C++ library, driver software code for the low-power small WLP package MAX31875 temperature sensor. Code supports one-shot, shut-down/standby, hysteresis, alarm limits.
Dependents: MAX31875_Temperature_Sensor_Small_Package
max31875.cpp
00001 /******************************************************************************* 00002 * Copyright (C) 2019 Maxim Integrated Products, Inc., All Rights Reserved. 00003 * 00004 * Permission is hereby granted, free of charge, to any person obtaining a 00005 * copy of this software and associated documentation files (the "Software"), 00006 * to deal in the Software without restriction, including without limitation 00007 * the rights to use, copy, modify, merge, publish, distribute, sublicense, 00008 * and/or sell copies of the Software, and to permit persons to whom the 00009 * Software is furnished to do so, subject to the following conditions: 00010 * 00011 * The above copyright notice and this permission notice shall be included 00012 * in all copies or substantial portions of the Software. 00013 * 00014 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS 00015 * OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF 00016 * MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. 00017 * IN NO EVENT SHALL MAXIM INTEGRATED BE LIABLE FOR ANY CLAIM, DAMAGES 00018 * OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, 00019 * ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR 00020 * OTHER DEALINGS IN THE SOFTWARE. 00021 * 00022 * Except as contained in this notice, the name of Maxim Integrated 00023 * Products, Inc. shall not be used except as stated in the Maxim Integrated 00024 * Products, Inc. Branding Policy. 00025 * 00026 * The mere transfer of this software does not imply any licenses 00027 * of trade secrets, proprietary technology, copyrights, patents, 00028 * trademarks, maskwork rights, or any other form of intellectual 00029 * property whatsoever. Maxim Integrated Products, Inc. retains all 00030 * ownership rights. 00031 ******************************************************************************* 00032 */ 00033 #include "max31875.h" 00034 #include "mbed.h" 00035 // #include "USBSerial.h" 00036 00037 00038 /******************************************************************************/ 00039 MAX31875::MAX31875(I2C &i2c_bus, uint8_t slaveAddress): 00040 m_i2c(i2c_bus), 00041 m_writeAddress(slaveAddress <<1), 00042 m_readAddress ((slaveAddress << 1) | 1), 00043 m_extended_format(0) 00044 { 00045 } 00046 00047 00048 /******************************************************************************/ 00049 MAX31875::~MAX31875(void) 00050 { 00051 /** empty block */ 00052 } 00053 00054 00055 /******************************************************************************/ 00056 int MAX31875::read_reg(uint16_t *value, char reg) 00057 { 00058 int32_t ret; 00059 char data[2] = {0, 0}; 00060 max31875_raw_data tmp; 00061 00062 if (reg <= MAX31875_REG_MAX) { 00063 /* write to the Register Select */ 00064 ret = m_i2c.write(m_writeAddress, ®, 1, true); 00065 /* read the two bytes of data */ 00066 if (ret == 0) { 00067 ret = m_i2c.read(m_readAddress, data, 2, false); 00068 if (ret == 0) { 00069 tmp.msb = data[0]; /* MSB */ 00070 tmp.lsb = data[1]; /* LSB */ 00071 *value = tmp.swrd; 00072 return MAX31875_NO_ERROR; 00073 } else { 00074 printf( 00075 "%s: failed to read data: ret: %d\r\n", __func__, ret); 00076 } 00077 } else { 00078 printf("%s: failed to write to Register Select: ret: %d\r\n", 00079 __func__, ret); 00080 } 00081 } else { 00082 printf("%s: register address is not correct: register: %d\r\n", 00083 __func__, reg); 00084 } 00085 return MAX31875_ERROR; 00086 } 00087 00088 00089 float MAX31875::read_reg_as_temperature(uint8_t reg) 00090 { 00091 max31875_raw_data tmp; 00092 float temperature; 00093 if (reg == MAX31875_REG_TEMPERATURE || 00094 reg == MAX31875_REG_THYST || reg == MAX31875_REG_TOS) { 00095 read_reg(&tmp.uwrd, reg); 00096 temperature = (float)tmp.swrd; 00097 if (m_extended_format) 00098 temperature *= MAX31875_CF_EXTENDED_FORMAT; 00099 else 00100 temperature *= MAX31875_CF_NORMAL_FORMAT; 00101 return temperature; 00102 } else { 00103 printf("%s: register is invalid, %d r\n", __func__, reg); 00104 return 0; 00105 } 00106 } 00107 00108 00109 /******************************************************************************/ 00110 int MAX31875::write_reg(uint16_t value, char reg) 00111 { 00112 int32_t ret; 00113 char cmd[3]; 00114 max31875_raw_data tmp; 00115 00116 if (reg >= MAX31875_REG_CONFIGURATION && reg <= MAX31875_REG_MAX) { 00117 cmd[0] = reg; 00118 tmp.uwrd = value; 00119 cmd[1] = tmp.msb; 00120 cmd[2] = tmp.lsb; 00121 ret = m_i2c.write(m_writeAddress, cmd, 3, false); 00122 if (ret == 0) { 00123 if (tmp.uwrd & MAX31875_CFG_EXTENDED_FORMAT) 00124 m_extended_format = 1; 00125 else 00126 m_extended_format = 0; 00127 return MAX31875_NO_ERROR; 00128 } else { 00129 printf("%s: I2C write error %d\r\n",__func__, ret); 00130 return MAX31875_ERROR; 00131 } 00132 } else { 00133 printf("%s: register value invalid %x\r\n",__func__, reg); 00134 return MAX31875_ERROR; 00135 } 00136 } 00137 00138 00139 int MAX31875::write_cfg(uint16_t cfg) 00140 { 00141 return write_reg(cfg, MAX31875_REG_CONFIGURATION); 00142 } 00143 00144 00145 int MAX31875::write_trip_low(float temperature) 00146 { 00147 max31875_raw_data raw; 00148 if (m_extended_format) 00149 temperature /= MAX31875_CF_EXTENDED_FORMAT; 00150 else 00151 temperature /= MAX31875_CF_NORMAL_FORMAT; 00152 raw.uwrd = uint16_t(temperature); 00153 return write_reg(raw.uwrd, MAX31875_REG_THYST); 00154 } 00155 00156 00157 int MAX31875::write_trip_high(float temperature) 00158 { 00159 max31875_raw_data raw; 00160 if (m_extended_format) 00161 temperature /= MAX31875_CF_EXTENDED_FORMAT; 00162 else 00163 temperature /= MAX31875_CF_NORMAL_FORMAT; 00164 raw.uwrd = uint16_t(temperature); 00165 return write_reg(raw.uwrd, MAX31875_REG_TOS); 00166 } 00167 00168 00169 float MAX31875::celsius_to_fahrenheit(float temp_c) 00170 { 00171 float temp_f; 00172 temp_f = ((temp_c * 9)/5) + 32; 00173 return temp_f; 00174 }
Generated on Wed Jul 13 2022 19:22:43 by
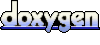