XBee API operation library for mbed for miniprojects
Dependencies: SmartLabXBeeCore
Fork of SmartLabXBeeAPI2 by
SerialData.h
00001 #ifndef UK_AC_HERTS_SMARTLAB_XBEE_SerialData 00002 #define UK_AC_HERTS_SMARTLAB_XBEE_SerialData 00003 00004 #include "mbed.h" 00005 #include "ISerial.h" 00006 00007 /// The detailed implementation of ISerial, which is responsible for sending and receiving seial data. 00008 class SerialData: public ISerial 00009 { 00010 private: 00011 /// mbed serial port interface. 00012 Serial * serialPort; 00013 00014 public: 00015 /** Create a SerialData instance which using pin tx and rx. 00016 * 00017 * @param tx data transmission line 00018 * @param rx data receiving line 00019 * 00020 */ 00021 SerialData(PinName tx, PinName rx); 00022 /** Create a SerialData instance which using pin tx and rx. 00023 * 00024 * @param tx data transmission line 00025 * @param rx data receiving line 00026 * @param baudRate baud rate 00027 * 00028 */ 00029 SerialData(PinName tx, PinName rx, int baudRate); 00030 00031 ~SerialData(); 00032 00033 /** 00034 * Read the next avaliable data. 00035 * 00036 * @returns [0x00-0xFF], -1 means data not avaliable. 00037 * 00038 */ 00039 virtual int readByte(); 00040 00041 /** 00042 * Write one byte of data. 00043 * 00044 * @param data [0x00-0xFF] 00045 * 00046 */ 00047 virtual void writeByte(char data); 00048 00049 /** Check if the serial port is open, not implemented and has no affect, 00050 * @returns always true 00051 */ 00052 virtual bool isOpen(); 00053 00054 /// Open the serial port, not implemented and has no affect. 00055 virtual void open(); 00056 00057 /// Close the serial port, not implemented and has no affect. 00058 virtual void close(); 00059 00060 /** Check if data is ready to read. 00061 * @returns true data avaliable. 00062 * false data not avaliable. 00063 */ 00064 virtual bool peek(); 00065 }; 00066 00067 #endif
Generated on Sun Jul 17 2022 19:50:18 by
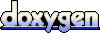