
Project for MPOA 2015/2016. Communication link with nRF24L01+.
Fork of nRF24L01P_Hello_World by
menu.cpp
00001 #include "mbed.h" 00002 #include "nRF24L01P.h" 00003 #include "menu.h" 00004 00005 extern bool tx_mode; //Global variable for TX/RX mode setting 00006 extern bool keyboard_mode; //Global variable for keyboard mode setting 00007 extern uint8_t interval; //Global variable for time interval setting 00008 00009 Serial pc2(USBTX, USBRX); // tx, rx 00010 nRF24L01P nrf24l01p(PTD2, PTD3, PTD1, PTD0, PTD5, PTA13); // mosi, miso, sck, csn, ce, irq 00011 00012 00013 void settings() { 00014 uint16_t frequency; 00015 char input[]= ""; 00016 char *ptr; 00017 00018 pc2.printf("***Experimental 2.4 GHz Radio Link. Setup below: ***\n\n"); 00019 00020 pc2.printf("Select TX or RX Mode. TX calculates BER (only in automatic mode) and ACKs,\n RX shows number of incoming packets.:\n"); 00021 pc2.printf("1: TX\n2: RX\n"); 00022 //Select TX or RX mode 00023 switch (pc2.getc()) { 00024 case '1': 00025 tx_mode = true; 00026 break; 00027 case '2': 00028 tx_mode = false; 00029 break; 00030 default: 00031 tx_mode = true; 00032 pc2.printf("Wrong number! Default TX Mode was set"); 00033 wait_ms(1000); 00034 break; 00035 } 00036 //Clear the console window 00037 pc2.puts("\e[2J\e[H"); 00038 00039 pc2.printf("Select Input. If automatic is set, the message is transmitted repeatedly\n in short intervals.:\n"); 00040 pc2.printf("1: Keyboard\n2: Automatic\n"); 00041 //Select keyboard input or automatic mode, if automatic mode is set and the device is in TX mode, select time interval 00042 switch (pc2.getc()) { 00043 case '1': 00044 keyboard_mode = true; 00045 break; 00046 case '2': 00047 keyboard_mode = false; 00048 if (tx_mode) { 00049 pc2.puts("\e[2J\e[H"); 00050 pc2.printf("Please specify a time interval. The interval must be an integer number:\n"); 00051 interval = pc2.getc() - '0'; 00052 } 00053 break; 00054 default: 00055 keyboard_mode = true; 00056 pc2.printf("Wrong number! Default Automatic mode was set"); 00057 wait_ms(1000); 00058 break; 00059 } 00060 //Clear the console window 00061 pc2.puts("\e[2J\e[H"); 00062 00063 pc2.printf("Type in the RF channel depending on the speed in the range of 2400 - 2525.\n The channel must be the same for RX and TX!: \n"); 00064 //Type the number of desired RF channel in range 00065 pc2.gets(input, 5); 00066 frequency = (int) strtol(input, &ptr, 10); 00067 pc2.printf("Selected frequency: %d\n", frequency); 00068 if (frequency >= 2400 && frequency <=2525) { 00069 nrf24l01p.setRfFrequency(frequency); 00070 } 00071 //If wrong number was set, default frequency will be set 00072 else { 00073 pc2.printf("Wrong frequency! Default frequency was set (2402 MHz)\n"); 00074 } 00075 //Clear the console window 00076 pc2.puts("\e[2J\e[H"); 00077 00078 pc2.printf("Select radio output power:\n"); 00079 pc2.printf("1: 0 dB\n2: -6 dB\n3: -12 dB\n4: -18 dB\n"); 00080 //Select the RF output power 00081 switch (pc2.getc()) { 00082 case '1': 00083 nrf24l01p.setRfOutputPower(NRF24L01P_TX_PWR_ZERO_DB); 00084 break; 00085 case '2': 00086 nrf24l01p.setRfOutputPower(NRF24L01P_TX_PWR_MINUS_6_DB); 00087 break; 00088 case '3': 00089 nrf24l01p.setRfOutputPower(NRF24L01P_TX_PWR_MINUS_12_DB); 00090 break; 00091 case '4': 00092 nrf24l01p.setRfOutputPower(NRF24L01P_TX_PWR_MINUS_18_DB); 00093 break; 00094 default: 00095 pc2.printf("Wrong number! Default value was set (0 dB)\n"); 00096 wait_ms(1000); 00097 break; 00098 } 00099 //Clear the console window 00100 pc2.puts("\e[2J\e[H"); 00101 00102 pc2.printf("Set air data rate. The air data rate must be the same for RX and TX!:\n"); 00103 pc2.printf("1: 250 KBps\n2: 1 MBps\n3: 2 MBps\n"); 00104 //Set the air data rate 00105 switch (pc2.getc()) { 00106 case '1': 00107 nrf24l01p.setAirDataRate(NRF24L01P_DATARATE_250_KBPS); 00108 break; 00109 case '2': 00110 nrf24l01p.setAirDataRate(NRF24L01P_DATARATE_1_MBPS); 00111 break; 00112 case '3': 00113 nrf24l01p.setAirDataRate(NRF24L01P_DATARATE_2_MBPS); 00114 break; 00115 default: 00116 pc2.printf("Wrong number! Default value was set (1 MBps)\n"); 00117 wait_ms(1000); 00118 break; 00119 } 00120 //Clear the console window 00121 pc2.puts("\e[2J\e[H"); 00122 } 00123 00124 00125
Generated on Thu Jul 14 2022 17:56:10 by
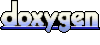