
Hack the PHS Shield, serial bridge
Fork of PHSShield_F405hack by
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * select: Nucleo F401RE 00003 */ 00004 #include "mbed.h" 00005 #include "phs_f405.h" 00006 00007 //#define BAUD 120000 00008 #define BAUD 9600 00009 00010 Serial pc(PB_6, PB_7); 00011 00012 ShieldSerial ser(BAUD); // PC_12, PD_2 00013 DigitalIn pwron(PC_5), regon(PC_4); 00014 DigitalOut state(PB_1); 00015 00016 Serial phs(PA_2, PA_3); 00017 DigitalOut rts(PA_1), dsr(PA_5); 00018 DigitalIn cts(PA_0), dcd(PA_7), dtr(PA_6), ri(PA_4); 00019 PhsReset reset; // PB_11 00020 00021 DigitalOut power(PC_9); 00022 DigitalOut led1(PC_7), led2(PC_6); 00023 00024 int main() { 00025 pwron.mode(PullUp); 00026 regon.mode(PullDown); 00027 reset = 0; 00028 power = 0; // DCDC on 00029 led1 = 0; 00030 led2 = 0; 00031 phs.baud(BAUD); 00032 cts.mode(PullUp); 00033 dsr = rts = 1; 00034 wait_ms(200); 00035 00036 for (;;) { 00037 if (phs.readable() && ser.writeable()) { 00038 ser.putc(phs.getc()); 00039 } 00040 if (ser.readable() && phs.writeable()) { 00041 phs.putc(ser.getc()); 00042 } 00043 00044 reset = pwron; 00045 led2 = pwron; 00046 dsr = rts = regon; 00047 } 00048 }
Generated on Wed Jul 13 2022 02:56:56 by
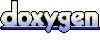