
Six crescent shaped legs
Embed:
(wiki syntax)
Show/hide line numbers
Encoder.cpp
00001 #include "Encoder.hpp" 00002 00003 /* 00004 Forward: 00005 A 0011001100 00006 B 1001100110 00007 pB 1100110011 00008 A != pB 00009 00010 Backward: 00011 A 0011001100 00012 B 0110011001 00013 pB 0011001100 00014 A == pB 00015 */ 00016 00017 Encoder::Encoder(EncoderData nData) : 00018 data(nData), 00019 intA(data.encAPin), intB(data.encBPin), 00020 valA(DigitalIn(data.encAPin).read()), 00021 valB(DigitalIn(data.encBPin).read()) 00022 { 00023 intA.rise(this, &Encoder::changeA); 00024 intA.fall(this, &Encoder::changeA); 00025 intB.rise(this, &Encoder::changeB); 00026 intB.fall(this, &Encoder::changeB); 00027 reset(); 00028 } 00029 00030 void Encoder::reset() 00031 { 00032 count = 0; 00033 } 00034 00035 long Encoder::getCount() const 00036 { 00037 return count; 00038 } 00039 00040 float Encoder::getTurn() const 00041 { 00042 return float(getCount()) / data.turnCount; 00043 } 00044 00045 void Encoder::changeA() 00046 { 00047 valA = !valA; 00048 changeCount(false); 00049 } 00050 00051 void Encoder::changeB() 00052 { 00053 valB = !valB; 00054 changeCount(true); 00055 } 00056 00057 void Encoder::changeCount(bool flipB) 00058 { 00059 if (valA == (valB ^ flipB)) 00060 count++; 00061 else 00062 count--; 00063 }
Generated on Thu Jul 14 2022 23:15:59 by
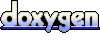