lib
Embed:
(wiki syntax)
Show/hide line numbers
H3LIS331.h
00001 #ifndef H3LIS331_H 00002 #define H3LIS331_H 00003 00004 /** 00005 * Includes 00006 */ 00007 #include "mbed.h" 00008 00009 /** 00010 * Defines 00011 */ 00012 #define H3LIS331_I2C_ADDRESS 0x18 //7-bit address. 00013 00014 //----------- 00015 // Registers 00016 //----------- 00017 #define WHO_AM_I_REG_H3LIS331 0x0F 00018 #define ACCEL_XOUT_H_REG 0x29 00019 #define ACCEL_XOUT_L_REG 0x28 00020 #define ACCEL_YOUT_H_REG 0x2B 00021 #define ACCEL_YOUT_L_REG 0x2A 00022 #define ACCEL_ZOUT_H_REG 0x2D 00023 #define ACCEL_ZOUT_L_REG 0x2C 00024 00025 00026 00027 #define CTRL_REG_1 0x20 00028 #define CTRL_REG_2 0x21 00029 #define CTRL_REG_3 0x22 00030 #define CTRL_REG_4 0x23 00031 #define CTRL_REG_5 0x24 00032 00033 #define STATUS_REG 0x27 00034 00035 #define INT1_CFG 0x30 00036 #define INT1_SRC 0x31 00037 #define INT1_THS 0x32 00038 #define INT1_DURATION 0x33 00039 #define INT2_CFG 0x34 00040 #define INT2_SRC 0x35 00041 #define INT2_THS 0x36 00042 #define INT2_DURATION 0x37 00043 00044 //------------------------------ 00045 // Power Mode and Output Data Rates 00046 //------------------------------ 00047 #define POWER_DOWN 0x00 00048 #define NORMAL_50HZ 0x27 00049 #define NORMAL_100HZ 0x2F 00050 #define NORMAL_400HZ 0x37 00051 #define NORMAL_1000HZ 0x3F 00052 #define LOW_POWER_0_5HZ 0x47 00053 #define LOW_POWER_1HZ 0x67 00054 #define LOW_POWER_2HZ 0x87 00055 #define LOW_POWER_5HZ 0xA7 00056 #define LOW_POWER_10HZ 0xC7 00057 00058 /** 00059 * H3LIS331 triple axis digital accelerometer. 00060 */ 00061 class H3LIS331 { 00062 00063 public: 00064 00065 /** 00066 * Constructor. 00067 * 00068 * Sets FS_SEL to 0x03 for proper opertaion. 00069 * 00070 * @param sda - mbed pin to use for the SDA I2C line. 00071 * @param scl - mbed pin to use for the SCL I2C line. 00072 */ 00073 H3LIS331(PinName sda, PinName scl); 00074 00075 /** 00076 * Get the identity of the device. 00077 * 00078 * @return The contents of the Who Am I register which contains the I2C 00079 * address of the device. 00080 */ 00081 char getWhoAmI(void); 00082 00083 00084 00085 00086 00087 00088 00089 00090 00091 /** 00092 * Set the power mode (power down, low power, normal mode) 00093 * 00094 * 00095 * @param 00096 * 00097 * Power Mode | Output Data Rate (Hz) | Low-pass Filter Cut off (Hz) | #define 00098 * -------------------------------------------------------------------------------- 00099 * Power-down | -- | -- | POWER_DOWN 00100 * Normal | 50 | 37 | NORMAL_50HZ 00101 * Normal | 100 | 74 | NORMAL_100HZ 00102 * Normal | 400 | 292 | NORMAL_400HZ 00103 * Normal | 1000 | 780 | NORMAL_1000HZ 00104 * Low-power | 0.5 | -- | LOW_POWER_0_5HZ 00105 * Low-power | 1 | -- | LOW_POWER_1HZ 00106 * Low-power | 2 | -- | LOW_POWER_2HZ 00107 * Low-power | 5 | -- | LOW_POWER_5HZ 00108 * Low-power | 10 | -- | LOW_POWER_10HZ 00109 */ 00110 00111 void setPowerMode(char power_mode); 00112 00113 00114 00115 /** 00116 * Get the current power mode 00117 * 00118 * @return 00119 */ 00120 char getPowerMode(void); 00121 00122 00123 char getInterruptConfiguration(void); 00124 00125 /** 00126 * Set the interrupt configuration. 00127 * 00128 * See datasheet for configuration byte details. 00129 * 00130 * 7 6 5 4 00131 * +-------+-------+------+--------+ 00132 * | IHL | PP_OD | LIR2 | I2_CFG | 00133 * +-------+-------+------+--------+ 00134 * 00135 * 3 2 1 0 00136 * +---------+------+---------+---------+ 00137 * | I2_CFG0 | LIR1 | I1_CFG1 | I1-CFG0 | 00138 * +---------+------+---------+---------+ 00139 * 00140 * IHL Interrupt active high or low. 0:active high; 1:active low (default:0) 00141 * PP_OD Push-pull/Open drain selection on interrupt pad. 0:push-pull; 1:open drain (default:0) 00142 * LIR2 Latch interupt request on INT2_SRC register, with INT2_SRC register cleared by reading INT2_SRC itself 00143 * 0: irq not latched; 1:irq latched (default:0) 00144 * I2_CFG1, I2_CFG0 See datasheet table 00145 * LIR1 Latch interupt request on INT1_SRC register, with INT1_SRC register cleared by reading INT1_SRC itself 00146 * 0: irq not latched; 1:irq latched (default:0) 00147 * I1_CFG1, I1_CFG0 See datasheet table 00148 * 00149 * @param config Configuration byte to write to INT_CFG register. 00150 */ 00151 00152 00153 // void setInterruptConfiguration(char config); 00154 00155 /** 00156 * Check the status register 00157 * 00158 * @return 00159 * 00160 */ 00161 00162 00163 /** 00164 * Set the Full Scale Range to +/- 400g's. 00165 * 00166 */ 00167 void setFullScaleRange400g(void); 00168 00169 /** 00170 * Set the Full Scale Range to +/- 200g's. 00171 * 00172 */ 00173 void setFullScaleRange200g(void); 00174 00175 /** 00176 * Set the Full Scale Range to +/- 100g's. 00177 * 00178 */ 00179 void setFullScaleRange100g(void); 00180 00181 00182 char getAccelStatus(void); 00183 00184 00185 00186 00187 /** 00188 * Get the output for the x-axis accelerometer. 00189 * 00190 * @return The output on the x-axis in engineering units (g's). 00191 */ 00192 float getAccelX(void); 00193 00194 /** 00195 * Get the output for the y-axis accelerometer. 00196 * 00197 * @return The output on the y-axis in engineering units (g's). 00198 */ 00199 float getAccelY(void); 00200 00201 /** 00202 * Get the output on the z-axis accelerometer. 00203 * 00204 * @return The output on the z-axis in engineering units (g's). 00205 */ 00206 float getAccelZ(void); 00207 00208 00209 private: 00210 00211 float scaling_factor; 00212 int current_range; 00213 00214 I2C i2c_; 00215 00216 }; 00217 00218 #endif /* H3LIS331_H */ 00219
Generated on Fri Jul 15 2022 09:28:08 by
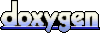