
Six crescent shaped legs
Embed:
(wiki syntax)
Show/hide line numbers
Encoder.hpp
00001 #ifndef ENCODER_H 00002 #define ENCODER_H 00003 00004 #include "mbed.h" 00005 00006 struct EncoderData 00007 { 00008 PinName encAPin, encBPin; 00009 int turnCount; 00010 }; 00011 00012 class Encoder 00013 { 00014 public: 00015 Encoder(EncoderData nData); 00016 00017 virtual void reset(); 00018 00019 long getCount() const; 00020 float getTurn() const; 00021 00022 protected: 00023 EncoderData data; 00024 00025 private: 00026 void changeA(); 00027 void changeB(); 00028 void changeCount(bool flipB); 00029 00030 InterruptIn intA, intB; 00031 volatile bool valA, valB; 00032 00033 volatile long count; 00034 }; 00035 00036 #endif // ENCODER_H
Generated on Thu Jul 14 2022 23:15:59 by
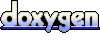