00055 data = rev((*ptrAddr))<<8; 00056 if (ptrBit+bits > 8) { 00057 data |= rev((*(ptrAddr+1))); 00058 } 00059 data <<= ptrBit; 00060 value = data >> (16-bits); 00061 ptrBit += bits; 00062 if (ptrBit >= 8) { 00063 ptrBit -= 8; 00064 ptrAddr++; 00065 } 00066 return value; 00067 } 00068 void Talkie::say(uint8_t* addr) 00069 { 00070 uint8_t energy; 00071 00072 if (!setup) { 00073 // Auto-setup. 00074 // 00075 // Enable the speech system whenever say() is called. 00076 00077 // Timer 2 set up as a 62500Hz PWM. 00078 // 00079 // The PWM 'buzz' is well above human hearing range and is 00080 // very easy to filter out. 00081 // 00082 speaker.period_us(16); 00083 00084 // Unfortunately we can't calculate the next sample every PWM cycle 00085 // as the routine is too slow. So use Timer 1 to trigger that. 00086 00087 // Timer 1 set up as a 8000Hz sample interrupt 00088 timeout.attach_us(TIMEOUT, 125); 00089 00090 setup = 1; 00091 } 00092 00093 setPtr(addr); 00094 do { 00095 uint8_t repeat; 00096 00097 // Read speech data, processing the variable size frames. 00098 00099 energy = getBits(4); 00100 if (energy == 0) { 00101 // Energy = 0: rest frame 00102 synthEnergy = 0; 00103 } else if (energy == 0xf) { 00104 // Energy = 15: stop frame. Silence the synthesiser. 00105 synthEnergy = 0; 00106 synthK1 = 0; 00107 synthK2 = 0; 00108 synthK3 = 0; 00109 synthK4 = 0; 00110 synthK5 = 0; 00111 synthK6 = 0; 00112 synthK7 = 0; 00113 synthK8 = 0; 00114 synthK9 = 0; 00115 synthK10 = 0; 00116 } else { 00117 synthEnergy = tmsEnergy[energy]; 00118 repeat = getBits(1); 00119 synthPeriod = tmsPeriod[getBits(6)]; 00120 // A repeat frame uses the last coefficients 00121 if (!repeat) { 00122 // All frames use the first 4 coefficients 00123 synthK1 = tmsK1[getBits(5)]; 00124 synthK2 = tmsK2[getBits(5)]; 00125 synthK3 = tmsK3[getBits(4)]; 00126 synthK4 = tmsK4[getBits(4)]; 00127 if (synthPeriod) { 00128 // Voiced frames use 6 extra coefficients. 00129 synthK5 = tmsK5[getBits(4)]; 00130 synthK6 = tmsK6[getBits(4)]; 00131 synthK7 = tmsK7[getBits(4)]; 00132 synthK8 = tmsK8[getBits(3)]; 00133 synthK9 = tmsK9[getBits(3)]; 00134 synthK10 = tmsK10[getBits(3)]; 00135 } 00136 } 00137 } 00138 thread_sleep_for(25); 00139 } while (energy != 0xf); 00140 } 00141 00142 #define CHIRP_SIZE 41 00143 int8_t chirp[CHIRP_SIZE] = {0, 42, -44, 50, -78, 18, 37, 20, 2, -31, -59, 2, 95, 90, 5, 15, 38, -4, -91, -91, -42, -35, -36, -4, 37, 43, 34, 33, 15, -1, -8, -18, -19, -17, -9, -10, -6, 0, 3, 2, 1}; 00144 void TIMEOUT(void) 00145 { 00146 static uint8_t nextPwm; 00147 static uint8_t periodCounter; 00148 static int16_t x0,x1,x2,x3,x4,x5,x6,x7,x8,x9,x10; 00149 int16_t u0,u1,u2,u3,u4,u5,u6,u7,u8,u9,u10; 00150 00151 speaker = float(nextPwm) * 0.392; 00152 // sei(); 00153 if (synthPeriod) { 00154 // Voiced source 00155 if (periodCounter < synthPeriod) { 00156 periodCounter++; 00157 } else { 00158 periodCounter = 0; 00159 } 00160 if (periodCounter < CHIRP_SIZE) { 00161 u10 = ((chirp[periodCounter]) * (uint32_t) synthEnergy) >> 8; 00162 } else { 00163 u10 = 0; 00164 } 00165 } else { 00166 // Unvoiced source 00167 static uint16_t synthRand = 1; 00168 synthRand = (synthRand >> 1) ^ ((synthRand & 1) ? 0xB800 : 0); 00169 u10 = (synthRand & 1) ? synthEnergy : -synthEnergy; 00170 } 00171 // Lattice filter forward path 00172 u9 = u10 - (((int16_t)synthK10*x9) >> 7); 00173 u8 = u9 - (((int16_t)synthK9*x8) >> 7); 00174 u7 = u8 - (((int16_t)synthK8*x7) >> 7); 00175 u6 = u7 - (((int16_t)synthK7*x6) >> 7); 00176 u5 = u6 - (((int16_t)synthK6*x5) >> 7); 00177 u4 = u5 - (((int16_t)synthK5*x4) >> 7); 00178 u3 = u4 - (((int16_t)synthK4*x3) >> 7); 00179 u2 = u3 - (((int16_t)synthK3*x2) >> 7); 00180 u1 = u2 - (((int32_t)synthK2*x1) >> 15); 00181 u0 = u1 - (((int32_t)synthK1*x0) >> 15); 00182 00183 // Output clamp 00184 if (u0 > 511) u0 = 511; 00185 if (u0 < -512) u0 = -512; 00186 00187 // Lattice filter reverse path 00188 x9 = x8 + (((int16_t)synthK9*u8) >> 7); 00189 x8 = x7 + (((int16_t)synthK8*u7) >> 7); 00190 x7 = x6 + (((int16_t)synthK7*u6) >> 7); 00191 x6 = x5 + (((int16_t)synthK6*u5) >> 7); 00192 x5 = x4 + (((int16_t)synthK5*u4) >> 7); 00193 x4 = x3 + (((int16_t)synthK4*u3) >> 7); 00194 x3 = x2 + (((int16_t)synthK3*u2) >> 7); 00195 x2 = x1 + (((int32_t)synthK2*u1) >> 15); 00196 x1 = x0 + (((int32_t)synthK1*u0) >> 15); 00197 x0 = u0; 00198 00199 nextPwm = (u0>>2)+0x80; 00200 }
Generated on Fri Jul 15 2022 05:56:23 by
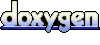