PHS module APM-002 library. see: https://developer.mbed.org/users/phsfan/notebook/abitusbmodem/
Dependencies: Socket lwip-sys lwip
Fork of AbitUSBModem by
AbitModemInterface.cpp
00001 /* AbitUSBModem.cpp */ 00002 /* Modified by 2015 phsfan 00003 * for ABIT SMA-01 00004 */ 00005 /* VodafoneUSBModem.cpp */ 00006 /* Copyright (C) 2012 mbed.org, MIT License 00007 * 00008 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00009 * and associated documentation files (the "Software"), to deal in the Software without restriction, 00010 * including without limitation the rights to use, copy, modify, merge, publish, distribute, 00011 * sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is 00012 * furnished to do so, subject to the following conditions: 00013 * 00014 * The above copyright notice and this permission notice shall be included in all copies or 00015 * substantial portions of the Software. 00016 * 00017 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00018 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00019 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00020 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00022 */ 00023 00024 00025 #define __DEBUG__ 0 00026 00027 #ifndef __MODULE__ 00028 #define __MODULE__ "AbitUSBModem.cpp" 00029 #endif 00030 00031 #include "core/fwk.h" 00032 00033 #include "AbitModemInterface.h" 00034 #include "Socket.h" 00035 00036 AbitModemInterface::AbitModemInterface (PinName tx, PinName rx, PinName cts, PinName rts, PinName reset, int baud) : 00037 m_module(tx, rx), // Construct AbitModemInterface: Serial interface 00038 #if !DEVICE_SERIAL_FC 00039 m_cts(cts), 00040 m_rts(rts), 00041 #endif 00042 m_reset(reset), 00043 m_pppStream(m_module), // PPP connections are managed via another serial channel. 00044 m_at(&m_pppStream), // Construct ATCommandsInterface with the AT serial channel 00045 m_sms(&m_at), // Construct SMSInterface with the ATCommandsInterface 00046 m_ppp(&m_pppStream, &m_pppStream, &m_at, false), // Construct PPPIPInterface with the PPP serial channel 00047 m_moduleConnected(false), // Dongle is initially not ready for anything 00048 m_ipInit(false), // PPIPInterface connection is initially down 00049 m_smsInit(false), // SMSInterface starts un-initialised 00050 m_atOpen(false) // ATCommandsInterface starts in a closed state 00051 { 00052 m_reset.output(); 00053 m_reset = 0; 00054 m_module.baud(baud); 00055 #if DEVICE_SERIAL_FC 00056 if (rts != NC && cts != NC) { 00057 m_module.set_flow_control(Serial::RTSCTS, rts, cts); 00058 } else 00059 if (rts != NC && cts == NC) { 00060 m_module.set_flow_control(Serial::RTS, rts); 00061 } else 00062 if (rts == NC && cts != NC) { 00063 m_module.set_flow_control(Serial::CTS, cts); 00064 } 00065 #else 00066 m_rts = 0; 00067 m_cts.mode(PullUp); 00068 #endif 00069 Thread::wait(100); 00070 m_reset.input(); 00071 m_reset.mode(PullUp); 00072 } 00073 00074 int AbitModemInterface::connect (const char* user, const char* password) { 00075 00076 if( !m_ipInit ) 00077 { 00078 m_ipInit = true; 00079 m_ppp.init(); 00080 } 00081 m_ppp.setup(user, password); 00082 00083 int ret = init(); 00084 if(ret) 00085 { 00086 return ret; 00087 } 00088 00089 m_at.close(); // Closing AT parser 00090 m_atOpen = false; //Will need to be reinitialized afterwards 00091 00092 DBG("Connecting PPP"); 00093 00094 ret = m_ppp.connect(); 00095 DBG("Result of connect: Err code=%d", ret); 00096 return ret; 00097 } 00098 00099 int AbitModemInterface::disconnect () { 00100 DBG("Disconnecting from PPP"); 00101 int ret = m_ppp.disconnect(); 00102 if(ret) 00103 { 00104 ERR("Disconnect returned %d, still trying to disconnect", ret); 00105 } 00106 00107 return OK; 00108 } 00109 00110 00111 00112 int AbitModemInterface::init() 00113 { 00114 //DBG("Entering init method for the VodafoneUSBModem"); 00115 if( !m_moduleConnected ) 00116 { 00117 DBG("Dongle is not connected"); 00118 00119 m_moduleConnected = true; 00120 Thread::wait(3000); 00121 00122 } 00123 00124 if(m_atOpen) 00125 { 00126 return OK; 00127 } 00128 00129 DBG("Starting AT thread if needed"); 00130 int ret = m_at.open(); 00131 if(ret) 00132 { 00133 return ret; 00134 } 00135 00136 DBG("Sending initialisation commands"); 00137 ret = m_at.init(); 00138 if(ret) 00139 { 00140 return ret; 00141 } 00142 00143 ret = m_at.executeSimple("ATE1", NULL); 00144 DBG("Result of command: Err code=%d", ret); 00145 if(ret != OK) 00146 { 00147 return NET_PROTOCOL; 00148 } 00149 00150 m_at.executeSimple("AT#S1", NULL); 00151 m_at.executeSimple("AT#B1", NULL); 00152 Thread::wait(5000); 00153 00154 m_atOpen = true; 00155 00156 return OK; 00157 } 00158 00159 00160 int AbitModemInterface::sendSM(const char* number, const char* message) 00161 { 00162 int ret = init(); 00163 if(ret) 00164 { 00165 return ret; 00166 } 00167 00168 if(!m_smsInit) 00169 { 00170 ret = m_sms.init(); 00171 if(ret) 00172 { 00173 return ret; 00174 } 00175 m_smsInit = true; 00176 } 00177 00178 ret = m_sms.send(number, message); 00179 if(ret) 00180 { 00181 return ret; 00182 } 00183 00184 return OK; 00185 } 00186 00187 int AbitModemInterface::getSM(char* number, char* message, size_t maxLength) 00188 { 00189 int ret = init(); 00190 if(ret) 00191 { 00192 return ret; 00193 } 00194 00195 if(!m_smsInit) 00196 { 00197 ret = m_sms.init(); 00198 if(ret) 00199 { 00200 return ret; 00201 } 00202 m_smsInit = true; 00203 } 00204 00205 ret = m_sms.get(number, message, maxLength); 00206 if(ret) 00207 { 00208 return ret; 00209 } 00210 00211 return OK; 00212 } 00213 00214 char* AbitModemInterface::getIPAddress() 00215 { 00216 return m_ppp.getIPAddress(); 00217 }
Generated on Wed Jul 13 2022 04:34:31 by
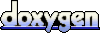