
LaOS Laser test program
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 * main.cpp 00003 * 00004 * Copyright (c) 2011 Peter Brier 00005 * 00006 * This file is part of the LaOS project (see: http://wiki.protospace.nl/index.php/LaOS) 00007 * 00008 * LaOS is free software: you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation, either version 3 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * LaOS is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License 00019 * along with LaOS. If not, see <http://www.gnu.org/licenses/>. 00020 * 00021 * This program implements Bresenham line algorithm program (2D) 00022 * see: http://en.wikipedia.org/wiki/Bresenham%27s_line_algorithm 00023 * In addition: we controll a Z-axis and a laser. 00024 * Note: Fixed speed lasering (no accelleration). 00025 * Simple program, no line buffering. Considerable jitter on pulses. Speed varies approx 30%, depending on angle (varies over 1.5 degree) 00026 * 00027 */ 00028 #include "mbed.h" 00029 00030 // serial comm 00031 Serial pc(USBTX, USBRX); 00032 00033 // status leds 00034 DigitalOut led1(LED1); 00035 DigitalOut led2(LED2); 00036 DigitalOut led3(LED3); 00037 DigitalOut led4(LED4); 00038 00039 // motors 00040 DigitalOut xdir(p26); 00041 DigitalOut xstep(p25); 00042 DigitalOut ydir(p24); 00043 DigitalOut ystep(p23); 00044 DigitalOut zdir(p22); 00045 DigitalOut zstep(p21); 00046 00047 // laser 00048 DigitalOut laser(p27); 00049 00050 // globals 00051 Ticker ticker; // Timer 00052 00053 // Besenham variables 00054 int state, laseron; 00055 int x0,y0,x1,y1,z0,z1; // positions: 0=current, 1=new 00056 int dx, sx; // step 00057 int dy, sy; // delta 00058 int err, e2; // error 00059 00060 00061 /** 00062 *** ticker: implement timer ticker, step if required 00063 **/ 00064 void tick() 00065 { 00066 // Control X,Y: line tracker 00067 switch ( state ) 00068 { 00069 case 0: // idle (make sure laser is off) 00070 laser = laseron = 0; 00071 break; 00072 case 1: // init 00073 dx = abs(x1-x0); 00074 sx = x0<x1 ? 1 : -1; 00075 dy = abs(y1-y0); 00076 sy = y0<y1 ? 1 : -1; 00077 err = (dx>dy ? dx : -dy)/2; 00078 xdir = sx > 0; 00079 ydir = sy > 0; 00080 state++; 00081 laser = laseron; 00082 break; 00083 case 2: // moving 00084 if (x0==x1 && y0==y1) 00085 state = 0; 00086 else 00087 { 00088 e2 = err; 00089 if (e2 >-dx) { err -= dy; x0 += sx; xstep = 1;} 00090 if (e2 < dy) { err += dx; y0 += sy; ystep = 1;} 00091 } 00092 break; 00093 } 00094 00095 // Controll Z: tracking controller (independent of XY motion, not queued) 00096 if ( z1 != z0 ) 00097 { 00098 zdir = z1 > z0; 00099 wait_us(5); 00100 zstep= 1; 00101 if ( z1 > z0 ) z0++; 00102 else z0--; 00103 } 00104 00105 if ( xstep == 1 || ystep == 1 || zstep == 1 ) 00106 { 00107 wait_us(5); 00108 xstep = ystep = zstep = 0; 00109 } 00110 00111 // diagnostic info 00112 led1 = xdir; 00113 led2 = ydir; 00114 led3 = zdir; 00115 led4 = laser; 00116 } // ticker 00117 00118 00119 00120 /** 00121 *** main() 00122 *** Read commands, report state 00123 **/ 00124 int main() 00125 { 00126 int v=100,c=0,z=0; 00127 00128 pc.baud(115200); 00129 ticker.attach_us(&tick, v); // the address of the function to be attached (flip) and the interval (microseconds) 00130 00131 // init 00132 state = laser = xstep = xdir = ystep = ydir = zstep = zdir = 0; 00133 00134 while( 1 ) 00135 { 00136 scanf("%d", &c); 00137 switch( c ) 00138 { 00139 case 0: // move x,y (laser off) 00140 scanf("%d %d", &x1, &y1); 00141 laseron = 0; 00142 state = 1; 00143 break; 00144 case 1: // line x,y (laser on) 00145 scanf("%d %d", &x1, &y1); 00146 laseron = 1; 00147 state = 1; 00148 break; 00149 case 2: // move z 00150 scanf("%d", &z1); 00151 break; 00152 case 3: // set t (1/v) 00153 scanf("%d", &v); 00154 ticker.attach_us(&tick, v); 00155 break; 00156 case 4: // set x,y,z absolute 00157 scanf("%d %d %d", &x0, &y0, &z); 00158 z1 = z0 = z; 00159 case 5: // nop 00160 break; 00161 default: // I do not understand: stop motion 00162 state = 0; 00163 break; 00164 } 00165 printf("%d %d %d %d %d %d\r\n", state, x0, y0, z0, v, laseron); 00166 } 00167 }
Generated on Sun Jul 17 2022 05:10:53 by
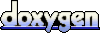