
The final project of Embedde class.
Dependencies: C12832 LM75B ESP-call MMA7660
SWITCH.cpp
00001 #include "SWITCH.h" 00002 00003 InterruptIn switch2(SW2); 00004 InterruptIn switch3(SW3); 00005 00006 Semaphore semaphoreISRSwitchLeft(0, 1); 00007 Semaphore semaphoreISRSwitchRight(0, 1); 00008 00009 static bool _switchData[2] = {0, 0}; 00010 00011 static void _switchBeep() 00012 { 00013 buzzerInstruction = BUZZER_INSTRUCTION_RINGING; 00014 buzzerDelay = SWITCH_DEBOUNCING_DELAY; 00015 buzzerCnt = SWITCH_BEEP_CNT; 00016 semaphoreBuzzerValues.release(); 00017 semaphoreBuzzer.release(); 00018 } 00019 static void _switchLeftPushed(void) 00020 { 00021 _switchData[SWITCH_LEFT] = 1; 00022 if(semaphoreBuzzerValues.try_acquire() == true) { 00023 _switchBeep(); 00024 } 00025 semaphoreISRSwitchLeft.release(); 00026 } 00027 static void _switchRightPushed(void) 00028 { 00029 _switchData[SWITCH_RIGHT] = 1; 00030 if(semaphoreBuzzerValues.try_acquire() == true) { 00031 _switchBeep(); 00032 } 00033 semaphoreISRSwitchRight.release(); 00034 } 00035 00036 static void _switchLeftReleased(void) 00037 { 00038 _switchData[SWITCH_LEFT] = 0; 00039 semaphoreISRSwitchLeft.release(); 00040 } 00041 00042 static void _switchRightReleased(void) 00043 { 00044 _switchData[SWITCH_RIGHT] = 0; 00045 semaphoreISRSwitchRight.release(); 00046 } 00047 00048 // ==================================================================================== 00049 00050 void switchInit(void) 00051 { 00052 printf("Init switches\r\n"); 00053 switch3.rise(_switchLeftReleased); 00054 switch2.rise(_switchRightReleased); 00055 switch3.fall(_switchLeftPushed); 00056 switch2.fall(_switchRightPushed); 00057 } 00058 00059 bool switchValue(int dir) 00060 { 00061 return _switchData[dir]; 00062 } 00063 00064 bool switchValueWait(int dir) 00065 { 00066 if(dir == SWITCH_LEFT) { 00067 semaphoreISRSwitchLeft.acquire(); 00068 } else { 00069 semaphoreISRSwitchRight.acquire(); 00070 } 00071 printf("Switch%d read\r\n",dir); 00072 return _switchData[dir]; 00073 }
Generated on Fri Jul 15 2022 05:56:23 by
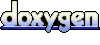