
The final project of Embedde class.
Dependencies: C12832 LM75B ESP-call MMA7660
BUZZER.cpp
00001 #include "BUZZER.h" 00002 00003 PwmOut buzzer(BUZZER_PIN); 00004 Thread buzzerThread; 00005 00006 Semaphore semaphoreBuzzer(0); 00007 Semaphore semaphoreBuzzerValues(1); 00008 00009 char buzzerInstruction = 0; 00010 short buzzerCnt = 0; 00011 int buzzerDelay = 0; 00012 int buzzerFreq = BUZZER_BEEP_FREQUENCY; 00013 00014 static void _buzzerThread(void) 00015 { 00016 int instruction; 00017 int delay; 00018 int cnt; 00019 int freq; 00020 while(1) { 00021 semaphoreBuzzer.acquire(); 00022 semaphoreBuzzerValues.acquire(); 00023 00024 instruction = buzzerInstruction; 00025 delay = buzzerDelay; 00026 cnt = buzzerCnt; 00027 buzzerInstruction = BUZZER_INSTRUCTION_OFF; 00028 freq = buzzerFreq; 00029 00030 semaphoreBuzzerValues.release(); 00031 00032 switch(instruction) { 00033 case BUZZER_INSTRUCTION_INIT: 00034 buzzer.period(1.0 / BUZZER_BEEP_FREQUENCY); 00035 case BUZZER_INSTRUCTION_OFF: 00036 buzzer = 0; 00037 break; 00038 00039 case BUZZER_INSTRUCTION_ON: 00040 buzzer.period(1.0 / freq); 00041 buzzer = 0.5; 00042 break; 00043 00044 case BUZZER_INSTRUCTION_RINGING: 00045 buzzer.period(1.0 / freq); 00046 for(cnt; cnt > 0; cnt--) { 00047 buzzer = 0.5; 00048 thread_sleep_for(delay); 00049 buzzer = 0.0; 00050 thread_sleep_for(delay); 00051 } 00052 break; 00053 } 00054 } 00055 } 00056 00057 void buzzerInit(void) 00058 { 00059 printf("Init buzzer\r\n"); 00060 semaphoreBuzzerValues.acquire(); 00061 buzzerInstruction = BUZZER_INSTRUCTION_INIT; 00062 buzzerCnt = 0; 00063 buzzerDelay = 0; 00064 semaphoreBuzzerValues.release(); 00065 semaphoreBuzzer.release(); 00066 buzzerThread.start(_buzzerThread); 00067 } 00068 00069 void buzzerOn(int freq) 00070 { 00071 semaphoreBuzzerValues.acquire(); 00072 buzzerFreq = freq; 00073 buzzerInstruction = BUZZER_INSTRUCTION_ON; 00074 semaphoreBuzzerValues.release(); 00075 semaphoreBuzzer.release(); 00076 } 00077 00078 void buzzerOn(void) 00079 { 00080 semaphoreBuzzerValues.acquire(); 00081 buzzerFreq = BUZZER_BEEP_FREQUENCY; 00082 buzzerInstruction = BUZZER_INSTRUCTION_ON; 00083 semaphoreBuzzerValues.release(); 00084 semaphoreBuzzer.release(); 00085 } 00086 00087 void buzzerOff(void) 00088 { 00089 semaphoreBuzzerValues.acquire(); 00090 buzzerInstruction = BUZZER_INSTRUCTION_OFF; 00091 semaphoreBuzzerValues.release(); 00092 semaphoreBuzzer.release(); 00093 } 00094 void buzzerRinging(short cnt, int delay, int freq) 00095 { 00096 semaphoreBuzzerValues.acquire(); 00097 buzzerFreq = freq; 00098 buzzerInstruction = BUZZER_INSTRUCTION_RINGING; 00099 buzzerCnt = cnt; 00100 buzzerDelay = delay; 00101 semaphoreBuzzerValues.release(); 00102 semaphoreBuzzer.release(); 00103 } 00104 00105 void buzzerRinging(short cnt, int delay) 00106 { 00107 semaphoreBuzzerValues.acquire(); 00108 buzzerFreq = BUZZER_BEEP_FREQUENCY; 00109 buzzerInstruction = BUZZER_INSTRUCTION_RINGING; 00110 buzzerCnt = cnt; 00111 buzzerDelay = delay; 00112 semaphoreBuzzerValues.release(); 00113 semaphoreBuzzer.release(); 00114 }
Generated on Fri Jul 15 2022 05:56:23 by
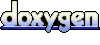