
The final project of Embedde class.
Dependencies: C12832 LM75B ESP-call MMA7660
JOYSTICK.cpp
00001 #include "JOYSTICK.h" 00002 00003 InterruptIn joystickU(A2); 00004 InterruptIn joystickD(A3); 00005 InterruptIn joystickL(A4); 00006 InterruptIn joystickR(A5); 00007 InterruptIn joystickC(D4); 00008 00009 static int _joystickDataForISR = -1; 00010 00011 static void _joystickBeep() 00012 { 00013 buzzerInstruction = BUZZER_INSTRUCTION_RINGING; 00014 buzzerDelay = JOYSTICK_BEEP_DELAY; 00015 buzzerCnt = JOYSTICK_BEEP_CNT; 00016 semaphoreBuzzerValues.release(); 00017 semaphoreBuzzer.release(); 00018 } 00019 00020 static void _joystickPushedU() 00021 { 00022 if(semaphoreBuzzerValues.try_acquire() == true) { 00023 _joystickDataForISR = JOYSTICK_U; 00024 _joystickBeep(); 00025 } 00026 } 00027 static void _joystickPushedD() 00028 { 00029 if(semaphoreBuzzerValues.try_acquire() == true) { 00030 _joystickDataForISR = JOYSTICK_D; 00031 _joystickBeep(); 00032 } 00033 } 00034 static void _joystickPushedL() 00035 { 00036 if(semaphoreBuzzerValues.try_acquire() == true) { 00037 _joystickDataForISR = JOYSTICK_L; 00038 _joystickBeep(); 00039 } 00040 00041 } 00042 static void _joystickPushedR() 00043 { 00044 if(semaphoreBuzzerValues.try_acquire() == true) { 00045 _joystickDataForISR = JOYSTICK_R; 00046 _joystickBeep(); 00047 00048 } 00049 } 00050 static void _joystickPushedC() 00051 { 00052 if(semaphoreBuzzerValues.try_acquire() == true) { 00053 _joystickDataForISR = JOYSTICK_C; 00054 _joystickBeep(); 00055 00056 } 00057 } 00058 static void _joystickReleased(void) 00059 { 00060 _joystickDataForISR = -1; 00061 } 00062 00063 00064 // ==================================================================================== 00065 00066 void joystickInit(void) 00067 { 00068 printf("Init joysticks\r\n"); 00069 joystickU.rise(_joystickPushedU); 00070 joystickD.rise(_joystickPushedD); 00071 joystickL.rise(_joystickPushedL); 00072 joystickR.rise(_joystickPushedR); 00073 joystickC.rise(_joystickPushedC); 00074 00075 joystickU.fall(_joystickReleased); 00076 joystickD.fall(_joystickReleased); 00077 joystickL.fall(_joystickReleased); 00078 joystickR.fall(_joystickReleased); 00079 joystickC.fall(_joystickReleased); 00080 } 00081 00082 00083 int joystickDir(void) 00084 { 00085 return _joystickDataForISR; 00086 }
Generated on Fri Jul 15 2022 05:56:23 by
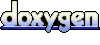