
test
Embed:
(wiki syntax)
Show/hide line numbers
threads_interface.h
Go to the documentation of this file.
00001 /* 00002 * Copyright 2015-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved. 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"). 00005 * You may not use this file except in compliance with the License. 00006 * A copy of the License is located at 00007 * 00008 * http://aws.amazon.com/apache2.0 00009 * 00010 * or in the "license" file accompanying this file. This file is distributed 00011 * on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either 00012 * express or implied. See the License for the specific language governing 00013 * permissions and limitations under the License. 00014 */ 00015 00016 /** 00017 * @file threads_interface.h 00018 * @brief Thread interface definition for MQTT client. 00019 * 00020 * Defines an interface that can be used by system components for multithreaded situations. 00021 * Starting point for porting the SDK to the threading hardware layer of a new platform. 00022 */ 00023 00024 #include "aws_iot_config.h" 00025 00026 #ifdef _ENABLE_THREAD_SUPPORT_ 00027 #ifndef __THREADS_INTERFACE_H_ 00028 #define __THREADS_INTERFACE_H_ 00029 00030 #ifdef __cplusplus 00031 extern "C" { 00032 #endif 00033 00034 /** 00035 * The platform specific timer header that defines the Timer struct 00036 */ 00037 #include "threads_platform.h" 00038 00039 #include <aws_iot_error.h> 00040 00041 /** 00042 * @brief Mutex Type 00043 * 00044 * Forward declaration of a mutex struct. The definition of this struct is 00045 * platform dependent. When porting to a new platform add this definition 00046 * in "threads_platform.h". 00047 * 00048 */ 00049 typedef struct _IoT_Mutex_t IoT_Mutex_t; 00050 00051 /** 00052 * @brief Initialize the provided mutex 00053 * 00054 * Call this function to initialize the mutex 00055 * 00056 * @param IoT_Mutex_t - pointer to the mutex to be initialized 00057 * @return IoT_Error_t - error code indicating result of operation 00058 */ 00059 IoT_Error_t aws_iot_thread_mutex_init(IoT_Mutex_t *); 00060 00061 /** 00062 * @brief Lock the provided mutex 00063 * 00064 * Call this function to lock the mutex before performing a state change 00065 * This is a blocking call. 00066 * 00067 * @param IoT_Mutex_t - pointer to the mutex to be locked 00068 * @return IoT_Error_t - error code indicating result of operation 00069 */ 00070 IoT_Error_t aws_iot_thread_mutex_lock(IoT_Mutex_t *); 00071 00072 /** 00073 * @brief Lock the provided mutex 00074 * 00075 * Call this function to lock the mutex before performing a state change. 00076 * This is not a blocking call. 00077 * 00078 * @param IoT_Mutex_t - pointer to the mutex to be locked 00079 * @return IoT_Error_t - error code indicating result of operation 00080 */ 00081 IoT_Error_t aws_iot_thread_mutex_trylock(IoT_Mutex_t *); 00082 00083 /** 00084 * @brief Unlock the provided mutex 00085 * 00086 * Call this function to unlock the mutex before performing a state change 00087 * 00088 * @param IoT_Mutex_t - pointer to the mutex to be unlocked 00089 * @return IoT_Error_t - error code indicating result of operation 00090 */ 00091 IoT_Error_t aws_iot_thread_mutex_unlock(IoT_Mutex_t *); 00092 00093 /** 00094 * @brief Destroy the provided mutex 00095 * 00096 * Call this function to destroy the mutex 00097 * 00098 * @param IoT_Mutex_t - pointer to the mutex to be destroyed 00099 * @return IoT_Error_t - error code indicating result of operation 00100 */ 00101 IoT_Error_t aws_iot_thread_mutex_destroy(IoT_Mutex_t *); 00102 00103 #ifdef __cplusplus 00104 } 00105 #endif 00106 00107 #endif /*__THREADS_INTERFACE_H_*/ 00108 #endif /*_ENABLE_THREAD_SUPPORT_*/
Generated on Tue Jul 12 2022 20:38:50 by
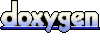